Improving Observability: Monitoring AWS CloudWatch Metrics with Cypress Tests
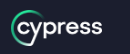
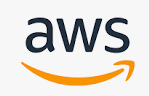
In today's world of cloud-based applications, monitoring and observability are crucial for ensuring smooth operation and quick resolution of issues. One tool that can help with this is AWS CloudWatch, which allows you to collect and monitor metrics, logs, and events from various AWS resources.
However, manually monitoring these metrics can be time-consuming and error-prone. That's where Cypress tests come in.
Cypress is a popular end-to-end testing framework for web applications, but it can also be used for monitoring purposes. By using Cypress tests to collect and report AWS CloudWatch metrics, you can gain insight into the performance and behavior of your cloud-based applications. Here's how to do it:
Set up AWS CloudWatch
First, you'll need to set up AWS CloudWatch to collect the metrics you want to monitor.
This involves creating a CloudWatch dashboard, setting up alarms, and configuring the AWS resources you want to monitor. You can find detailed instructions on how to do this in the AWS documentation.
Write Cypress tests
Next, you'll need to write Cypress tests to collect the metrics you want to monitor.
For example, you might write a test that simulates a user signing up for your application and then checks the CloudWatch metrics for the number of signups. You can use the AWS SDK for JavaScript to programmatically collect CloudWatch metrics from your Cypress tests.
Report CloudWatch metrics in Cypress
Once you've collected the CloudWatch metrics in your Cypress tests, you can report them using Cypress custom commands or plugins.
This will allow you to view the metrics alongside your other Cypress test results, giving you a holistic view of your application's performance.
Set up alerts and notifications
Finally, you'll want to set up alerts and notifications based on the CloudWatch metrics you're monitoring. For example, you might want to receive an email notification if the number of signups drops below a certain threshold. You can configure this in the CloudWatch dashboard.
By using Cypress tests to monitor AWS CloudWatch metrics, you can improve the observability of your cloud-based applications and catch issues before they become major problems.
With the right setup and configuration, you can gain valuable insights into your application's performance and behavior, allowing you to make informed decisions and deliver a better user experience.
Here is the example that demonstrates how you can use the AWS SDK for JavaScript to collect CloudWatch metrics from your Cypress tests:
const AWS = require('aws-sdk');
const cw = new AWS.CloudWatch({region: 'us-west-2'});
describe('My App', () => {
it('should have a successful login', () => {
cy.visit('/login');
cy.get('input[name="username"]').type('user123');
cy.get('input[name="password"]').type('pass123');
cy.get('button[type="submit"]').click();
// Collect CloudWatch metric for successful login
cw.putMetricData({
MetricData: [
{
MetricName: 'SuccessfulLogins',
Dimensions: [
{
Name: 'App',
Value: 'MyApp'
},
],
Timestamp: new Date(),
Value: 1,
Unit: 'Count'
},
],
Namespace: 'MyAppMetrics'
}, function(err, data) {
if (err) console.log(err, err.stack);
else console.log(data);
});
});
});
In this example, we're using the AWS.CloudWatch class from the AWS SDK for JavaScript to put a metric for successful logins into CloudWatch.
The putMetricData method takes a MetricData parameter that includes the name and value of the metric, as well as any dimensions and a timestamp.
The Namespace parameter specifies the namespace for the metric. In this case, we're using the MyAppMetrics namespace for our metrics.
Read Next :
- Setting up a test environment for Cypress with AWS services
- Integrating Cypress with AWS CodePipeline for continuous testing and deployment
- Using AWS Lambda functions in Cypress tests for serverless testing
- Managing test data with AWS DynamoDB in Cypress tests
- Creating visual regression tests with AWS Visual Testing in Cypress
- Load testing AWS applications with Cypress and AWS Load Testing services
- Monitoring AWS CloudWatch metrics with Cypress tests for improved observability
- Debugging Cypress tests in AWS environments using AWS X-Ray.
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer