Selenium Cross-Browser Testing: A Comprehensive Guide with Code Examples
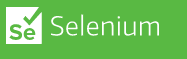
With so many different web browsers and versions available, it's important to ensure that your web application functions as expected across all of them.
Cross-browser testing is the process of testing your web application across multiple browsers to ensure compatibility.
Selenium is a powerful tool for automated testing, and it can be used to perform cross-browser testing as well. In this article, we will explore how to perform cross-browser testing with Selenium and provide examples of how to do so.
Setting Up Cross-Browser Testing in Selenium
To perform cross-browser testing in Selenium, you will need to set up your testing environment to include the different browsers you want to test on. This involves downloading and installing the browser-specific drivers for each browser you want to test on.
Here's an example of how to set up cross-browser testing in Selenium with Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class CrossBrowserTestingExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
System.setProperty("webdriver.ie.driver", "/path/to/IEDriverServer");
WebDriver chromeDriver = new ChromeDriver();
WebDriver firefoxDriver = new FirefoxDriver();
WebDriver ieDriver = new InternetExplorerDriver();
chromeDriver.get("https://www.example.com/");
firefoxDriver.get("https://www.example.com/");
ieDriver.get("https://www.example.com/");
chromeDriver.quit();
firefoxDriver.quit();
ieDriver.quit();
}
}
In this example, we have set up cross-browser testing using Chrome, Firefox, and Internet Explorer. We have set the system properties for each driver and instantiated a driver object for each browser. We then navigate to the same URL in each browser and close the drivers.
Performing Cross-Browser Testing in Selenium
Once you have set up your testing environment, you can start writing tests that will run on multiple browsers. You can use the same code for each browser, or you can write browser-specific code for each test.
Here's an example of how to perform cross-browser testing in Selenium with Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class CrossBrowserTestingExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
System.setProperty("webdriver.ie.driver", "/path/to/IEDriverServer");
WebDriver chromeDriver = new ChromeDriver();
WebDriver firefoxDriver = new FirefoxDriver();
WebDriver ieDriver = new InternetExplorerDriver();
chromeDriver.get("https://www.example.com/");
firefoxDriver.get("https://www.example.com/");
ieDriver.get("https://www.example.com/");
WebElement element1 = chromeDriver.findElement(By.id("example-element"));
WebElement element2 = firefoxDriver.findElement(By.id("example-element"));
WebElement element3 = ieDriver.findElement(By.id("example-element"));
System.out.println(element1.getText());
System.out.println(element2.getText());
System.out.println(element3.getText());
chromeDriver.quit();
firefoxDriver.quit();
ieDriver.quit();
}
}
In this example, we have set up cross-browser testing using Chrome, Firefox, and Internet Explorer. We have navigated to the same URL in each browser and retrieved the text of an element with the ID "example-element" in each browser. We have then printed the text to the console.
Best Practices for Cross-Browser Testing in Selenium
Here are some best practices to follow when performing cross-browser testing in Selenium:
- Test on the most popular browsers: Focus on testing your web application on the most popular browsers used by your target audience. This will ensure that you cover the majority of your users.
- Use a testing framework: A testing framework like TestNG can help you write and execute tests across multiple browsers more efficiently.
- Use cloud-based testing platforms: Cloud-based testing platforms like Sauce Labs and BrowserStack can provide access to a wide range of browsers and versions, making cross-browser testing much easier.
- Test on different operating systems: Ensure that your web application functions as expected on different operating systems as well as different browsers.
Conclusion
Cross-browser testing is an important part of ensuring the quality and compatibility of your web application.
With Selenium, you can easily set up and perform cross-browser testing across multiple browsers and versions. By following best practices and using the right tools, you can ensure that your web application functions as expected for all of your users.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer