Selenium with Java Tutorial - Learn Selenium WebDriver with Java
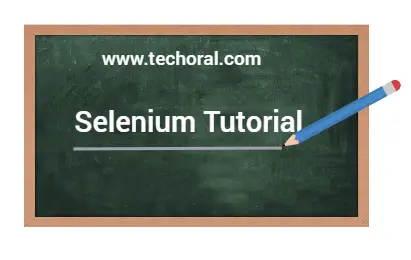
- Introduction to Selenium
- Setting up the Selenium Environment
- Using Locators in Selenium
- Understanding Waits in Selenium
- Performing Actions on Web Elements
- Introduction to TestNG
- Implementing Page Objects
- Implementing Data-Driven Testing
- Performing Cross-Browser Testing
- Integrating Selenium with Continuous Integration
- Selenium Grid
- Advanced Topics
Introduction to Selenium
In this section, we'll introduce Selenium, an open-source automation testing tool for web applications. We'll cover:
- What is Selenium and How Does it Work?
- Benefits and Limitations of Using Selenium
- Getting Started with Selenium WebDriver
- Creating Your First Selenium Test
Please refer Introduction to Selenium , Installation and more
Setting up the Selenium Environment
In this section, we'll cover how to set up your environment for Selenium testing, including:
- Installing and Configuring the Java Development Kit (JDK)
- Installing and Configuring Eclipse IDE
- Setting Up Selenium WebDriver with Eclipse
- Configuring Chrome Driver for Selenium
Please refer Selenium webdriver, eclipse and chrome setup and more
Using Locators in Selenium
In this section, we'll explore how to use locators in Selenium to identify and interact with web elements on a web page. We'll cover:
- Understanding Locators in Selenium
- Using ID, Name, Class Name, and Tag Name Locators
- Using CSS Selector and XPath Locators
- Best Practices for Locators in Selenium
Please refer Selenium Locators, Selector / XPath / Tag / CSS and more
Understanding Waits in Selenium
In this section, we'll cover how to use waits in Selenium to ensure that your tests run reliably and consistently, even if the page takes some time to load or if elements on the page take some time to become visible. We'll cover:
- Understanding Waits in Selenium
- Types of Waits in Selenium
- Using Explicit Waits in Selenium
- Using Implicit Waits in Selenium
Please refer Selenium Implicit / Explicit waits and more
Performing Actions on Web Elements
In this section, we'll explore how to perform various actions on web elements in Selenium, including:
- Clicking, Typing, and Selecting Values from Dropdowns
- Handling Alerts and Popups in Selenium
- Using JavaScript Executor in Selenium
- Handling Frames and Windows in Selenium
Introduction to TestNG
In this section, we'll introduce TestNG, a powerful testing framework for Java applications, and explore how to use it with Selenium. We'll cover:
- What is TestNG and How Does it Work?
- Benefits and Limitations of Using TestNG with Selenium
- Installing TestNG and Creating a TestNG Project
- Creating and Running TestNG Tests with Selenium
Please refer TestNG introduction, installation and more
Implementing Page Objects
In this section, we'll explore the Page Object Model (POM) design pattern, which is widely used in Selenium testing to create reusable and maintainable tests. We'll cover:
- Understanding the Page Object Model (POM)
- Creating Page Objects in Selenium
- Refactoring Tests with Page Objects
- Best Practices for Implementing POM in Selenium
Please refer Page Object Model (POM) Pattern and more
Data-Driven Testing with Selenium and Apache POI
Data-driven testing is an essential part of Selenium testing that enables us to run the same test script with multiple sets of data. In this section, we'll explore how to implement data-driven testing with Selenium and Apache POI, a popular Java library for working with Microsoft Office documents. We'll cover:
- Understanding Data-Driven Testing
- Creating Data-Driven Tests with Apache POI
- Reading Data from Excel Sheets in Selenium Tests
- Best Practices for Data-Driven Testing in Selenium
Cross-Browser Testing with Selenium and Java
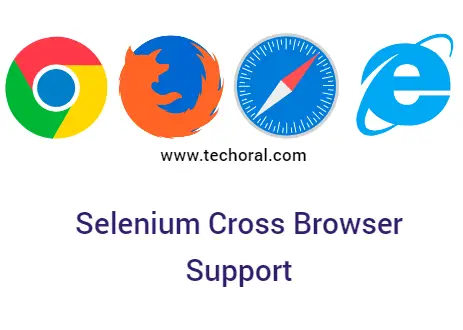
Cross-browser testing is a critical aspect of Selenium testing that ensures that web applications are compatible with different browsers and their versions. In this section, we'll explore how to perform cross-browser testing with Selenium and Java. We'll cover:
- Understanding Cross-Browser Testing
- Creating Cross-Browser Tests with Selenium and Java
- Testing with Different Browsers and Versions
- Best Practices for Cross-Browser Testing in Selenium
Please refer Selenium Cross Browser and more
Continuous Integration with Selenium and Jenkins
Continuous integration (CI) is a software development practice that involves automatically building, testing, and deploying software changes. In this section, we'll explore how to set up a continuous integration pipeline for Selenium tests using Jenkins, a popular open-source automation server. We'll cover:
- Understanding Continuous Integration with Selenium
- Installing and Configuring Jenkins
- Setting up a Jenkins Job for Selenium Tests
- Best Practices for Continuous Integration with Selenium
Distributed Testing with Selenium Grid
Selenium Grid is a tool that enables you to run Selenium tests on multiple machines at the same time, speeding up test execution and reducing the time it takes to get feedback on test results. In this section, we'll explore how to set up and use Selenium Grid for distributed testing. We'll cover:
- Understanding Distributed Testing with Selenium Grid
- Setting up Selenium Grid
- Configuring Selenium Tests to Run on Selenium Grid
- Best Practices for Distributed Testing with Selenium Grid
Advanced Topics in Selenium with Java
In this section, we'll cover some advanced topics in Selenium with Java, including:
- Handling Dynamic Web Elements in Selenium
- Testing with Different Browsers and Devices in Selenium
- Integrating Selenium with Other Tools and Frameworks
- Best Practices for Advanced Topics in Selenium
Read Next :
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Selenium Tutorials
- Setting up selenium IDE - Download and install selenium IDE on windows
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
- Selenium WebDriver API
- Selenium WebDriver Setup
- Selenium Maven Dependancy
- Selenium Locators
- Selenium with Maven
- Selenium Page Object Model (POM)
- Selenium TestNG
- Selenium Handling Alerts
- Selenium Handling Frames and Windows
- Selenium Waits
- Selenium Cross-browser Testing
- Selenium Reporting
- Selenium Stale Element Exception
- TestNg Class Version Error
- Selenium IDE Download & Installation on Chrome
- Selenium IDE Download & Installation on Microsoft Edge
- Selenium IDE Download & Installation on Mozilla Firefox
- Selenium vs Cypress vs Puppeteer
- Selenium Interview Questions
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer