Creating visual regression tests with AWS Visual Testing in Cypress
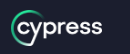
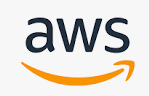
In today's fast-paced development environment, it's important to ensure that your web application is consistent across different browsers and devices. One way to achieve this is through visual regression testing, which compares the visual output of a new version of your application with its previous version.
AWS Visual Testing is a powerful tool that can help you automate your visual regression testing process. It integrates seamlessly with Cypress, a popular end-to-end testing framework, and allows you to easily create visual tests for your web application.
In this article, we'll walk you through the process of setting up AWS Visual Testing in Cypress and creating your first visual regression test.
Here's a detailed example of how to use cypress-visual-regression with AWS Visual Testing to perform visual regression testing in Cypress:
Step 1: Install Required Packages
First, you'll need to install cypress-visual-regression and the AWS SDK for JavaScript:
npm install --save-dev cypress-visual-regression
npm install --save aws-sdk
Step 2: Set Up AWS Credentials
Next, you'll need to set up AWS credentials so that Cypress can interact with your S3 bucket. You can create a new IAM user with appropriate permissions, and then store the access key ID and secret access key in environment variables:
export AWS_ACCESS_KEY_ID=your_access_key_id
export AWS_SECRET_ACCESS_KEY=your_secret_access_key
Step 3: Add Cypress Plugin
You'll need to add the cypress-visual-regression plugin to your Cypress configuration file (cypress/plugins/index.js):
const {
addMatchImageSnapshotPlugin,
} = require('cypress-visual-regression/dist/plugin');
module.exports = (on, config) => {
addMatchImageSnapshotPlugin(on, config);
};
Step 4: Set Up AWS S3 Bucket
Create a new S3 bucket in the AWS Management Console to store the baseline screenshots and diffs. Once created, you'll need to configure the bucket's permissions to allow public read access to the screenshots and diffs:
Select the bucket and choose the "Permissions" tab.
Click "Bucket Policy" and enter the following policy:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": ["s3:GetObject"],
"Resource": ["arn:aws:s3:::your-bucket-name/*"]
}
]
}
Replace "your-bucket-name" with the actual name of your S3 bucket.
Step 5: Set Up Cypress Configuration
Add the following configuration options to your Cypress configuration file (cypress.json):
{
"viewportWidth": 1280,
"viewportHeight": 800,
"defaultCommandTimeout": 10000,
"baseUrl": "https://techoral.com",
"screenshotsFolder": "cypress/screenshots",
"trashAssetsBeforeRuns": true,
"env": {
"awsBucketName": "your-bucket-name",
"awsBucketRegion": "us-east-1"
}
}
Replace "your-bucket-name" with the actual name of your S3 bucket, and "us-east-1" with the actual region of your bucket.
Step 6: Write Cypress Test
Finally, you can write your Cypress test. Here's an example test that navigates to the login page, enters valid credentials, and submits the form. It then captures a baseline screenshot and a success screenshot, and compares them to the baseline using matchImageSnapshot():
describe('Login page visual regression test', () => {
it('should match the baseline screenshot', () => {
cy.visit('/login');
cy.get('#username').type('myusername');
cy.get('#password').type('mypassword');
cy.get('#submit').click();
cy.get('body').matchImageSnapshot('login-page-success');
});
});
Step 7: Run Cypress Test and Upload Baseline Screenshots
Finally, you can run your Cypress test and upload the baseline screenshots to your S3 bucket. To do this, run the following command:
npx cypress run --env mode=baseline
This will run your tests in "baseline" mode, which captures the baseline screenshots and uploads them to S3.
Step 8: Run Cypress Test and Compare Screenshots
Now that you have the baseline screenshots in S3, you can run your test in "test" mode to compare the current screenshots to the baseline:
npx cypress run --env mode=test
This will run your tests in "test" mode, which captures the current screenshots and compares them to the baseline in S3.
If the screenshots don't match, the test will fail with a visual diff showing the differences between the two images. You can then investigate the differences and decide whether to accept the new screenshot as the new baseline, or whether to fix the issue in your code.
And that's it! With these steps, you can use cypress-visual-regression with AWS Visual Testing to perform visual regression testing in Cypress.
Read Next :
- Setting up a test environment for Cypress with AWS services
- Integrating Cypress with AWS CodePipeline for continuous testing and deployment
- Using AWS Lambda functions in Cypress tests for serverless testing
- Managing test data with AWS DynamoDB in Cypress tests
- Creating visual regression tests with AWS Visual Testing in Cypress
- Load testing AWS applications with Cypress and AWS Load Testing services
- Monitoring AWS CloudWatch metrics with Cypress tests for improved observability
- Debugging Cypress tests in AWS environments using AWS X-Ray.
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer