Selenium Reporting: How to Generate Detailed Test Reports with Code Examples
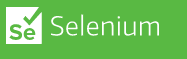
Selenium is a popular automation testing tool used for web application testing. When running a large number of tests, it can be difficult to analyze the results and identify issues. This is where reporting comes in. Reporting helps to generate detailed reports that provide insights into the test results and highlight areas that require attention.
In this article, we will explore the various reporting frameworks and tools available for Selenium and how to generate detailed test reports using them. We will also provide code examples to illustrate how to implement these frameworks.
Reporting Frameworks and Tools for Selenium
1. TestNG Reports:
TestNG is a popular testing framework for Java-based applications. It provides built-in reporting functionality that generates detailed HTML reports for test execution results. TestNG reports provide information on the number of tests run, the number of tests passed and failed, the execution time, and more.
2. Extent Reports:
Extent Reports is an open-source reporting framework for Java and .NET-based applications. It provides detailed and interactive HTML reports that can be easily customized. Extent Reports provide features such as screenshots, logging, and attachments to help in identifying issues.
3. Allure Reports:
Allure Reports is an open-source reporting framework that generates detailed HTML reports with features such as graphical representations, history trends, and attachments. It is compatible with various test frameworks including TestNG, JUnit, and Cucumber.
Generating Test Reports in Selenium with Code Examples
Let's take a look at how to generate test reports using TestNG and Extent Reports.
TestNG Reports:
To generate TestNG reports, you need to add the following code to your test script:
import org.testng.annotations.AfterMethod;
import org.testng.annotations.AfterSuite;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.BeforeSuite;
import org.testng.annotations.Test;
import org.testng.Assert;
import org.testng.Reporter;
import org.testng.annotations.*;
public class TestReportExample {
@BeforeSuite
public void beforeSuite() {
// Code for before suite
}
@BeforeMethod
public void beforeMethod() {
// Code for before method
}
@Test
public void testMethod() {
// Code for test method
}
@AfterMethod
public void afterMethod() {
// Code for after method
}
@AfterSuite
public void afterSuite() {
// Code for after suite
}
}
In this example, we have added the necessary annotations for TestNG reporting. The @BeforeSuite and @AfterSuite annotations are used to define actions that should occur before and after the test suite. The @BeforeMethod and @AfterMethod annotations are used to define actions that should occur before and after each test method. The @Test annotation is used to define the test method.
You can also add listeners to your TestNG suite to generate more detailed reports. TestNG provides various listeners that you can use to generate customized reports. To add a listener to your TestNG suite, you can use the following code:
import org.testng.annotations.Listeners;
@Listeners(TestListener.class)
public class TestClass {
// Test code here
}
In the above code, we have added the @Listeners annotation and specified the name of the listener class (TestListener) that we want to use. You can create your own listener class by implementing the TestNG ITestListener interface.
Extent Reports:
To use the Extent report library, first, you need to add the dependency to your project:
com.aventstack
extentreports
4.1.5
Then, you can use the following code to create a basic Extent report:
ExtentReports extent = new ExtentReports();
ExtentTest test = extent.createTest("Test Name", "Test Description");
test.log(Status.PASS, "Test Step Passed");
extent.flush();
You can also add more details to your report by using different logging levels such as Info, Warning, Error, etc.
Allure Report:
To use the Allure report library, first, you need to add the dependency to your project:
io.qameta.allure
allure-testng
2.13.7
Then, you can use the following code to create a basic Allure report:
import io.qameta.allure.Allure;
import io.qameta.allure.Description;
import io.qameta.allure.Feature;
import io.qameta.allure.Story;
import org.testng.annotations.Test;
public class TestClass {
@Test
@Feature("Feature Name")
@Story("Story Name")
@Description("Test Description")
public void testMethod() {
// Test Code Here
Allure.addAttachment("Attachment Name", "Attachment Content");
}
}
In the above code, we have added annotations such as @Feature, @Story, and @Description to provide more details about the test.
You can also add attachments to your report using the Allure.addAttachment() method.
Note: Both Extent and Allure reporting libraries provide different customization options and features that you can explore in their respective documentation.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer