How to Write Custom Commands in Cypress: A Quick Guide
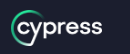
Cypress is a popular end-to-end testing framework used to test web applications. It provides a wide range of built-in commands that can be used to perform various actions on web elements.
However, sometimes these built-in commands may not be enough to test complex web applications. In such cases, we can create our own custom commands to extend the functionality of Cypress.
In this article, we will explain how to write custom commands in Cypress.
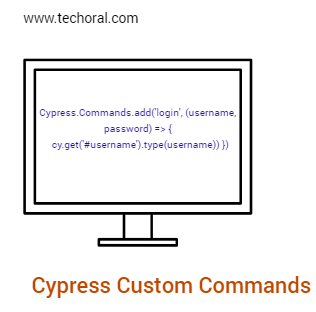
What are Custom Commands?
Custom commands are user-defined commands that can be created to perform a specific action in Cypress. They can be used to simplify repetitive tasks and make test scripts more readable and reusable. Custom commands can be created using JavaScript, and they can be called in Cypress tests just like any other built-in command.
Creating Custom Commands in Cypress
Creating custom commands in Cypress is a straightforward process. It involves adding the command to the Cypress.Commands object, which is available globally. Here's an example of how to create a custom command:
Cypress.Commands.add('login', (username, password) => {
cy.get('#username').type(username)
cy.get('#password').type(password)
cy.get('#login-button').click()
})
In this example, we have created a custom command called 'login'. This command takes two arguments, username and password, and performs the actions required to log in to the application. We can call this custom command in our Cypress tests just like any other built-in command, like this:
cy.login('testuser', 'testpassword')
This will log in to the application with the provided credentials. By using custom commands, we can simplify our tests and make them more readable.
Passing Parameters to Custom Commands
Custom commands can take parameters just like regular functions. We can pass parameters to custom commands when calling them in our tests. Here's an example of how to pass parameters to a custom command:
Cypress.Commands.add('search', (searchTerm) => {
cy.get('#search-bar').type(searchTerm)
cy.get('#search-button').click()
})
cy.search('Cypress Custom Commands')
In this example, we have created a custom command called 'search' that takes one argument, searchTerm. We can call this custom command in our tests and pass the searchTerm parameter to it.
Using Custom Commands in Chained Commands
Custom commands can be used in chained commands just like any other built-in command. Here's an example of how to use a custom command in a chained command:
Cypress.Commands.add('selectProduct', (productName) => {
cy.get('.product').contains(productName).click()
})
cy.get('.category').contains('Electronics')
.click()
.selectProduct('Smartphone')
In this example, we have created a custom command called 'selectProduct' that takes one argument, productName. We can call this custom command in a chained command and pass the productName parameter to it. This will select the product with the given name from the Electronics category.
Summary:
Custom commands in Cypress can be a powerful tool to extend the functionality of Cypress and make tests more readable and reusable.
In this article, we have explained how to create custom commands in Cypress with code examples. We have also explained how to pass parameters to custom commands and use them in chained commands. By using custom commands, you
Read Next :
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer