Cypress Test Automation Example: A Comprehensive Guide
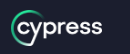
Cypress is a popular open-source test automation tool that helps you write and run tests for web applications. With its simple and easy-to-use syntax, Cypress allows developers to create end-to-end tests, integration tests, and unit tests quickly and efficiently.
In this article, we'll provide a step-by-step guide on how to perform Cypress test automation with an example project.
Getting Started with Cypress
To get started with Cypress, you first need to install it on your system. You can install it via npm or yarn, or by downloading the Cypress executable from the Cypress website. Please refer How to Install Cypressfor detailed steps to install cypress on your system.
Once you have installed Cypress, you can create a new project by running the following command:
npm init
npm install cypress --save-dev
This will create a new project folder and install Cypress as a dev dependency. You can then open the Cypress Test Runner by running the following command:
npx cypress open
This will launch the Cypress Test Runner, where you can write and run your tests.
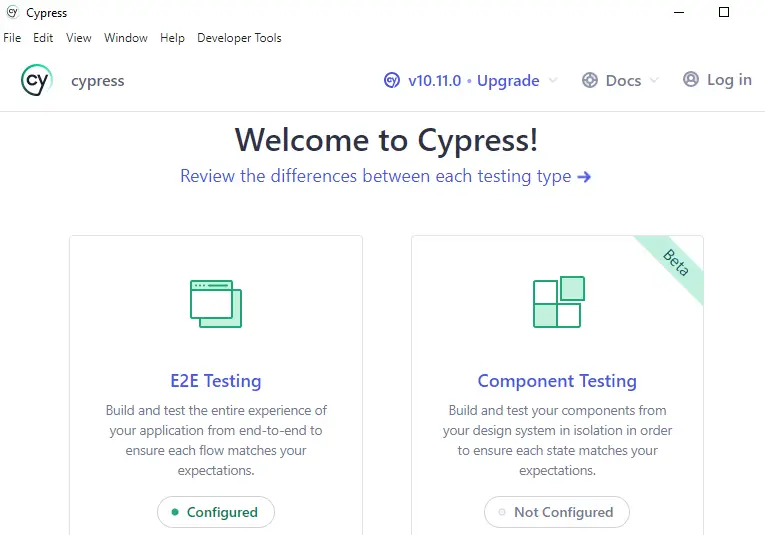
Click on E2E Testing
Start E2E Testing in Electron / Chrome / Edge
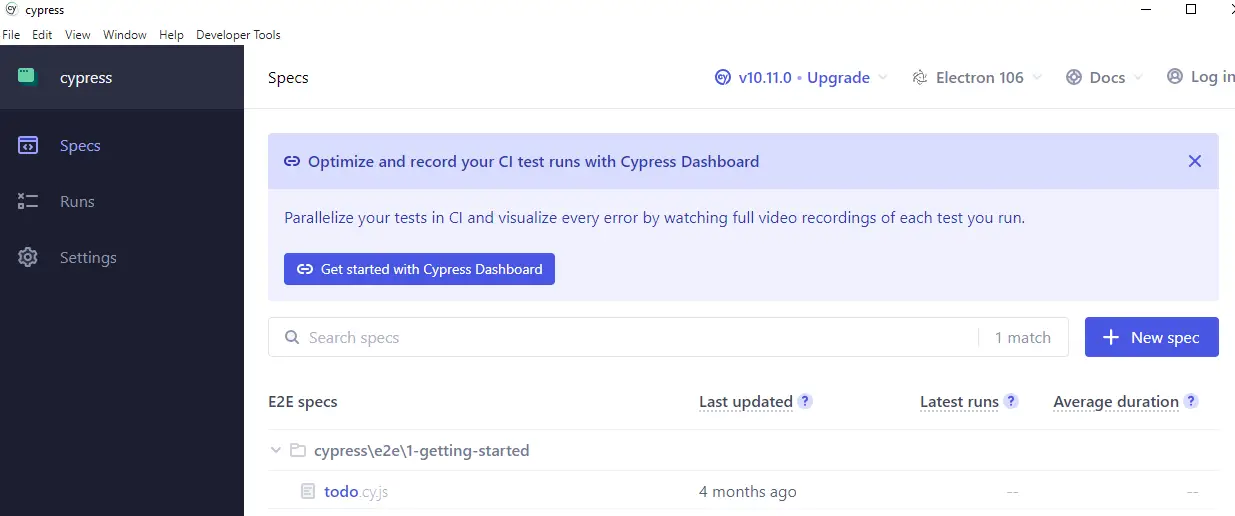
Run tests
Writing Tests with Cypress
Cypress uses a simple and intuitive syntax to write tests. Here's an example of a test that checks if a login form works correctly:
describe('Login Form', () => {
it('logs in successfully', () => {
cy.visit('https://techoral.com/login')
cy.get('input[name="email"]').type('info@techoral.com')
cy.get('input[name="password"]').type('password123')
cy.get('button[type="submit"]').click()
cy.url().should('include', '/dashboard')
})
})
In this test, we use the describe and it functions to define a test suite and a test case, respectively. We then use Cypress commands like cy.visit, cy.get, and cy.url to interact with the login form and check if the user is redirected to the dashboard page after a successful login.
More Cypress test automation examples with code snippets
Here are 10 Cypress test automation examples with code snippets:
1. Checking if an element exists on a page:
it('should have a header', () => {
cy.visit('https://techoral.com');
cy.get('header').should('exist');
});
2. Clicking on a button and verifying its behavior:
it('should submit the form', () => {
cy.visit('https://techoral.com/form');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/success');
});
3. Filling out a form and submitting it:
it('should fill out and submit the form', () => {
cy.visit('https://techoral.com/form');
cy.get('input[name="name"]').type('techoral');
cy.get('input[name="email"]').type('info@techoral.com');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/success');
});
4. Testing an API endpoint:
it('should get a list of users', () => {
cy.request('/api/users').then((response) => {
expect(response.status).to.eq(200);
expect(response.body).to.have.length(10);
});
});
5. Testing authentication:
it('should log in the user', () => {
cy.visit('https://techoral.com/login');
cy.get('input[name="username"]').type('techoral');
cy.get('input[name="password"]').type('password123');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
});
6. Checking if an element is visible:
it('should show the modal when the button is clicked', () => {
cy.visit('https://techoral.com');
cy.get('button').click();
cy.get('.modal').should('be.visible');
});
7. Checking if an element contains certain text:
it('should display the correct message', () => {
cy.visit('https://techoral.com');
cy.get('h1').should('contain', 'Welcome to techoral.com');
});
8. Navigating between pages:
it('should navigate to the about page', () => {
cy.visit('https://techoral.com');
cy.get('a[href="/about"]').click();
cy.url().should('include', '/about');
});
9. Testing a dropdown menu:
it('should select an option from the dropdown', () => {
cy.visit('https://techoral.com');
cy.get('select').select('Option 2');
cy.get('select').should('have.value', '2');
});
10. Testing a checkbox:
it('should check the checkbox', () => {
cy.visit('https://techoral.com');
cy.get('input[type="checkbox"]').check();
cy.get('input[type="checkbox"]').should('be.checked');
});
Best Practices for Cypress Test Automation
To make the most out of Cypress test automation, here are some best practices that you should follow:
1. Write clear and concise tests that cover all the important use cases of your application.
2. Use fixtures to load test data from external files.
3. Use Cypress commands like cy.wait and cy.intercept to control the flow of your tests.
4. Use custom commands and reusable functions to reduce code duplication and improve test readability.
5.Run your tests in headless mode for faster and more efficient testing.
Summary:
Cypress is a powerful and easy-to-use test automation tool that can help you improve the quality of your web applications. With its simple syntax and powerful features, Cypress makes it easy for developers to write and run tests quickly and efficiently. We hope this article has provided you with a comprehensive guide on how to perform Cypress test automation with an example project and best practices.
Read Next :
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer