Java Collection Framework Coding Problems with Solutions
The Java Collection Framework provides a set of interfaces and classes to store and manipulate groups of data as a single unit. In this article, we will discuss some common coding problems related to the Java Collection Framework and provide solutions to these problems.
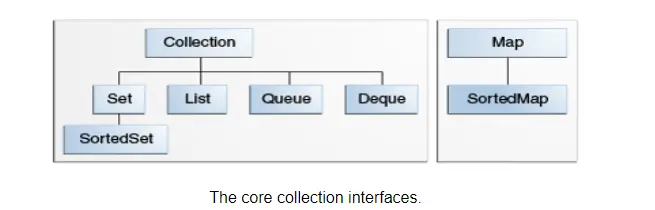
Problem 1: Remove Duplicates from a List
Given a list of integers, write a Java program to remove all duplicate elements from the list.
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class RemoveDuplicates {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(2);
list.add(3);
list.add(4);
list.add(4);
list.add(5);
Set<Integer> set = new HashSet<>(list);
list.clear();
list.addAll(set);
System.out.println("List after removing duplicates: " + list);
}
}
Solution: In the above code, we use a HashSet
to remove duplicates because sets do not allow duplicate elements. We then clear the original list and add all elements from the set back to the list.
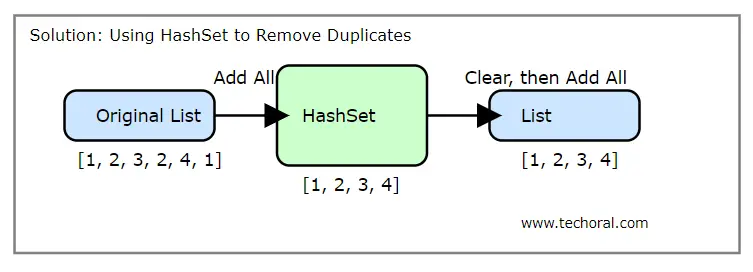
Problem 2: Find the Frequency of Elements in a List
Write a Java program to find the frequency of each element in a given list.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class FrequencyCounter {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("apple");
list.add("banana");
list.add("apple");
list.add("orange");
list.add("banana");
list.add("apple");
Map<String, Integer> frequencyMap = new HashMap<>();
for (String item : list) {
frequencyMap.put(item, frequencyMap.getOrDefault(item, 0) + 1);
}
System.out.println("Frequency of elements: " + frequencyMap);
}
}
Solution: This code uses a HashMap
to count the frequency of each element in the list. The getOrDefault
method is used to handle the case where an element is not already present in the map.
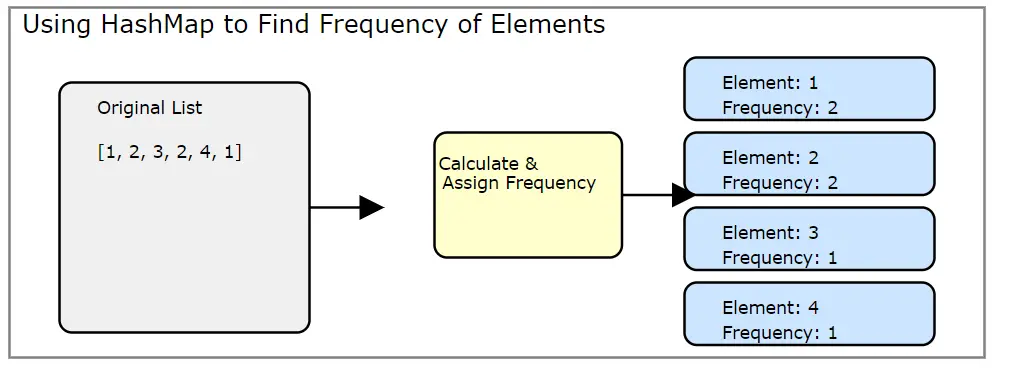
Problem 3: Sort a List of Custom Objects
Given a list of custom objects (e.g., a list of employees with names and ages), write a Java program to sort the list by age.
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
class Employee {
String name;
int age;
Employee(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return name + " (" + age + ")";
}
}
public class SortEmployees {
public static void main(String[] args) {
List<Employee> employees = new ArrayList<>();
employees.add(new Employee("Alice", 30));
employees.add(new Employee("Bob", 25));
employees.add(new Employee("Charlie", 35));
Collections.sort(employees, new Comparator<Employee>() {
@Override
public int compare(Employee e1, Employee e2) {
return Integer.compare(e1.age, e2.age);
}
});
System.out.println("Sorted list of employees: " + employees);
}
}
Solution: In this example, we define a custom Employee
class with name
and age
attributes. We then use the Collections.sort
method along with a custom Comparator
to sort the list of employees by age.
Problem 4: Sort a List of Strings by Length
Given a list of strings, write a Java program to sort the list by the length of the strings in ascending order.
1. Using Compartor
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class SortByLength {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("apple");
list.add("banana");
list.add("kiwi");
list.add("pear");
list.add("pineapple");
Collections.sort(list, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return Integer.compare(s1.length(), s2.length());
}
});
System.out.println("List sorted by length: " + list);
}
}
Solution: In the above code, we use the Collections.sort
method with a custom Comparator
to sort the list of strings by their lengths.
2. Using Comparable
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
class StringWithLength implements Comparable {
private String value;
public StringWithLength(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public int compareTo(StringWithLength other) {
return Integer.compare(this.value.length(), other.value.length());
}
@Override
public String toString() {
return value;
}
}
public class SortStringsByLength {
public static void main(String[] args) {
List list = new ArrayList<>();
list.add(new StringWithLength("apple"));
list.add(new StringWithLength("banana"));
list.add(new StringWithLength("kiwi"));
list.add(new StringWithLength("pear"));
list.add(new StringWithLength("pineapple"));
Collections.sort(list);
System.out.println("List sorted by length: " + list);
}
}
Solution: In this solution, we use the compareTo method in a custom class to define how the strings should be compared based on their lengths. This demonstrates how to implement sorting using compareTo for a specific comparison logic..
Key Differences
Context of Use:
compare
is used within aComparator
for custom sorting logic.compareTo
is used within a class implementingComparable
to define natural ordering.
Method Signature:
compare
takes two objects and compares them.compareTo
compares the current object with another object.
Implementation:
compare
is typically used when you need to define multiple different ways to compare objects.compareTo
is used to define a single natural order for objects.
Using the Comparable interface, you define a single natural ordering for the objects of your class. This is useful when there's one primary way to compare the objects. For example, let's say the natural ordering of Person objects is based on age.
By solving these common coding problems, you can better understand and utilize the Java Collection Framework in your applications. For more advanced topics and examples, explore the Java documentation and other resources available online.
Read Next
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java
Archives
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java