How to Effectively Load Test Your AWS Applications with Cypress and AWS Load Testing Services
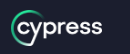
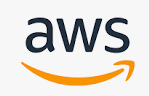
Load testing is an essential aspect of ensuring the reliability and scalability of any AWS application. It involves simulating real-world user traffic to measure how well an application can handle high levels of usage.
Effective load testing helps identify potential bottlenecks and performance issues before they impact end-users.
In this article, we’ll discuss how you can use Cypress and AWS Load Testing services to perform effective load testing on your AWS applications.
Cypress is a popular open-source end-to-end testing framework that is ideal for load testing AWS applications. It offers a simple and easy-to-use interface for testing user interactions, making it an excellent tool for simulating user traffic. Cypress is also highly customizable, allowing you to configure your tests to suit your specific needs.
AWS offers several load testing services, including Amazon CloudWatch, Amazon Elastic Load Balancing, and AWS Load Testing services. These services allow you to simulate high levels of traffic and test your application's performance under heavy loads.
To get started with load testing your AWS applications using Cypress and AWS Load Testing services, follow these steps:
#1 Set up your Cypress environment by installing Cypress and creating a new project.
#2 Write Cypress tests that simulate user interactions on your application.
#3 Set up your AWS Load Testing service by selecting the appropriate service and configuring the load test.
#4 Run your Cypress tests using the AWS Load Testing service to simulate real-world traffic.
By using Cypress and AWS Load Testing services together, you can effectively test the performance and reliability of your AWS applications. This approach provides a comprehensive testing solution that helps ensure your application can handle high levels of usage and maintain its performance over time.
Here's an example of how to perform load testing for a simple AWS application using Cypress and AWS Load Testing services:
Prerequisites:
- An AWS account with access to AWS Load Testing services
- Node.js and npm installed on your local machine
- Create a new Cypress project
- In the Cypress Test Runner, create a new file cypress/integration/load_testing_spec.js with the following contents:
- Save and close the Test Runner.
- Install the AWS SDK for JavaScript
- In the project root, create a new file load-testing.js with the following contents:
- In the AWS console, create a new Test Plan and copy the ARN.
- Update the testPlan object in load-testing.js with the ARN of your Test Plan and other relevant details.
- Run the load test:
-
node load-testing.js
mkdir cypress-load-testing-example
cd cypress-load-testing-example
npm init -y
npm install cypress --save-dev
npx cypress open
describe('Load Testing Example', () => {
it('Visits the home page', () => {
cy.visit('https://your-application-url.com')
})
})
npm install aws-sdk --save
const AWS = require('aws-sdk')
const { exec } = require('child_process')
AWS.config.update({
accessKeyId: 'your-access-key-id',
secretAccessKey: 'your-secret-access-key',
region: 'your-aws-region'
})
const loadtest = new AWS.LoadTesting({
apiVersion: '2020-05-03'
})
const testPlan = {
// Define your test plan here
}
loadtest.createTest({
Name: 'Load Testing Example',
Type: 'HTTP',
TestPlan: JSON.stringify(testPlan)
}, (err, data) => {
if (err) {
console.log(err, err.stack)
} else {
console.log('Load test started:', data.LoadTestArn)
// Wait for the test to complete
const interval = setInterval(() => {
loadtest.describeTest({
LoadTestArn: data.LoadTestArn
}, (err, data) => {
if (err) {
console.log(err, err.stack)
} else {
if (data.Test.Status === 'COMPLETED') {
console.log('Load test completed:', data.Test.Status)
// Download the load test report
const reportFile = `loadtest-report-${data.Test.StartTime}.html`
exec(`aws loadtesting download-report --report-arn ${data.Test.Reports[0].Arn} --output-file ${reportFile}`, (err, stdout, stderr) => {
if (err) {
console.error(err)
} else {
console.log('Load test report downloaded:', reportFile)
}
})
clearInterval(interval)
} else {
console.log('Load test status:', data.Test.Status)
}
}
})
}, 5000)
}
})
This example code performs a basic load test on the home page of your application using Cypress and AWS Load Testing services. You can customize the test plan to suit your specific needs and test multiple pages and user interactions.
Note: Be sure to replace the placeholders in the code (e.g., your-application-url.com, your-access-key-id, etc.) with your own values.
Analyze the load test report to identify performance bottlenecks and areas for improvement in your application. You can view the report by opening the loadtest-report-{timestamp}.html file that was downloaded in step 8.
Iterate and improve your application based on the insights gained from the load test results.
By using Cypress and AWS Load Testing services, you can easily perform load testing on your AWS application to ensure that it can handle high traffic and loads. With this approach, you can identify and fix performance issues before they impact end users, leading to a better user experience and higher user satisfaction.
In summary, load testing is a critical aspect of AWS application development that helps to ensure your application can handle high traffic and loads. Using Cypress and AWS Load Testing services together, you can easily create and execute load tests on your application and gain valuable insights into its performance.
By analyzing the results of these load tests, you can make improvements to your application to ensure it delivers the best possible user experience.
Read Next :
- Setting up a test environment for Cypress with AWS services
- Integrating Cypress with AWS CodePipeline for continuous testing and deployment
- Using AWS Lambda functions in Cypress tests for serverless testing
- Managing test data with AWS DynamoDB in Cypress tests
- Creating visual regression tests with AWS Visual Testing in Cypress
- Load testing AWS applications with Cypress and AWS Load Testing services
- Monitoring AWS CloudWatch metrics with Cypress tests for improved observability
- Debugging Cypress tests in AWS environments using AWS X-Ray.
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer