Selenium Page Object Model (POM): Best Practices and Example
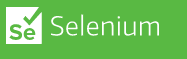
Selenium is a powerful tool for automating web testing, but writing and maintaining automated tests can be challenging, especially as your test suite grows in size and complexity. One way to address this challenge is to use the Page Object Model (POM), a design pattern that can help make your tests more maintainable, reliable, and easier to understand.
The Page Object Model is a pattern that separates the code that interacts with web elements from the actual test logic. This means that instead of interacting with web elements directly in your test code, you create separate classes for each page of your web application. These classes, known as Page Objects, encapsulate the web elements and their interactions, making your tests more readable and easier to maintain.
Here's a practical example of how to implement the Page Object Model in Selenium using Java:
First, create a new class for the home page of your web application, and define the web elements and methods that you need to interact with:
public class HomePage {
private WebDriver driver;
private By searchBox = By.name("q");
private By searchButton = By.name("btnK");
public HomePage(WebDriver driver) {
this.driver = driver;
}
public void searchFor(String text) {
driver.findElement(searchBox).sendKeys(text);
driver.findElement(searchButton).click();
}
}
In this example, we have defined a Page Object class for the home page of our web application. We have encapsulated the search box and search button web elements and their interactions in this class. The searchFor method accepts a search query and performs a search on the Google search page.
Next, in our test code, we can use the HomePage class to interact with the search box and perform a search:
public class SearchTest {
private WebDriver driver;
@Before
public void setUp() {
driver = new ChromeDriver();
driver.get("https://www.google.com/");
}
@Test
public void testSearch() {
HomePage homePage = new HomePage(driver);
homePage.searchFor("Selenium Page Object Model");
// Assert search results here
}
@After
public void tearDown() {
driver.quit();
}
}
In this example, we have created a new test class that uses the HomePage class to perform a search on the Google search page. By encapsulating the web elements and their interactions in the HomePage class, we have made our test code more readable and easier to maintain.
In conclusion, the Page Object Model is a powerful design pattern that can help make your Selenium tests more maintainable, reliable, and easier to understand. By encapsulating web elements and their interactions in separate Page Object classes, you can make your test code more readable and easier to maintain.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer