Selenium Handling Frames and Windows: A Comprehensive Guide with Examples
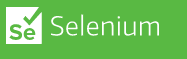
Frames and windows are common features in web applications and can be challenging to handle when writing automated tests with Selenium.
In this article, we will cover everything you need to know about handling frames and windows in Selenium.
To get started, let's first understand what frames and windows are. Frames are HTML documents that are embedded within other HTML documents.
Windows are separate browser windows that can be opened by a web application. Selenium can interact with frames and windows in a variety of ways, depending on your testing requirements.
Here's an example of how to switch to a frame in Selenium with Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class FrameExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.techoral.com/");
driver.switchTo().frame("frame-name");
WebElement element = driver.findElement(By.id("frame-element"));
System.out.println(element.getText());
driver.quit();
}
}
In this example, we have created a new Selenium test that switches to a frame on a web page using the switchTo() method and the frame's name. We then find an element within the frame using the findElement() method and output its text to the console.
Handling windows can be more complicated than frames, as we need to keep track of multiple browser windows and switch between them as needed. Here's an example of how to handle a new window in Selenium with Java:
import java.util.Set;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class WindowExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.techoral.com/");
String parentWindowHandle = driver.getWindowHandle();
driver.findElement(By.id("new-window-button")).click();
Set windowHandles = driver.getWindowHandles();
for (String windowHandle : windowHandles) {
if (!windowHandle.equals(parentWindowHandle)) {
driver.switchTo().window(windowHandle);
WebElement element = driver.findElement(By.id("window-element"));
System.out.println(element.getText());
driver.close();
}
}
driver.switchTo().window(parentWindowHandle);
driver.quit();
}
}
In this example, we have created a new Selenium test that opens a new window when a button is clicked on a web page.
We then switch to the new window using the switchTo() method and the window's handle, find an element within the window using the findElement() method, and output its text to the console. We then close the window using the close() method and switch back to the parent window using the switchTo() method.
In conclusion, handling frames and windows in Selenium is an essential skill for writing robust and reliable automated tests.
With the examples and best practices outlined in this article, you should be well-equipped to handle frames and windows in your Selenium tests.
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer