Java Coding Problems with Solutions #2
Below are some common coding problems in Java with their solutions. These problems are designed to help you improve your coding skills and prepare for interviews.
Problem 1: Check if a String is a Palindrome
Write a method to check if a given string is a palindrome.
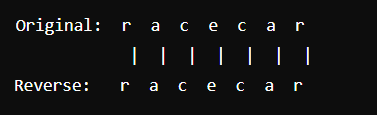
public class PalindromeCheck {
public static boolean isPalindrome(String s) {
int left = 0, right = s.length() - 1;
while (left < right) {
if (s.charAt(left) != s.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
public static void main(String[] args) {
System.out.println(isPalindrome("racecar")); // true
System.out.println(isPalindrome("hello")); // false
}
}
Checkout Palindrome.java on GitHub
Checkout PalidromeStreams.java on GitHub
Problem 2: Find Two Numbers that Add Up to a Specific Target
Given an array of integers, write a function to find the two numbers that add up to a specific target.
import java.util.HashMap;
import java.util.Map;
public class TwoSum {
public static int[] findTwoSum(int[] nums, int target) {
Map map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
public static void main(String[] args) {
int[] nums = {2, 7, 11, 15};
int target = 9;
int[] result = findTwoSum(nums, target);
System.out.println("Indices: " + result[0] + ", " + result[1]); // Indices: 0, 1
}
}
View on GitHub
Problem 3: Implement a Simple LRU Cache
Implement a simple Least Recently Used (LRU) cache in Java.
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache extends LinkedHashMap {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry eldest) {
return size() > capacity;
}
public static void main(String[] args) {
LRUCache cache = new LRUCache<>(3);
cache.put(1, "One");
cache.put(2, "Two");
cache.put(3, "Three");
System.out.println(cache);
cache.get(1);
cache.put(4, "Four");
System.out.println(cache);
}
}
View on GitHub
Problem 4: Perform Binary Search on a Sorted Array
Write a function to perform a binary search on a sorted array.
public class BinarySearch {
public static int binarySearch(int[] nums, int target) {
int left = 0, right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) {
return mid;
}
if (nums[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
public static void main(String[] args) {
int[] nums = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int target = 5;
System.out.println("Index of target: " + binarySearch(nums, target)); // Index of target: 4
}
}
View on GitHub
Problem 5: In-Order Traversal of a Binary Tree
Given a binary tree, write a function to perform in-order traversal.
import java.util.ArrayList;
import java.util.List;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public class InOrderTraversal {
public static List inOrderTraversal(TreeNode root) {
List result = new ArrayList<>();
inOrderHelper(root, result);
return result;
}
private static void inOrderHelper(TreeNode node, List result) {
if (node != null) {
inOrderHelper(node.left, result);
result.add(node.val);
inOrderHelper(node.right, result);
}
}
public static void main(String[] args) {
TreeNode root = new TreeNode(1);
root.right = new TreeNode(2);
root.right.left = new TreeNode(3);
List result = inOrderTraversal(root);
System.out.println("In-order Traversal: " + result); // In-order Traversal: [1, 3, 2]
}
}
View on GitHub
By solving these common coding problems, you can better understand and utilize the Java Collection Framework in your applications. For more advanced topics and examples, explore the Java documentation and other resources available online.
Read Next
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java
Archives
- Java is not recognized
- IntellijIdea Installation guide
- Java Reserved vs Contextual Keywords
- Java ERROR code 1603
- Procyon Java Decompiler
- Resultset has now rows. Quick Fix!
- Java Update on Mac
- Java Update check fails
- Java wont install on windows 10
- Java installation error code 80
- Windows does not recognize java
- Access Adobe programs Java
- Failed to install java update
- System breaks jdk8 javac command
- Java Uninstall Stops at Progress Bar
- Could not find java dll
- Eclipse Error code 13
- ERROR - Postgresql Jdbc Driver
- Java Garbage Collection
- Input mismatch exception in java
- Error Connecting Postgresql
- Multithreading in Java