Debugging Cypress Tests in AWS Environments using AWS X-Ray
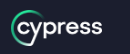
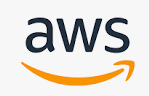
Are you struggling to debug Cypress tests in your AWS environment? Look no further than AWS X-Ray. With AWS X-Ray, you can quickly and easily identify the root cause of issues in your tests, saving you time and effort.
Here are some tips and tricks for using AWS X-Ray to debug your Cypress tests in AWS environments:
Enable X-Ray tracing for your AWS environment.
Before you can begin using AWS X-Ray, you need to enable tracing for your environment. This can be done through the AWS Management Console, AWS CLI, or AWS SDKs. Once tracing is enabled, AWS X-Ray will automatically collect trace data for your environment.
Integrate Cypress with AWS X-Ray.
To use AWS X-Ray with Cypress, you'll need to integrate the two services. This can be done by installing the aws-xray-sdk package and configuring it in your Cypress tests.
Use X-Ray to trace your test executions.
Once you've integrated Cypress with AWS X-Ray, you can start tracing your test executions. AWS X-Ray will capture a trace for each test execution, showing you the path that the request took through your environment.
Analyze trace data to identify issues.
With AWS X-Ray, you can easily analyze trace data to identify issues in your tests. The trace data will show you where errors occurred and which services were involved, allowing you to quickly pinpoint the root cause of any issues.
Optimize your debugging process with X-Ray Insights.
X-Ray Insights is a powerful feature that can help you optimize your debugging process. With Insights, you can create custom views of your trace data, allowing you to focus on the data that's most relevant to your debugging efforts.
AWS X-Ray with Cypress: Example
Here's an example of how you can integrate AWS X-Ray with Cypress tests:
Enable X-Ray tracing for your AWS environment:
const AWSXRay = require('aws-xray-sdk');
AWSXRay.captureAWS(require('aws-sdk'));
Integrate Cypress with AWS X-Ray:
const AWSXRay = require('aws-xray-sdk');
const aws = AWSXRay.captureAWS(require('aws-sdk'));
// configure AWS X-Ray
AWSXRay.middleware.setSamplingRules({
rules: [
{
description: 'Capture all requests',
host: '*',
http_method: '*',
url_path: '*',
fixed_target: 1,
rate: 1.0,
},
],
});
// configure Cypress to use X-Ray
Cypress.on('before:browser:launch', (browser, launchOptions) => {
launchOptions.args.push('--remote-debugging-port=9222');
return new Promise(resolve => {
const xrayPlugin = new AWSXRay.plugins.CaptureAWSPlugin(aws);
AWSXRay.enableManualMode(xrayPlugin);
const intervalId = setInterval(() => {
if (!xrayPlugin.getEmitter()) {
return;
}
clearInterval(intervalId);
resolve();
}, 100);
});
});
Cypress.Commands.add('xray', (name, fn) => {
AWSXRay.captureAsyncFunc(name, (subsegment) => {
cy.wrap(fn(subsegment)).then(() => {
subsegment.close();
});
});
});
Use X-Ray to trace your test executions:
describe('My Cypress test suite', () => {
it('should do something', () => {
cy.xray('my-test', (subsegment) => {
// perform your test logic here
});
});
});
With this code, AWS X-Ray will capture traces for each test execution, and you can use the AWS X-Ray console to analyze the trace data and identify any issues in your tests. You can also use the xray command to wrap your test logic and capture subsegments within your trace.
In conclusion, AWS X-Ray is a powerful tool for debugging Cypress tests in AWS environments. By following these tips and tricks, you can quickly and easily identify and resolve issues in your tests, improving the quality of your application and saving time and effort in the process.
Read Next :
- Setting up a test environment for Cypress with AWS services
- Integrating Cypress with AWS CodePipeline for continuous testing and deployment
- Using AWS Lambda functions in Cypress tests for serverless testing
- Managing test data with AWS DynamoDB in Cypress tests
- Creating visual regression tests with AWS Visual Testing in Cypress
- Load testing AWS applications with Cypress and AWS Load Testing services
- Monitoring AWS CloudWatch metrics with Cypress tests for improved observability
- Debugging Cypress tests in AWS environments using AWS X-Ray.
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer