Java 8 Features
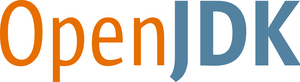
Whats new in Java 8 ?
With Java 8, developers have access to a number of new language features. These include lambda expressions, method references, default methods, and more. You can use either Oracle JDK with valid license or OpenJDK ( at free of cost ).
If you would like to go with OpenJDK, Please refer below section to Download and install OpenJDK
Why OpenJDK ?
OpenJDK 8 is the open source implementation of the Java Platform Standard Edition and is free to use with General Public License Version 2 ; GPLv2. OpenJDK 8 is the reference implementation of the Java SE 8 Specification. For more details you can refer the official Java SE 8 specification from Java Community Process here - JSR366.
Open JDK source code can be downloaded from Mercurial repository
OpenJDK Java 8 Download and Installation on Windows
How to install openjdk 8 on windows ?. If you are already familiar with Java Development Kit (JKD) installation, please Go to https://jdk.java.net/ and get your copy of OpenJDK. If you are new to Java, here is quick guide to help you get started OpenJDK Java 8 Download and Installation On Windows
Java 8 features
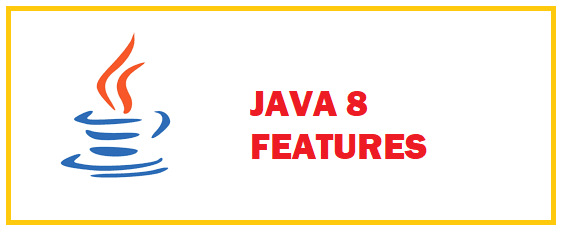
Java 8 introduces many new features that will improve the way programmers write code. Here are the main features.
1# Lambda Expressions
Lambda expressions allow you to define functions inline. They make it easier to express complex logic without having to use multiple lines of code. You can also use them as parameters to other functions.
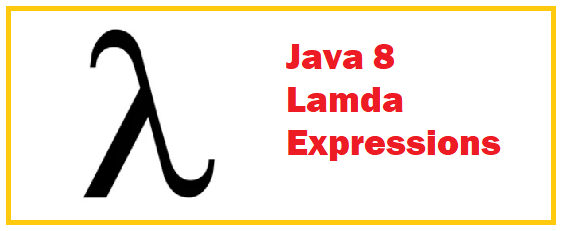
Lambda expressions are a powerful tool in programming languages that allow programmers to create functions without having to write code for each function separately. They also provide an easy way to define anonymous classes.
What Are Lambda Expressions?
Lambda expressions are used to create anonymous classes. Anonymous classes are classes whose instances cannot be accessed outside the class definition. This means that they cannot be referenced by name anywhere else in the program.
When Should You Use Lambda Expressions? Why?
Lambda expressions are very powerful tools that allow programmers to write code more concisely. They also make programming easier because they eliminate the need to use variables.
How Do Lambda Expressions Work?
A lambda expression is an anonymous function. It has no name and does not return anything. Instead, it takes input parameters and returns a result based on those inputs.
2# Method References
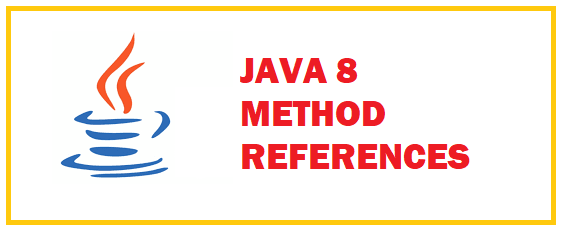
Method references let you refer to a function by name instead of using its full signature. This makes it easy to call a function with only one parameter. It also lets you avoid writing out the entire signature when calling a function.
A method reference is an alternative way to call a method. It looks like a normal variable name, except for the "::" prefix. For example, instead of writing new MyClass().myMethod(), you could write new MyClass()::myMethod().
Method References Examples :
1. The String::length() Method Reference
The abs() method takes one argument, x, and returns the absolute value of x. If x is positive, then abs(x) will return the same as x. If x is negative, then abs(x), will return -x.
2. The Math::sqrt() Method Reference
The sqrt() method takes no arguments and returns the square root of its first argument. It's similar to Math::pow(), except that it only works with numbers.
3. The Math::pow() Method Reference
pow(x, y) = x^y
4. The Math::sin() Method Reference
sin(x) = x * Math::cos(x) / 2
3# Default Methods
A default method is a special kind of method that has no parameters. You can use them to add functionality to an existing class without having to write any code. They can make your code easier to read because you don’t need to specify every possible argument.
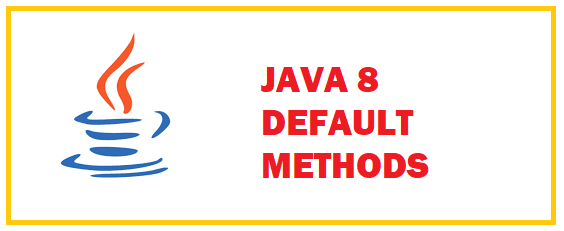
If you're familiar with interfaces then you probably know what a default method is. A default method is one that's defined by the interface itself. It's not something that you define yourself. So, let's say you have an interface called MyInterface. And inside that interface there's a method named myMethod(). Now, if you were to implement that interface, you would do so by implementing the method myMethod() as well. However, if you didn't implement that method, then the compiler would complain because it wouldn't know how to call it. That's where default methods come into play.
4# Streams
In addition to these new language features, Java 8 also includes some changes to how streams work. Java Streams allows us to perform complex data transformations without having to write code.
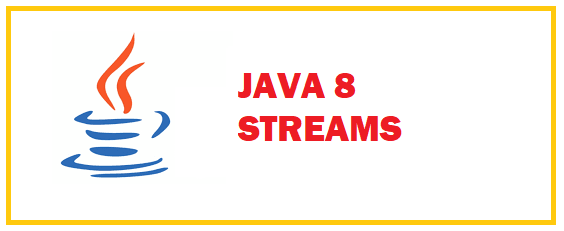
Streams are a powerful feature of Java 8. They allow us to transform collections of data into other collections of data. This allows us to do things like filtering, mapping, grouping, etc. We will start by creating our first stream.
Creating a Stream from an Iterable
To create a stream we need to use the java.util.stream package. It contains classes that provide methods to work with streams. One of those classes is the java.util.Stream class. It has two main methods; map() and filter().
Filtering a Stream
filter() method takes an instance of a Predicate as its parameter. A predicate is just a function that returns true or false. If the predicate returns true then the element will pass through the filter. Otherwise, it won't.
Combining Streams
To combine two streams together, we use the merge() method. It accepts two streams as parameters and merges them into one stream. We can also chain multiple filters together by chaining methods after each other.
5# Optional Chaining
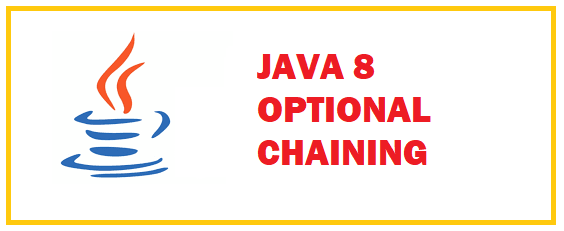
One of the biggest changes to Java 8 is the introduction of optional chaining. Optional chaining allows you to use an expression instead of null when checking whether something exists. Java 8 introduced a new feature called "Optional Chaining" which allows us to chain method calls without having to worry about null values.
6# Nashorn JavaScript Engine
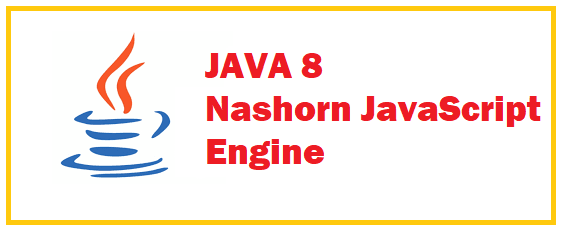
Java 8 has introduced a new JavaScript engine called Nashorn. Nashorn is an open source implementation of the ECMAScript 5th edition specification. It is used by Oracle JRockit as the default JavaScript engine on their JDKs. Nashorn is a lightweight, fast, and efficient JavaScript engine. It is also compatible with other languages such as Groovy, Clojure, Scala, and Ruby. Nashorn is a full-featured JavaScript engine that supports most of the language features. It includes support for object literals, closures, dynamic typing, type inference, and more.
Nashorn is available under the Apache License version 2.0. The Nashorn project was started in 2009 by James Gosling, who worked at Sun Microsystems until 2010. Nashorn is written entirely in Java. And Nashorn is part of the Java SE Platform
- In addition to being a JavaScript engine, Nashorn is also a Java Virtual Machine (JVM) implementation.
- Nashorn provides a set of APIs that allow developers to interact with the JVM.
- Nashorn is designed to run on any platform that supports Java.
- Nashorn is not only a JavaScript engine; it is also a general purpose programming language. and Nashorn is a drop-in replacement for Rhino, the current JavaScript engine in Java.
- Nashorn is a fully compliant implementation of the ECMAscript 5th Edition standard. Nashorn is a complete implementation of the ECMA-262 standard.
- Nashorn is a free software project and Nashorn is developed by Oracle Corporation. Nashorn is released under the Apache license.
- Nashorn is available on GitHub Nashorn Github
- Nashorn is available via Maven. You can add the below dependency in POM.xml file
<dependency>
<groupId>org.openjdk.jmc</groupId>
<artifactId>nashorn </artifactId>
<version>1.8.0_40 </version>
</dependency>
7# Parallel Array Sorting
Introduction
Java Parallel Arrays are the next generation of sorting algorithms. You can now sort your data using parallel arrays!. We can use parallel arrays as a replacement for bubble sort.A parallel array is an array whose elements are stored contiguously in memory. This means that each element occupies one contiguous block of memory. An array with N elements will take up N bytes of memory. If there are M threads running concurrently, then the total space required by the array is (N * M) bytes.
The Problem
Let's say we have an array of integers called intArray. Each integer in the array has a value between 0 and 9. Now let's assume that we need to find out whether any two adjacent numbers in the array sum to 15. To do so, we would loop through the entire array and compare each pair of adjacent values.
A Solution
If we were using sequential arrays, we'd have to perform a linear search through the entire array to find the first number that sums to 15. This would take O(n) time, where n is the length of the array. However, if we use a parallel array, we can reduce the runtime complexity to O(log n).
A parallel array is an array whose elements are stored in different parts of memory. It's similar to a linked list, except instead of storing pointers to other nodes, it stores the actual data.
One of the main benefits of using a parallel array is that it allows us to access any element in O(1) time. This means that we can find the index of any element in constant time.
Complete list of Java 8 features :
Below is the consolidated list of java 8 features
1 Lambda Expressions & Virtual Extension Methodsz
2 Autoconf-Based Build System
3 Lambda-Form Representation for Method Handles
4 Compact Profiles
5 Prepare for Modularization
6 Leverage CPU Instructions for AES Cryptography
7 Nashorn JavaScript Engine
8 Mechanical Checking of Caller-Sensitive Methods
9 Document JDK API Support and Stability
10 Reduce Cache Contention on Specified Fields
11 Remove the Permanent Generation
12 Retire Some Rarely-Used GC Combinations
13 Enhanced Verification Errors
14 Reduce Class Metadata Footprint
15 Small VM
16 Fence Intrinsics core
17 Launch JavaFX Applications core/lang
18 Generalized Target-Type Inference
19 Annotations on Java Types
20 DocTree API
21 Add Javadoc to javax.tools
22 Remove the Annotation-Processing Tool (apt)
23 Access to Parameter Names at Runtime
24 Repeating Annotations
25 Enhance javac to Improve Build Speed
26 DocLint core/libs
27 Parallel Array Sorting
28 Bulk Data Operations for Collections
29 Enhance Core Libraries with Lambda
30 Charset Implementation Improvements
OpenJDK Java 8 Open Issues/bugs
All Known Issues and bugs can be tracked here:
OpenJDK 8 bugs/issues