Reserved vs. Contextual Keywords in Java: Understanding the Differences
Java programming language consists of keywords that are reserved for specific functionalities. These keywords are classified into two main categories: reserved keywords and contextual keywords. Understanding the distinction between these two types of keywords is crucial for writing efficient and error-free Java code. In this article, we will delve into the definitions, differences, and examples of reserved and contextual keywords in Java.
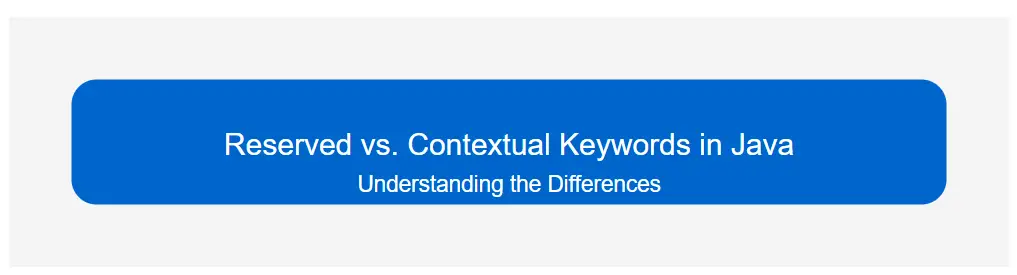
1: What are Reserved Keywords in Java?
Reserved keywords in Java are predefined words that have specific meanings and purposes within the language. These keywords are integral to the syntax and cannot be used for any other purpose such as naming variables, methods, or classes. Here is a list of reserved keywords in Java:
abstract assert boolean break byte
case catch char class const*
continue default do double else
enum extends final finally float
for goto* if implements import
instanceof int interface long native
new null package private protected
public return short static strictfp
super switch synchronized this throw
throws transient try void volatile
while
Note: const
and goto
are reserved but not used in the language.
const
: Theconst
keyword was reserved with the intention of possibly introducing it in future versions of Java, similar to its use in C/C++. However, Java introduced thefinal
keyword to declare constants instead, makingconst
unnecessary.goto
: Thegoto
keyword is reserved to prevent the creation of potentially harmful and hard-to-maintain code. In many programming languages,goto
is used to jump to different parts of the code, which can lead to "spaghetti code" – code that is tangled and difficult to follow. Java avoids this by not implementinggoto
and encouraging structured programming practices.
2: Understanding Contextual Keywords
Contextual keywords are words that have a special meaning only in specific contexts within the Java language. Unlike reserved keywords, they can be used as identifiers (variable names, method names, etc.) outside of their special context. Here are a couple of examples of contextual keywords in Java:
var
Keyword
The var
keyword, introduced in Java 10, is used for local variable type inference. It allows the compiler to infer the type of the variable based on the assigned value. Here is an example:
var list = new ArrayList<String>(); // `var` here is used for type inference
However, var
can also be used as an identifier:
int var = 10;
System.out.println(var); // Prints: 10
yield
Keyword
The yield
keyword, introduced in Java 13, is used in switch
expressions to return a value from a case block. Here is an example:
public class Main {
public static void main(String[] args) {
String day = "MONDAY";
String result = switch (day) {
case "MONDAY", "FRIDAY", "SUNDAY" -> "Weekend";
case "TUESDAY" -> "Working Day";
default -> {
yield "Midweek"; // `yield` is used in switch expression
}
};
System.out.println(result); // This will correctly print: "Weekend"
}
}
Checkout ContextualKeywords.java on GitHub
3: Key Differences Between Reserved and Contextual Keywords
- Usage Restriction: Reserved keywords cannot be used as identifiers, while contextual keywords can be used as identifiers outside their special context.
- Context Sensitivity: Reserved keywords have a fixed meaning regardless of context, whereas contextual keywords have special meanings only in specific contexts.
4: Conclusion
Understanding the differences between reserved and contextual keywords in Java is essential for writing clear and effective code. Reserved keywords are strictly reserved for their predefined purposes, whereas contextual keywords offer more flexibility and can serve different roles depending on the context. By recognizing and appropriately using these keywords, Java developers can avoid common pitfalls and enhance their coding efficiency.
5: Relevant Resources for Further Reading
- Java Language Keywords - Official Java documentation on language keywords.
- Java Collections - Comprehensive guide on Java Collections.
- Java Decompiler JD - An article on using Java Decompiler JD.
- Installing Zulu OpenJDK - Step-by-step guide on installing Zulu OpenJDK.
Read Next
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java
Archives
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java