Selenium Handling Alerts: A Complete Guide with Examples
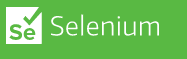
Alerts are a common feature in web applications and can be challenging to handle when writing automated tests with Selenium. In this article, we will cover everything you need to know about handling alerts in Selenium.
To get started, let's first understand what alerts are. Alerts are pop-up windows that appear in web applications to notify users of important information or to ask for confirmation before performing a critical action. Selenium can interact with alerts in three ways - accepting, dismissing, or getting the text of the alert.
Here's an example of how to handle an alert with Selenium in Java:
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AlertExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.techoral.com/");
driver.findElement(By.id("alert-button")).click();
Alert alert = driver.switchTo().alert();
String alertText = alert.getText();
System.out.println(alertText);
alert.accept();
driver.quit();
}
}
In this example, we have created a new Selenium test that clicks a button on a web page to trigger an alert. We then switch to the alert using the switchTo() method and get the text of the alert using the getText() method. Finally, we accept the alert using the accept() method.
Handling alerts can be more complicated when dealing with confirm and prompt alerts that require user input. In these cases, we need to use the sendKeys() method to enter text into the alert before accepting or dismissing it.
Here's an example of how to handle a confirm alert in Selenium with Java:
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ConfirmAlertExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.techoral.com/");
driver.findElement(By.id("confirm-button")).click();
Alert alert = driver.switchTo().alert();
String alertText = alert.getText();
System.out.println(alertText);
alert.sendKeys("Yes");
alert.accept();
driver.quit();
}
}
In this example, we have created a new Selenium test that clicks a button on a web page to trigger a confirm alert. We then switch to the alert using the switchTo() method, enter text using the sendKeys() method, and accept the alert using the accept() method.
In conclusion, handling alerts in Selenium is an essential skill for writing robust and reliable automated tests. With the examples and best practices outlined in this article, you should be well-equipped to handle alerts in your Selenium tests.
Use the examples above as a guide to get started with alert handling in Selenium and take your automated testing to the next level.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer