Efficient Test Data Management with AWS DynamoDB in Cypress Tests
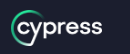
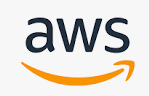
In this article, we will discuss how to leverage the power of DynamoDB to manage test data in Cypress tests and explore its benefits.
Why Use AWS DynamoDB for Test Data Management?
Traditionally, test data is managed using static files or databases, which can lead to several challenges. Static files can become difficult to manage as the number of test cases and data grows. Moreover, if the application's data model changes, updating the test data can become a cumbersome process.
Databases can be a good option for managing test data, but it can be challenging to set up and maintain a test database environment. In addition, test data can be contaminated by manual testing or other automated tests, leading to unreliable test results.
AWS DynamoDB is a fully-managed NoSQL database service that is designed for high scalability, performance, and reliability. It provides flexible data models and fast, consistent performance for any workload. With DynamoDB, developers can easily create, modify, and delete test data programmatically, making it an ideal solution for test automation.
How to Use AWS DynamoDB in Cypress Tests?
To use AWS DynamoDB in Cypress tests, developers can use the AWS SDK for JavaScript, which provides APIs for interacting with DynamoDB. Here's an example of how to use DynamoDB to manage test data in Cypress tests:
Create a DynamoDB table for test data: Using the AWS Management Console or AWS CLI, developers can create a DynamoDB table for test data. The table can have a simple or complex data model, depending on the application's requirements.
Write test data to DynamoDB: In the Cypress test script, developers can use the AWS SDK to write test data to the DynamoDB table. For example, if the test case requires creating a new user, developers can use the putItem API to write the user's data to the DynamoDB table.
Read test data from DynamoDB: In the Cypress test script, developers can use the getItem API to read test data from the DynamoDB table. For example, if the test case requires verifying a user's login credentials, developers can use the getItem API to read the user's data from the DynamoDB table.
Delete test data from DynamoDB: After the test case is executed, developers can use the deleteItem API to delete test data from the DynamoDB table. This ensures that the test data is cleaned up after each test run.
Benefits of Using AWS DynamoDB for Test Data Management
- Using AWS DynamoDB for test data management in Cypress tests provides several benefits, including:
- Scalability: DynamoDB is designed for high scalability, allowing developers to manage test data for any workload.
- Performance: DynamoDB provides fast and consistent performance, enabling developers to write and read test data quickly.
- Flexibility: DynamoDB supports flexible data models, allowing developers to create tables with simple or complex data structures.
- Programmatic Data Management: DynamoDB provides APIs for programmatically managing test data, making it easy to create, modify, and delete test data in Cypress tests.
- Reliability: DynamoDB is a fully-managed service provided by AWS, ensuring high availability and reliability.
- Setting up a test environment for Cypress with AWS services
- Integrating Cypress with AWS CodePipeline for continuous testing and deployment
- Using AWS Lambda functions in Cypress tests for serverless testing
- Managing test data with AWS DynamoDB in Cypress tests
- Creating visual regression tests with AWS Visual Testing in Cypress
- Load testing AWS applications with Cypress and AWS Load Testing services
- Monitoring AWS CloudWatch metrics with Cypress tests for improved observability
- Debugging Cypress tests in AWS environments using AWS X-Ray.
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress with DynamoDB : TODO example
Here's an example of how to use AWS DynamoDB in Cypress tests:
Let's say we have a web application that allows users to create and manage their to-do lists. We want to write automated tests using Cypress to ensure that users can create, edit, and delete their to-do lists successfully.
To manage test data in Cypress tests using DynamoDB, we can follow these steps:
Create a DynamoDB table for test data: Using the AWS Management Console or AWS CLI, we can create a DynamoDB table called "todos" with a partition key of "userId" and a sort key of "taskId". This table will store the to-do lists created by users during the test runs.
Write test data to DynamoDB: In our Cypress test script, we can use the AWS SDK for JavaScript to write test data to the DynamoDB table. For example, to create a new to-do list, we can use the putItem API to write the to-do list data to the DynamoDB table:
import AWS from 'aws-sdk';
const docClient = new AWS.DynamoDB.DocumentClient();
describe('To-Do List Tests', () => {
it('Should create a new to-do list', () => {
const userId = 'user1';
const taskId = 'task1';
const taskName = 'Buy groceries';
const params = {
TableName: 'todos',
Item: {
userId: userId,
taskId: taskId,
taskName: taskName
}
};
// Write the to-do list data to DynamoDB
return docClient.put(params).promise()
.then(() => {
// Perform the test steps
...
});
});
});
Read test data from DynamoDB: In our Cypress test script, we can use the getItem API to read test data from the DynamoDB table. For example, to verify that a to-do list was created successfully, we can use the getItem API to read the to-do list data from the DynamoDB table:
describe('To-Do List Tests', () => {
it('Should create a new to-do list', () => {
const userId = 'user1';
const taskId = 'task1';
const taskName = 'Buy groceries';
const params = {
TableName: 'todos',
Key: {
userId: userId,
taskId: taskId
}
};
// Read the to-do list data from DynamoDB
return docClient.get(params).promise()
.then((result) => {
// Verify that the to-do list data is correct
expect(result.Item).to.deep.equal({
userId: userId,
taskId: taskId,
taskName: taskName
});
// Perform the remaining test steps
...
});
});
});
Delete test data from DynamoDB: After the test case is executed, we can use the deleteItem API to delete test data from the DynamoDB table. This ensures that the test data is cleaned up after each test run:
describe('To-Do List Tests', () => {
it('Should create a new to-do list', () => {
const userId = 'user1';
const taskId = 'task1';
const taskName = 'Buy groceries';
const params = {
TableName: 'todos',
Key: {
userId: userId,
taskId: taskId
}
};
// Delete the to-do list data from DynamoDB
return docClient.delete(params).promise()
.then(() => {
// Perform the remaining test steps
...
});
});
By using DynamoDB to manage test data in Cypress tests, we can ensure that our tests are repeatable and reliable. We can also easily set up test data for our test cases and clean up the data after each test run.
In addition to managing test data, DynamoDB can also be used for other purposes in Cypress tests, such as storing test configuration data, tracking test results, and generating test reports. With its scalability, flexibility, and high availability, DynamoDB is a great tool to use in automated testing with Cypress.
Conclusion
In conclusion, managing test data in Cypress tests using AWS DynamoDB can help developers overcome the challenges of traditional test data management methods. It provides scalability, performance, flexibility,
Read Next :
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer