Java Collection Framework Coding Problems with Solutions #1
The Java Collection Framework provides a set of interfaces and classes to store and manipulate groups of data as a single unit. In this article, we will discuss some common coding problems related to the Java Collection Framework and provide solutions to these problems.
Problem 1: Remove Duplicates from a List
Given a list of integers, write a Java program to remove all duplicate elements from the list.
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class RemoveDuplicates {
public static void main(String[] args) {
List numbers = new ArrayList<>(List.of(1, 2, 2, 3, 4, 4, 5));
Set uniqueNumbers = new HashSet<>(numbers);
numbers.clear();
numbers.addAll(uniqueNumbers);
System.out.println("List after removing duplicates: " + numbers);
}
}
**************************************************************************
OUTPUT
**************************************************************************
list after removing duplicates : [1, 2, 3, 4, 5, 6, 7, 8, 9]
View RemoveDuplicates.java on GitHub
Problem 2: Find Maximum Salary
Given a list of employees, write a Java program to find the employee with the maximum salary greater than 30,000.
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
}
public class MaxSalary {
public static void main(String[] args) {
List employeeList = Arrays.asList(
new Employee("techoral", 45000.0),
new Employee("com", 35000.0),
new Employee("Avi", 55000.0),
new Employee("Kevin", 55000.0),
new Employee("Nash", 25000.0),
new Employee("guru", 15000.0)
);
Optional empMax = employeeList.stream()
.filter(emp -> emp.getSalary() > 30000)
.sorted((a, b) -> {
int maxSal = Double.compare(b.getSalary(), a.getSalary()); // descending order by salary
if (maxSal == 0) {
return a.getName().compareTo(b.getName()); // ascending order by name
}
return maxSal;
})
.findFirst(); // get the first element after sorting
System.out.println(empMax.map(e -> "Empname with Max salary: " + e.getName() + " : " + e.getSalary())
.orElse("No Employee with greater than 30000"));
}
}
**************************************************************************
OUTPUT
**************************************************************************
Empname with Max salary: Avi : 55000.0
Checkout EmpMaxSalary.java on GitHub
Problem 3: Merge Two Sorted Lists
Given two sorted lists, write a Java program to merge them into a single sorted list.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class MergeSortedLists {
public static void main(String[] args) {
List list1 = new ArrayList<>(Arrays.asList(1, 3, 5, 7));
List list2 = new ArrayList<>(Arrays.asList(2, 4, 6, 8));
List mergedList = new ArrayList<>();
int i = 0, j = 0;
while (i < list1.size() && j < list2.size()) {
if (list1.get(i) < list2.get(j)) {
mergedList.add(list1.get(i));
i++;
} else {
mergedList.add(list2.get(j));
j++;
}
}
while (i < list1.size()) {
mergedList.add(list1.get(i));
i++;
}
while (j < list2.size()) {
mergedList.add(list2.get(j));
j++;
}
System.out.println("Merged Sorted List: " + mergedList);
}
}
**************************************************************************
OUTPUT
**************************************************************************
Merged Sortred List : [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Checkout MergeSortedList.java on GitHub
Merge sorted list using Streams
Problem 4: Rotate a Matrix by 90 Degrees
Given a matrix, write a Java program to rotate the matrix by 90 degrees.
public class RotateMatrix {
public static void main(String[] args) {
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int n = matrix.length;
for (int i = 0; i < n / 2; i++) {
for (int j = i; j < n - i - 1; j++) {
int temp = matrix[i][j];
matrix[i][j] = matrix[n - 1 - j][i];
matrix[n - 1 - j][i] = matrix[n - 1 - i][n - 1 - j];
matrix[n - 1 - i][n - 1 - j] = matrix[j][n - 1 - i];
matrix[j][n - 1 - i] = temp;
}
}
System.out.println("Rotated Matrix by 90 degrees:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
}
}
**************************************************************************
OUTPUT
**************************************************************************
Merged Sortred List : [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Checkout MatrixRotate90.java on GitHub
Problem 5: Detect a Cycle in a Linked List
Given a linked list, write a Java program to detect if there is a cycle in the linked list.
A cycle exists if a node in the list is visited more than once while traversing the list.
To detect a cycle in a linked list, we can use Floyd’s Cycle-Finding Algorithm, also known as the Tortoise and Hare Algorithm. This approach uses two pointers that move at different speeds. If there is a cycle, the fast pointer will eventually meet the slow pointer.
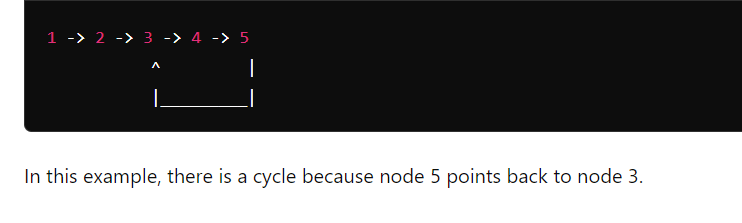
class ListNode {
int val;
ListNode next;
ListNode(int x) {
val = x;
next = null;
}
}
public class LinkedListCycle {
public boolean hasCycle(ListNode head) {
if (head == null || head.next == null) {
return false;
}
ListNode slow = head;
ListNode fast = head.next;
while (slow != fast) {
if (fast == null || fast.next == null) {
return false;
}
slow = slow.next;
fast = fast.next.next;
}
return true;
}
}
Checkout LinkedListCycle.java on GitHub
By solving these common coding problems, you can better understand and utilize the Java Collection Framework in your applications. For more advanced topics and examples, explore the Java documentation and other resources available online.
Read Next
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java
Archives
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java