Java Performance Optimization: Advanced Techniques for Senior Developers (2025)
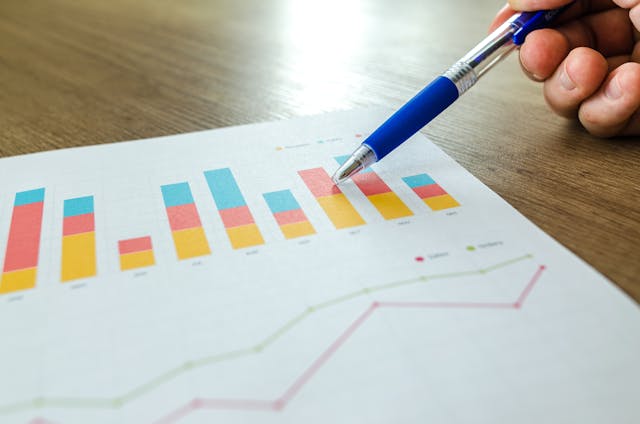
Performance optimization in Java applications is a critical skill for senior developers. This comprehensive guide covers advanced techniques, tools, and best practices for optimizing Java applications at various levels.
Pro Tip: Performance optimization should be approached systematically, starting with profiling to identify bottlenecks before making changes.
Table of Contents
JVM Tuning Fundamentals
Note: JVM tuning is not a one-size-fits-all solution. It requires understanding your application's specific needs and workload patterns.
Key JVM Parameters
Parameter | Description | Example Value |
---|---|---|
-Xms | Initial heap size | -Xms2g |
-Xmx | Maximum heap size | -Xmx4g |
-XX:MetaspaceSize | Initial metaspace size | -XX:MetaspaceSize=256m |
-XX:MaxMetaspaceSize | Maximum metaspace size | -XX:MaxMetaspaceSize=512m |
JVM Tuning Example
// Example JVM tuning configuration
-XX:+UseG1GC
-XX:MaxGCPauseMillis=200
-XX:+UseStringDeduplication
-XX:+UseCompressedOops
-XX:+UseFastUnorderedTimeStamps
Memory Management Optimization
Pro Tip: Memory leaks in Java are often caused by improper resource management or circular references.
Memory Leak Detection
// Example of potential memory leak
public class ResourceManager {
private static final Map resources = new HashMap<>();
public void addResource(String key, Resource resource) {
resources.put(key, resource);
}
// Missing cleanup method
}
// Correct implementation
public class ResourceManager {
private static final Map resources = new HashMap<>();
public void addResource(String key, Resource resource) {
resources.put(key, resource);
}
public void removeResource(String key) {
Resource resource = resources.remove(key);
if (resource != null) {
resource.close();
}
}
}
Garbage Collection Optimization
Note: Different garbage collectors are optimized for different scenarios. Choose based on your application's needs.
Garbage Collector Selection
Garbage Collector | Best For | Configuration |
---|---|---|
G1GC | Large heap applications | -XX:+UseG1GC |
ZGC | Low latency applications | -XX:+UseZGC |
Shenandoah | Large heap, low pause time | -XX:+UseShenandoahGC |
Code-Level Optimizations
String Optimization
// Unoptimized
String result = "";
for (int i = 0; i < 1000; i++) {
result += "text";
}
// Optimized
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append("text");
}
Collection Optimization
// Unoptimized
List list = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
list.add("item");
}
// Optimized
List list = new ArrayList<>(1000);
for (int i = 0; i < 1000; i++) {
list.add("item");
}
Performance Monitoring
Pro Tip: Regular monitoring and profiling are essential for maintaining optimal performance.
JVM Monitoring Tools
- JConsole
- VisualVM
- Java Flight Recorder
- JProfiler
For details refer to Java JVM Tuning
Performance Analysis Tools
Tool | Purpose | Key Features |
---|---|---|
JProfiler | Profiling | CPU, Memory, Thread profiling |
YourKit | Profiling | Memory leak detection |
JFR | Recording | Low overhead event recording |
For details Refer to Java Performance Analysis Tools
Best Practices and Patterns
Note: Performance optimization should be balanced with code maintainability and readability.
Key Best Practices
- Use appropriate data structures
- Implement proper resource cleanup
- Optimize database access
- Use caching effectively
- Implement proper exception handling
- Use concurrent collections when appropriate
Conclusion
Performance optimization in Java requires a comprehensive approach, combining JVM tuning, memory management, garbage collection optimization, and code-level improvements. Regular monitoring and profiling are essential for maintaining optimal performance.