Selenium TestNG: A Comprehensive Guide to Automated Testing
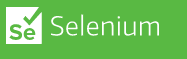
Selenium is a popular tool for automating web testing, but writing and managing automated tests can be a challenging task, especially as your test suite grows in size and complexity. This is where TestNG comes in - a powerful testing framework that can help you write more robust and scalable tests for your web applications.
TestNG is a testing framework designed to make testing more powerful and easier to maintain. It offers a wide range of features such as annotations, assertions, test dependency management, and parallel test execution. This makes it an excellent choice for creating complex and comprehensive test suites for web applications.
One of the key features of TestNG is the use of annotations to mark up your test code. Annotations are special markers that tell TestNG how to run your tests. For example, the @Test annotation is used to mark a method as a test case. The @BeforeTest and @AfterTest annotations are used to set up and tear down test fixtures.
Here's an example of how to use TestNG with Selenium in Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class TestNGExample {
private WebDriver driver;
@BeforeTest
public void setUp() {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
driver = new ChromeDriver();
driver.get("https://www.google.com/");
}
@Test
public void testGoogleSearch() {
driver.findElement(By.name("q")).sendKeys("Selenium TestNG");
driver.findElement(By.name("btnK")).click();
Assert.assertTrue(driver.getTitle().contains("Selenium TestNG"));
}
@AfterTest
public void tearDown() {
driver.quit();
}
}
In this example, we have created a new TestNG test class that opens the Google search page, enters a search query, and asserts that the search results page contains the expected title.
TestNG also offers several other useful features, such as grouping, parameterization, and data providers, which can help you write more efficient and flexible tests.
In conclusion, TestNG is a powerful testing framework that can help you write more robust and scalable automated tests for your web applications.
With its rich set of features and easy-to-use annotations, TestNG makes it easy to create comprehensive test suites that cover all aspects of your application. Use the example above as a guide to get started with TestNG and take your Selenium testing to the next level.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer