A Guide to Handling Multiple Tabs in Cypress with Examples
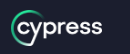
Cypress is a popular JavaScript end-to-end testing framework that allows developers to write automated tests for web applications. When testing web applications, it's common to encounter scenarios where a new tab or window is opened, and it becomes necessary to interact with it.
In this article, we'll explore how to handle multiple tabs in Cypress using various methods and provide examples to illustrate their usage.
Method 1: Using the window:contains event
The window:contains event is triggered when a new tab or window is opened, and it contains a specific string in its title. To handle a new tab using this method, we need to listen to this event and switch to the new tab when it's triggered. Here's an example:
cy.on('window:contains', (str) => {
if (str === 'New Tab Title') {
cy.window().then((win) => {
cy.wrap(win).as('newTab');
});
}
});
cy.get('a[target="_blank"]').click();
cy.get('@newTab').its('document').should('exist');
In this example, we're listening to the window:contains event and checking if the new tab's title contains the string "New Tab Title". If it does, we're wrapping the new tab's window object in a Cypress alias called @newTab. We can then use this alias to interact with the new tab as we would with the current tab.
Method 2: Using the window.open method
Another way to handle a new tab is to use the window.open method to open a new tab and then switch to it. Here's an example:
cy.window().then((win) => {
win.open('https://www.example.com', '_blank');
});
cy.window().then((win) => {
cy.stub(win, 'open').as('windowOpen');
});
cy.get('a[target="_blank"]').click();
cy.get('@windowOpen').should('be.called');
cy.get('@windowOpen').should('be.calledWith', 'https://www.example.com', '_blank');
In this example, we're using the window.open method to open a new tab with the URL "https://www.example.com". We're also using a Cypress alias called @windowOpen to stub the window.open method, so we can assert that it was called with the correct arguments.
Method 3: Using the cy.visit method
The cy.visit method is typically used to visit a new URL in the current tab, but it can also be used to open a new tab with a specified URL. Here's an example:
cy.visit('https://www.example.com', {
onLoad: (win) => {
cy.stub(win, 'open').as('windowOpen');
}
});
cy.get('a[target="_blank"]').click();
cy.get('@windowOpen').should('be.called');
cy.get('@windowOpen').should('be.calledWith', 'https://www.example.com', '_blank');
In this example, we're using the cy.visit method to open a new tab with the URL "https://www.example.com". We're also using a Cypress alias called @windowOpen to stub the window.open method, so we can assert that it was called with the correct arguments.
Conclusion
Handling multiple tabs in Cypress can be tricky, but there are several methods available to make it easier. By using the `window:
Read Next :
- Cypress Installation Errors
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer