Selenium Waits: A Comprehensive Guide with Code Examples
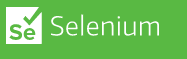
One of the biggest challenges when writing automated tests with Selenium is dealing with synchronization issues. Tests can fail when the application under test is not yet ready for the next step. To address this, Selenium provides different types of waits that allow tests to wait for certain conditions to be met before moving on to the next step.
In this article, we will explore the different types of waits available in Selenium and provide examples of how to use them in your automated tests.
Implicit Waits
Implicit waits are set globally for the entire test execution and apply to all elements that Selenium interacts with. An implicit wait tells Selenium to wait for a specified amount of time before throwing a NoSuchElementException if an element is not found.
Here's an example of how to use an implicit wait in Selenium with Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class ImplicitWaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.example.com/");
WebElement element = driver.findElement(By.id("example-element"));
System.out.println(element.getText());
driver.quit();
}
}
In this example, we have set an implicit wait of 10 seconds using the implicitlyWait() method. This means that Selenium will wait up to 10 seconds for an element to be found before throwing a NoSuchElementException. We then find an element using the findElement() method and output its text to the console.
Explicit Waits
Explicit waits allow for more granular control over when Selenium waits for a certain condition to be met. An explicit wait can be set for a specific condition, such as waiting for an element to be visible or clickable.
Here's an example of how to use an explicit wait in Selenium with Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com/");
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("example-element")));
System.out.println(element.getText());
driver.quit();
}
}
In this example, we have created an explicit wait using the WebDriverWait class and the ExpectedConditions.visibilityOfElementLocated() method. This waits for up to 10 seconds for the element with the ID "example-element" to become visible before moving on to the next step.
We then find the element using the until() method and output its text to the console.
In conclusion, using waits is essential for improving the reliability and stability of your Selenium automated tests. With the examples and best practices outlined in this article, you should be well-equipped to use waits in your Selenium tests.
Use the examples above as a guide to get started with waits in Selenium and take your automated testing to the next level.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer