File Upload Using Cypress: An In-Deapth Guide
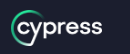
File uploads are a common functionality in modern web applications. Testing this feature is crucial as it can cause potential data loss and security issues if not implemented correctly.
Cypress, an end-to-end testing framework, provides an easy-to-use API for testing file upload functionality. In this article, we will discuss how to upload files using Cypress and various tips to make your testing efficient.
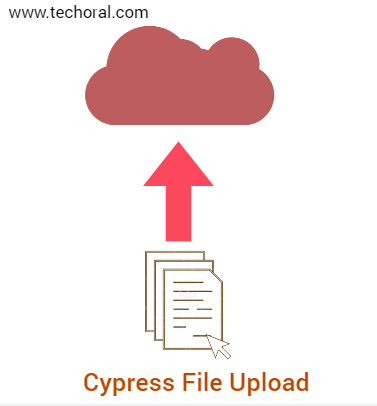
Getting Started with File Upload in Cypress
Before we dive into the details, let's get started with a basic example of uploading a file using Cypress. Cypress provides a cy.fixture() method to load a file from the fixtures folder. We can use this method to load the file and then pass it to the cy.get() command to select the file input element and attach the file.
Here's an example of how to upload a file using Cypress:
cy.fixture('techoral.txt').then(fileContent => {
cy.get('input[type="file"]').attachFile({
fileContent: fileContent.toString(),
fileName: 'techoral.txt',
mimeType: 'text/plain'
});
});
In the example above, we load a file called example.txt from the fixtures folder and then use the cy.get() command to select the file input element. We then use the attachFile() command to attach the file to the input element. The fileContent option takes the file content as a string, the fileName option specifies the name of the file, and the mimeType option specifies the MIME type of the file.
Using Cypress File Upload Plugin
Cypress also provides a file upload plugin that makes testing file uploads even easier. The plugin provides a cy.uploadFile() command that can upload files directly without using the attachFile() command. To use the plugin, we need to install it first. We can do this by running the following command in the terminal:
npm install -D cypress-file-upload
After installing the plugin, we can use the cy.uploadFile() command to upload files. Here's an example of how to use the plugin to upload a file:
cy.fixture('techoral.txt').then(fileContent => {
cy.get('input[type="file"]').uploadFile({
fileContent: fileContent.toString(),
fileName: 'example.txt',
mimeType: 'text/plain'
});
});
The plugin provides the same options as the attachFile() command, making it easy to switch between the two methods.
Handling Drag and Drop File Uploads
Some applications support drag and drop file uploads, which can be a challenge to test. Cypress provides the cy.get() command to select the file input element, but it cannot select the drag and drop area. To test drag and drop file uploads, we can use the HTML5 drag and drop API.
Here's an example of how to test drag and drop file uploads using Cypress:
cy.fixture('example.txt').then(fileContent => {
const blob = Cypress.Blob.binaryStringToBlob(fileContent.toString());
const dragEvent = new DragEvent('drop', { dataTransfer: { files: [blob] } });
cy.get('#drag-and-drop-area').trigger('dragenter').trigger('dragover').trigger('drop', dragEvent);
});
In the example above, we load the file using the cy.fixture() method and then convert it to a Blob object. We then create a DragEvent object with the drop event and pass it to the drop event of the drag and drop area. The dragenter and dragover events are also triggered to simulate a drag and drop interaction.
Troubleshooting File Uploads in Cypress
Testing file uploads can be challenging at times, especially when the file upload functionality is not working as expected. Here are some tips to help you troubleshoot file uploads in Cypress:
1. Check the file input element: Make sure that the file input element has the correct attributes and is visible on the page. Use the cy.get() command to select the file input element and assert its properties.
2. Check the file size and type: Make sure that the file size and type meet the application's requirements. Use the cy.fixture() method to load the file and then assert its size and type.
3. Check the server response: Verify that the server response is as expected. Use the cy.route() command to intercept the HTTP requests and responses and assert the server response.
4. Use the Cypress debugger: The Cypress debugger allows you to pause the test at any point and inspect the application's state. Use the cy.debug() command to open the debugger and troubleshoot the issue.
Summary:
File uploads are a common functionality in modern web applications, and testing this feature is crucial for ensuring data integrity and security. Cypress provides an easy-to-use API for testing file uploads, and the file upload plugin makes testing even easier.
By following the tips and best practices discussed in this article, you can write efficient and effective file upload tests in Cypress. Happy testing!
Read Next :
- How to Install Cypress
- Cypress Installation Errors
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- How to use Environment Variables in Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer