How to Handle Cypress Uncaught Exception Errors - A Quick Guide
Are you tired of dealing with Cypress test failures caused by uncaught exception errors? These errors can disrupt your test automation process, leading to wasted time and effort. Fortunately, there are several methods available to handle Cypress uncaught exception errors and ensure that your tests run smoothly.
In this article, we'll provide you with tips and methods to handle Cypress uncaught exception errors and improve the reliability of your test automation.
Understanding Cypress Uncaught Exception Errors
Uncaught exception errors occur when there is an error in your test code that is not caught and handled. This error can cause your Cypress tests to fail, leading to unreliable test automation results.
One scenario where Cypress code can fail with an uncaught exception error is when a test case tries to access an element on the page that does not exist.
For example, let's say you have a test case that verifies that a user can successfully log in to your web application. The test case uses the cy.get() command to find the username and password input fields and enters valid credentials. However, if the test case is executed on a page where the input fields have different IDs or class names than what the test expects, the cy.get() command will not find the elements, resulting in an uncaught exception error.
Here's an example code snippet that demonstrates the above scenario:
describe('Login functionality', () => {
it('should log in successfully', () => {
cy.visit('https://techoral.com/login')
// The following command tries to find the username input field, but it may not exist on the page
cy.get('#username').type('testuser')
// The following command tries to find the password input field, but it may not exist on the page
cy.get('#password').type('testpass')
cy.get('button[type="submit"]').click()
cy.url().should('include', '/dashboard')
})
})
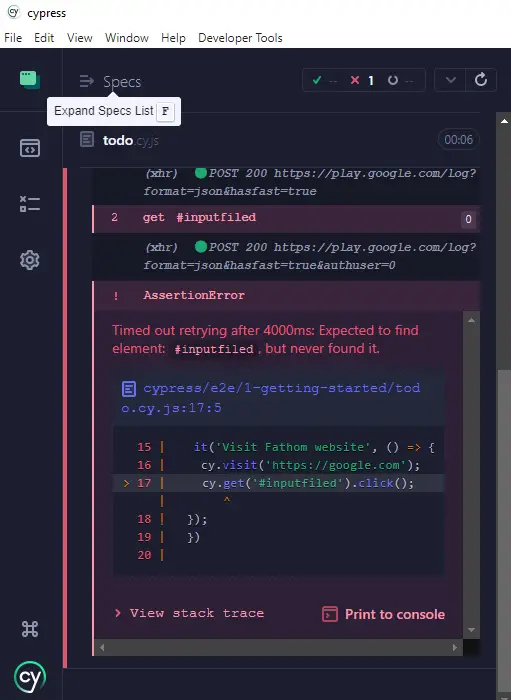
In this example, the cy.get('#username') and cy.get('#password') commands try to find the username and password input fields on the page using their IDs.
However, if the IDs of the input fields have been changed on the page, the commands will not find the elements, resulting in an uncaught exception error.
To handle this error, you can use the Cypress.on() or cy.on() method to log the error, take a screenshot, or perform any other action that you need to ensure that your test continues running.
Methods to Handle Cypress Uncaught Exception Errors
1. Use the Cypress.on() Method
The Cypress.on() method allows you to handle uncaught exception errors globally across all tests. You can use this method to log the error, take a screenshot, or perform any other action that you need to ensure that your tests continue running.
Here's an example of how to use the Cypress.on() method:
Cypress.on('uncaught:exception', (err, runnable) => {
// handle the error
// we can simply return false to avoid failing the test on uncaught error
// return false
// but a better strategy is to make sure the error is expected
if (e.message.includes('Things went bad')) {
// we expected this error, so let's ignore it
// and let the test continue
return false
}
})
2. Use the cy.on() Method
The cy.on() method allows you to handle uncaught exception errors within a specific test. You can use this method to handle the error, take a screenshot, or perform any other action that you need to ensure that your test continues running.
Here's an example of how to use the cy.on() method:
cy.on('uncaught:exception', (err, runnable) => {
// handle the error
return false
})
3. Use the Cypress.Commands.onError() Method
The Cypress.Commands.onError() method allows you to handle errors that occur within a custom Cypress command. You can use this method to log the error, take a screenshot, or perform any other action that you need to ensure that your test continues running.
Here's an example of how to use the Cypress.Commands.onError() method:
Cypress.Commands.onError((err) => {
// handle the error
})
Summary
Handling Cypress uncaught exception errors is crucial for ensuring that your test automation is reliable and provides fast feedback. By using the methods provided by Cypress, you can quickly identify and handle these errors, preventing your Cypress tests from failing due to unhandled exceptions.
Follow these tips and methods to improve the reliability of your test automation and get faster feedback.
Read Next :
- How to Install Cypress
- How to use Environment Variables in Cypress
- Cypress Installation Errors
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- How to use Environment Variables in Cypress
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer