How to Handle Tokens and Set Them in Local Storage in Cypress Tests: A Step-by-Step Guide
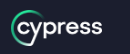
Cypress is a popular end-to-end testing framework that is widely used in the web development industry. It offers a lot of features that make testing web applications much easier and efficient.
One such feature is the cypress-localstorage-commands plugin. In this article, we will explore what cypress-localstorage-commands is, how to use it, and why it is useful.
Also, we will discuss how to handle tokens in Cypress tests and set them in local storage, which can help improve the accuracy of your tests.
What is cypress-localstorage-commands?
Cypress-localstorage-commands is a plugin for Cypress that allows you to easily interact with the local storage of your web application during tests. Local storage is a mechanism that allows web applications to store data on a user's computer. It is often used to store user preferences, settings, and other data that needs to persist across sessions.
Cypress-localstorage-commands provides a set of custom commands that allow you to read, write, and clear data from the local storage of your application. These commands make it easy to set up your tests by pre-populating the local storage with data, or to check that your application is correctly interacting with the local storage.
Why is cypress-localstorage-commands useful?
Cypress-localstorage-commands is useful for several reasons. First, it allows you to easily interact with the local storage of your application during tests. This can be useful for testing features that rely on local storage data, such as user preferences or settings.
Second, cypress-localstorage-commands allows you to set up your tests by pre-populating the local storage with data. This can save you time and effort when writing tests, as you don't have to manually set up the local storage each time.
Finally, cypress-localstorage-commands allows you to check that your application is correctly interacting with the local storage. This can help you catch bugs early in the development process, and ensure that your application is working as expected.
Step 1: Understand the Concept of Tokens
Before we dive into the technical aspects of handling tokens in Cypress, it's important to understand what tokens are and why they are used.
In the context of web applications, tokens are essentially pieces of information that are used for authentication and authorization purposes.
They are used to validate a user's identity and determine what actions they are authorized to perform within the application.
Step 2: Install Cypress Local Storage Plugin
To set tokens in local storage in Cypress tests, we need to use the Cypress Local Storage Plugin. This plugin allows us to add items to the browser's local storage, which is a key-value store that can be used to persist data between test runs.
To install the plugin, simply run the following command in your project directory:
npm install --save-dev cypress-localstorage-commands
Step 3: Write Your Test
Now that we have installed the Cypress Local Storage Plugin, we can write our test. Let's say we have a web application that requires a user to log in before accessing certain features. We can write a test to ensure that the user is redirected to the login page if they are not authenticated.
describe('Authentication', () => {
it('redirects unauthenticated users to login page', () => {
cy.visit('/dashboard')
cy.get('h1').should('contain', 'Login')
})
})
This test will visit the dashboard page and assert that the heading contains the word "Login". Since the user is not authenticated, they should be redirected to the login page.
Step 4: Set the Token in Local Storage
To set the token in local storage, we need to use the cy.setLocalStorage() command provided by the Cypress Local Storage Plugin. This command takes two arguments: the key and the value.
Let's assume that our web application returns a JSON Web Token (JWT) upon successful authentication. We can extract this token from the response and set it in local storage as follows:
describe('Authentication', () => {
it('redirects unauthenticated users to login page', () => {
cy.request('POST', '/login', {
username: 'testuser',
password: 'password'
}).then((response) => {
const token = response.body.token
cy.setLocalStorage('token', token)
})
cy.visit('/dashboard')
cy.get('h1').should('contain', 'Dashboard')
})
})
In this example, we make a POST request to the login endpoint with a test username and password. We then extract the token from the response and set it in local storage with the key "token".
Finally, we visit the dashboard page and assert that the heading contains the word "Dashboard", indicating that the user has been successfully authenticated.
Step 5: Test Your Application
With the token set in local storage, we can now test our application with greater accuracy. The Cypress Local Storage Plugin will persist the token between test runs, allowing us to simulate real-world scenarios where the user is authenticated and authorized to perform certain actions.
Conclusion
In this article, we discussed how to handle tokens in Cypress tests and set them in local storage, which can help improve the accuracy of your tests.
By using the Cypress Local Storage Plugin, we can easily set the token in local storage and simulate real-world scenarios where the user is authenticated and authorized to perform certain actions.
Handling tokens is an important aspect of testing web applications, and with the right tools and techniques, it can be made much easier.
By following the steps outlined in this article, you can ensure that your Cypress tests accurately reflect the behavior of your application, which can lead to a more reliable and robust testing suite.
Read Next :
- Cypress Installation Errors
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer