50+ Cypress Automation Tool Interview Questions with Answers
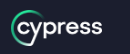
cypress-questions.png
If you're interviewing for a position that involves working with the Cypress automation tool, it's important to be prepared for the types of questions you might be asked. To help you out, we've compiled a list of 100 common Cypress automation tool interview questions and provided answers to each one.
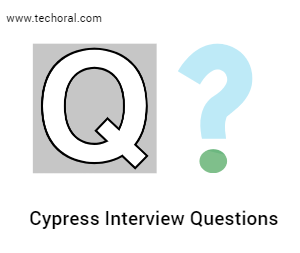
1. What is Cypress?
Cypress is an open-source end-to-end testing framework used for web applications. It allows developers to write and run automated tests that simulate how users interact with their application.
2. How does Cypress differ from other automation tools?
Cypress is unique in that it runs directly in the browser, as opposed to other automation tools that rely on a separate driver. This means that Cypress tests can more accurately simulate how a user interacts with a web application. Additionally, Cypress includes built-in debugging and time-travel features that make it easier to diagnose and fix issues in your tests.
3. How do you install Cypress?
You can install Cypress via npm by running the command: npm install cypress --save-dev
. This will install the latest version of Cypress and save it as a development dependency in your project's package.json
file.
4. What programming languages does Cypress support?
Cypress supports JavaScript and TypeScript for writing tests. It also supports BDD-style syntax using Cucumber or Mocha.
5. How do you write a basic Cypress test?
Here's an example of a basic Cypress test:
describe('My First Test', () => {
it('Visits the homepage', () => {
cy.visit('https://www.techoral.com/')
cy.contains('Welcome to techoral.com')
})
})
6. How do you select elements in Cypress?
You can select elements using CSS selectors or Cypress-specific commands like cy.get()
and cy.contains()
. For example:
// Select an element by ID
cy.get('#myElement')
// Select an element by class
cy.get('.myClass')
// Select an element by text
cy.contains('Submit')
7. How do you handle asynchronous behavior in Cypress?
Cypress automatically waits for commands to complete before moving on to the next one, so you don't need to worry about adding explicit waits or timeouts. However, if you do need to wait for an asynchronous action to complete, you can use commands like cy.wait()
or cy.get().should()
with a callback function.
8. How do you debug Cypress tests?
You can use Cypress's built-in debugger
9. What are some common mistakes or pitfalls when using Cypress?
One common mistake is not properly waiting for asynchronous actions to complete before moving on to the next command. Another pitfall is relying too much on hard-coded values that may change over time, such as element IDs or CSS classes. It's also important to keep your tests organized and maintainable, and to avoid testing implementation details rather than user-facing functionality.
10. How do you handle authentication in Cypress tests?
You can use Cypress's cy.request()
command to make HTTP requests to your server and authenticate using credentials or tokens. You can also use the cy.setCookie()
command to set cookies that contain authentication information.
11. How do you run Cypress tests in parallel?
You can use a tool like Cypress Dashboard to run tests in parallel across multiple machines or containers. You can also use a CI/CD pipeline to automatically trigger Cypress tests when code is pushed to a repository and run them in parallel using a cloud-based service.
12. How do you handle cross-browser testing in Cypress?
Cypress runs tests in the browser, so you can easily test your application in different browsers by simply specifying a different browser in the Cypress configuration file. However, it's important to keep in mind that different browsers may behave differently, so you may need to adjust your tests accordingly.
13. How do you handle testing in different environments, such as development, staging, and production?
You can use Cypress's environment variables to specify different configuration options for different environments. You can also use Cypress's cy.request()
command to make HTTP requests to different endpoints depending on the environment.
14. How do you handle testing with third-party integrations, such as APIs or external services?
You can use Cypress's cy.request()
command to make HTTP requests to APIs and external services. You can also use mocking and stubbing to simulate the behavior of external services and dependencies.
15. How do you handle testing with dynamic content, such as user-generated content or content that changes frequently?
You can use Cypress's cy.wait()
command to wait for dynamic content to load, and you can use Cypress's cy.intercept()
command to intercept and modify requests and responses. You can also use fixtures or data-driven testing to test different scenarios with different data.
16. How do you handle testing with multiple languages or locales?
You can use Cypress's cy.visit()
command to visit pages with different language or locale settings, and you can use fixtures or data-driven testing to test different scenarios with different languages or locales. You can also use browser extensions or browser settings to simulate different language or locale settings.
17. How do you handle testing with accessibility in mind?
You can use Cypress's cy.get('element').should('have.attr', 'aria-label')
command to test for accessibility attributes, and you can use a tool like axe-core to perform automated accessibility testing. It's also important to keep in mind that accessibility testing should be an ongoing process, not just a one-time check.
18. How do you handle performance testing with Cypress?
You can use Cypress's built-in metrics and timers to measure
19. How do you handle testing with file uploads in Cypress?
You can use Cypress's cy.fixture()
command to create fixture files containing test data, and you can use the cy.get('input[type="file"]').upload()
command to upload files in your tests. You can also use tools like cypress-file-upload to simplify file uploads.
20. How do you handle testing with iframes in Cypress?
You can use Cypress's cy.iframe()
command to interact with elements inside iframes, and you can use the cy.wrap()
command to chain commands together across different iframes. It's also important to ensure that the iframe you want to interact with is fully loaded before attempting to interact with its contents.
21. How do you handle testing with pop-up windows or alerts in Cypress?
You can use Cypress's cy.window()
command to interact with pop-up windows or alerts, and you can use the cy.on('window:alert')
command to capture and handle alert messages. It's important to ensure that the window or alert you want to interact with is fully loaded before attempting to interact with its contents.
22. How do you handle testing with drag and drop functionality in Cypress?
You can use Cypress's cy.get()
command to select the element you want to drag, and you can use the cy.drag()
command to simulate dragging and dropping that element to a target location. It's important to ensure that the elements you want to interact with are visible and fully loaded before attempting to drag and drop them.
23. How do you handle testing with responsive design in Cypress?
You can use Cypress's cy.viewport()
command to set the viewport size for your tests, and you can use media queries and responsive design testing tools to test your application's behavior across different screen sizes and devices.
24. How do you handle testing with animations or transitions in Cypress?
You can use Cypress's cy.wait()
command to wait for animations or transitions to complete before asserting on their results, and you can use the cy.clock()
command to control the timing of your tests. It's important to ensure that animations or transitions are fully loaded and complete before attempting to interact with them.
25. How do you handle testing with complex interactions or workflows in Cypress?
You can break down complex interactions or workflows into smaller, more manageable test cases, and you can use the cy.wrap()
and cy.then()
commands to chain together commands across multiple test cases. It's also important to keep your tests organized and easy to understand, and to avoid testing too many things at once.
26. How do you handle testing with non-English languages or character sets in Cypress?
You can use Cypress's cy.visit()
command to visit pages with different character sets or language settings, and you can use fixtures or data-driven testing to test different scenarios with different data. It's important to ensure that your application can handle non-English characters and language settings correctly.
27. How do you handle testing with complex data structures or APIs in Cypress?
You can use Cypress
28. How do you handle testing with dynamic data in Cypress?
You can use Cypress's cy.intercept()
command to intercept and modify HTTP requests and responses, and you can use the cy.wait()
command to wait for data to load before asserting on its results. It's also important to use fixtures or data-driven testing to test different scenarios with different data.
29. How do you handle testing with authentication or authorization in Cypress?
You can use Cypress's cy.login()
command to simulate logging in to your application, and you can use the cy.intercept()
command to intercept and modify HTTP requests and responses related to authentication or authorization. It's important to ensure that your application's authentication and authorization mechanisms are working correctly.
30. How do you handle testing with long-running or asynchronous processes in Cypress?
You can use Cypress's cy.wait()
command to wait for processes to complete before asserting on their results, and you can use the cy.clock()
command to control the timing of your tests. It's important to ensure that long-running or asynchronous processes are fully loaded and complete before attempting to interact with them.
31. How do you handle testing with multiple browser tabs or windows in Cypress?
You can use Cypress's cy.visit()
command to open multiple browser tabs or windows, and you can use the cy.window()
command to interact with elements in those tabs or windows. It's important to ensure that the tabs or windows you want to interact with are fully loaded before attempting to interact with their contents.
32. How do you handle testing with audio or video content in Cypress?
You can use Cypress's cy.visit()
command to visit pages with audio or video content, and you can use the cy.wait()
command to wait for the content to load before interacting with it. It's also important to ensure that your application's audio and video content is playing correctly and has the expected behavior.
33. How do you handle testing with websockets in Cypress?
You can use Cypress's cy.server()
and cy.route()
commands to set up and intercept websocket requests and responses, and you can use the cy.wait()
command to wait for data to be received before asserting on its results. It's important to ensure that your application's websocket functionality is working correctly.
34. How do you handle testing with third-party APIs or services in Cypress?
You can use Cypress's cy.request()
command to make HTTP requests to third-party APIs or services, and you can use the cy.wait()
command to wait for data to be received before asserting on its results. It's important to ensure that your application can correctly handle data from third-party APIs or services.
35. How do you handle testing with accessibility in Cypress?
You can use Cypress's cy.injectAxe()
command to integrate with the axe-core accessibility testing library, and you can use the cy.checkA11y()
command to check for accessibility issues in your application. It's important to ensure that your application is accessible to all users, including those with
36. How do you handle testing with different screen sizes or resolutions in Cypress?
You can use Cypress's cy.viewport()
command to set the screen size or resolution for your tests, and you can use the cy.screenshot()
command to take screenshots of your application at different sizes. It's important to ensure that your application looks and functions correctly on different screen sizes and resolutions.
37. How do you handle testing with drag-and-drop or other complex interactions in Cypress?
You can use Cypress's cy.get()
command to select elements for dragging and dropping, and you can use the cy.trigger()
command to simulate mouse events like mousedown
, mousemove
, and mouseup
. It's important to ensure that your application's drag-and-drop and other complex interactions work correctly.
38. How do you handle testing with date and time pickers in Cypress?
You can use Cypress's cy.get()
command to select date and time picker elements, and you can use the cy.clock()
command to control the date and time for your tests. It's important to ensure that your application's date and time pickers work correctly and handle different timezones and formats.
39. How do you handle testing with geolocation or location-based features in Cypress?
You can use Cypress's cy.visit()
command to visit pages with geolocation or location-based features, and you can use the cy.window()
command to interact with the browser's geolocation API. It's important to ensure that your application's geolocation and location-based features work correctly and handle different locations and devices.
40. How do you handle testing with browser-specific behavior or features in Cypress?
You can use Cypress's cy.visit()
command to visit pages with browser-specific behavior or features, and you can use the cy.window()
command to interact with the browser's APIs and features. It's important to ensure that your application's browser-specific behavior and features work correctly and handle different browsers and versions.
41. How do you handle testing with cookies or local storage in Cypress?
You can use Cypress's cy.setCookie()
and cy.getCookie()
commands to set and get cookies, and you can use the cy.clearLocalStorage()
and cy.getLocalStorage()
commands to clear and get items from local storage. It's important to ensure that your application's cookies and local storage are being set and read correctly.
42. How do you handle testing with forms or input validation in Cypress?
You can use Cypress's cy.get()
command to select form elements, and you can use the cy.type()
command to simulate user input. It's important to ensure that your application's forms and input validation are working correctly and handle different inputs and edge cases.
43. How do you handle testing with animations or transitions in Cypress?
You can use Cypress's cy.wait()
command to wait for animations or transitions to complete before asserting on their results, and you can use the cy.clock()
command to
44. How do you handle testing with pop-ups or modals in Cypress?
You can use Cypress's cy.get()
command to select pop-up or modal elements, and you can use the cy.contains()
command to interact with their content. It's important to ensure that your application's pop-ups or modals are working correctly and can be closed or dismissed.
45. How do you handle testing with third-party integrations or APIs in Cypress?
You can use Cypress's cy.request()
command to make HTTP requests to third-party integrations or APIs, and you can use the cy.route()
command to intercept and stub responses. It's important to ensure that your application's third-party integrations or APIs are working correctly and handle different inputs and responses.
46. How do you handle testing with security or authentication in Cypress?
You can use Cypress's cy.request()
command to make authenticated requests, and you can use the cy.route()
command to intercept and stub authentication responses. It's important to ensure that your application's security and authentication mechanisms are working correctly and handle different inputs and scenarios.
47. How do you handle testing with websockets or real-time updates in Cypress?
You can use Cypress's cy.wait()
command to wait for websockets or real-time updates to complete before asserting on their results, and you can use the cy.server()
and cy.route()
commands to intercept and stub responses. It's important to ensure that your application's websockets or real-time updates are working correctly and handle different scenarios.
48. How do you handle testing with audio or video playback in Cypress?
You can use Cypress's cy.get()
command to select audio or video elements, and you can use the cy.wait()
command to wait for playback to complete before asserting on the results. It's important to ensure that your application's audio or video playback is working correctly and handle different formats and scenarios.
49. How do you handle testing with internationalization or localization in Cypress?
You can use Cypress's cy.get()
command to select elements with internationalized or localized content, and you can use the cy.contains()
command to assert on the correct content. It's important to ensure that your application's internationalization or localization is working correctly and handle different languages and locales.
50. How do you handle testing with accessibility or screen readers in Cypress?
You can use Cypress's cy.get()
command to select elements with accessible or screen reader content, and you can use the cy.contains()
command to assert on the correct content. It's important to ensure that your application's accessibility or screen reader content is working correctly and handle different disabilities and scenarios.
51. How do you handle testing with GraphQL APIs in Cypress?
You can use Cypress's cy.request()
command to make GraphQL queries to APIs, and you can use the cy.route()
command to intercept and stub responses. It's important to ensure that your application's GraphQL APIs are working correctly and handle different queries and responses.
52. How
53. How do you handle testing with file uploads in Cypress?
You can use Cypress's cy.fixture()
command to load a file, and you can use the cy.get()
command to select the file upload element and cy.upload()
command to upload the file. It's important to ensure that your application's file upload functionality is working correctly and handle different file types and sizes.
54. How do you handle testing with drag and drop functionality in Cypress?
You can use Cypress's cy.get()
command to select the drag and drop elements, and you can use the cy.drag()
and cy.drop()
commands to simulate dragging and dropping. It's important to ensure that your application's drag and drop functionality is working correctly and handle different scenarios and browsers.
55. How do you handle testing with scrolling or pagination in Cypress?
You can use Cypress's cy.scrollTo()
command to scroll to a specific element, and you can use the cy.wait()
command to wait for pagination to complete before asserting on the results. It's important to ensure that your application's scrolling or pagination functionality is working correctly and handle different scenarios and layouts.
56. How do you handle testing with dynamic or changing elements in Cypress?
You can use Cypress's cy.get()
command with the force: true
option to select dynamically generated or changing elements, and you can use the cy.wait()
command to wait for the element to appear before asserting on it. It's important to ensure that your application's dynamic or changing elements are working correctly and handle different scenarios and states.
57. How do you handle testing with multiple browser windows or tabs in Cypress?
You can use Cypress's cy.visit()
command with the {failOnStatusCode: false}
option to visit a different URL in a new browser window or tab, and you can use the cy.window()
and cy.getCookie()
commands to interact with the new window or tab. It's important to ensure that your application's multiple browser windows or tabs functionality is working correctly and handle different scenarios and browsers.
58. How do you handle testing with responsive design or mobile views in Cypress?
You can use Cypress's cy.viewport()
command to set the viewport size to test responsive design or mobile views, and you can use the cy.get()
command to select elements and cy.contains()
command to assert on their content. It's important to ensure that your application's responsive design or mobile views are working correctly and handle different devices and screen sizes.
59. How do you handle testing with long-running or performance-heavy tests in Cypress?
You can use Cypress's cy.clock()
command to control the time and speed up or slow down your tests, and you can use the cy.task()
command to offload heavy processing to a separate task. It's important to ensure that your application's long-running or performance-heavy tests are optimized and run efficiently.
Read Next :
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer