How to Pass Environment Variables to Cypress Tests
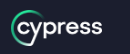
Cypress is a popular end-to-end testing framework that helps developers write and run tests for web applications. One of the key features of Cypress is its ability to use environment variables, which allow you to pass dynamic values to your tests. In this article, we will discuss how to set and use environment variables in Cypress tests using code snippets.
We assume that cypress is already installed in your system. If not, please refer Cypress Installation / setup on Visual Studio
Setting Environment Variables in Cypress
There are many ways to set environment variables in Cypress:
#1. Using the Cypress.env() method: This method allows you to set environment variables directly in your test code.
// Set environment variable in Cypress
Cypress.env('myVar', 'myValue');
#2. Using the Cypress CLI: This method allows you to set environment variables globally for all tests.
// Set environment variable using CLI
cypress run --env myVar=myValue
#3. Using .env file ( dotenv package ) as shown below.
a) Install the dotenv package by running the following command in your project directory:
npm install dotenv --save-dev
b) Create a .env file in the root directory of your project and define your variables
with the KEY=VALUE syntax.
For example:
MY_VAR=hello techoral
ANOTHER_VAR=1234
#4. We can also add variables in the setupNodeEvents as shown below
e2e: {
supportFile: false,
setupNodeEvents (on, config) {
config.env = config.env || {}
config.env.FOO = process.env.FOO
config.env.BAR = process.env.BAR
config.env.username = process.env.USER_NAME
console.log('extended config.env with process.env.{FOO, BAR, USER_NAME}')
return config
},
Using Environment Variables in Cypress Tests
Once you have set your environment variables, you can use them in your tests using the Cypress.env() method. Here are some examples:
// Get environment variable value
const myVar = Cypress.env('myVar');
// Use environment variable in test
cy.get('#myInput').type(myVar);
You can also use environment variables in your Cypress configuration file (cypress.json) using the following syntax:
{
"baseUrl": "https://www.techoral.com",
"env": {
"myVar": "myValue"
}
}
This will set the environment variable myVar to the value myValue and make it available to all tests.
Similarly, we can read env variables from .env file as shown below
In your Cypress test file, add require dotenv package at the top of the file:
require('dotenv').config()
Access your variables by using process.env.VAR_NAME syntax.
For example:
describe('Techoral Test Suite', () => {
it('should read variables from .env file', () => {
const myVar = process.env.MY_VAR
const anotherVar = process.env.ANOTHER_VAR
cy.log(myVar)
cy.log(anotherVar)
})
})
Conclusion
In this article, we discussed how to set and use environment variables in Cypress tests using code snippets. By leveraging environment variables, you can increase the efficiency and effectiveness of your Cypress test suite. Whether you are a beginner or an experienced Cypress user, this guide will help you take your testing to the next level.
Read Next :
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer
Cypress Tutorials
Cypress Archives
- Cypress Installation Errors
- How to Install Cypress
- Cypress Uncaught Exception Handling
- Cypress Automation Examples
- Cypress Automation Tool Interview Questions
- Cypress File Upload Examples
- Error 509 Bandwidth Exceeded
- Cypress Commands
- Cypress Custom Commands
- Handling Cypress Tokens & LocalStorage
- Handling Cypress Multitabs
- Cypress Parallelization
- Cypress waits
- Cypress Still Waiting Error
- Cypress Test Run Errors
- Cypress vs Selenium vs Puppeteer
- Cypress vs Selenium
- Cypress vs Puppeteer