Java Performance Analysis Tools: YourKit and JFR Guide (2025)
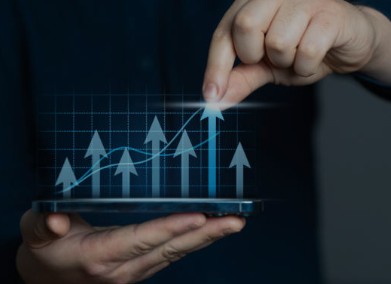
Performance analysis is crucial for optimizing Java applications. This guide explores powerful tools like YourKit and Java Flight Recorder (JFR) for comprehensive performance analysis.
Pro Tip: Regular performance analysis helps identify bottlenecks and optimize application performance.
Table of Contents
YourKit Profiler
Note: YourKit provides comprehensive profiling capabilities with minimal overhead.
Installation
1. Download YourKit:
# Download from https://www.yourkit.com/java/profiler/download/
2. Install the agent:
# For Windows
java -agentpath:C:\Program Files\YourKit\YourKit-JavaProfiler-2023.3\bin\win64\yjpagent.dll YourApplication
# For Linux
java -agentpath:/path/to/yourkit/bin/linux-x64/libyjpagent.so YourApplication
# For macOS
java -agentpath:/path/to/yourkit/bin/mac/libyjpagent.dylib YourApplication
Features
- CPU Profiling
- Memory Profiling
- Thread Profiling
- Deadlock Detection
- Method Call Tracking
- Memory Leak Detection
- Hot Spots Analysis
- Remote Profiling
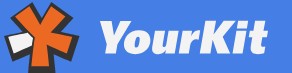
Java Flight Recorder
Pro Tip: JFR provides detailed event-based recording with minimal performance impact.
Installation
JFR is included with the JDK. Enable it with:
# Enable JFR
java -XX:StartFlightRecording=duration=60s,filename=recording.jfr YourApplication
# Configure JFR settings
java -XX:StartFlightRecording=duration=60s,filename=recording.jfr,settings=profile YourApplication
Features
- Event-based Recording
- Method Profiling
- Memory Allocation Tracking
- Thread Analysis
- GC Analysis
- System Events
- Custom Events
- Low Overhead
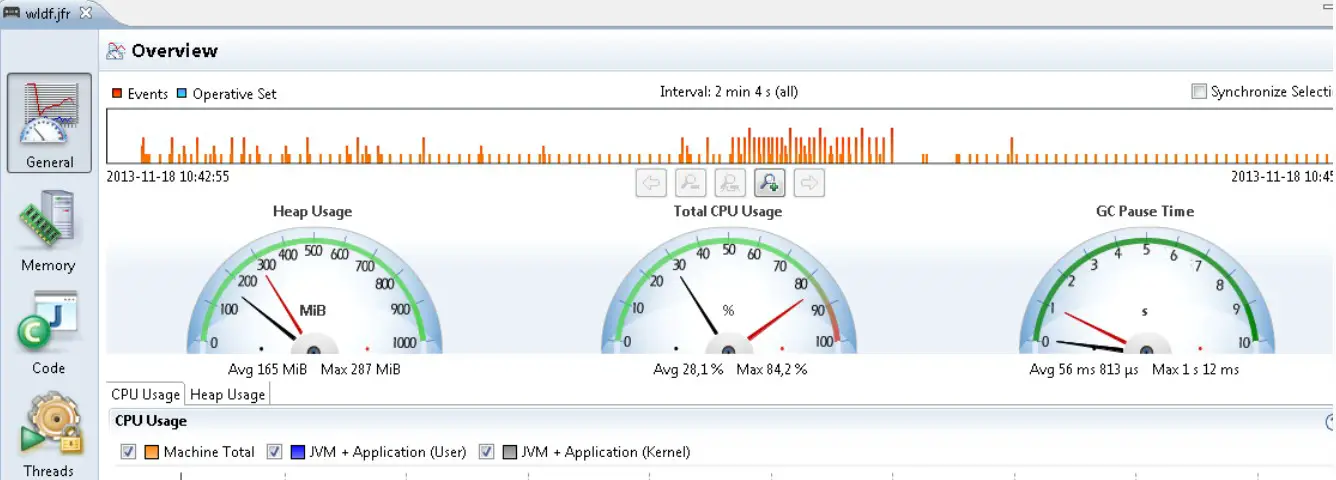
Use Cases
Note: Different performance analysis scenarios require different tools and approaches.
Performance Bottleneck Analysis
// Using YourKit
// 1. Start CPU profiling
// 2. Run the application
// 3. Analyze hot spots
// 4. Optimize slow methods
// Using JFR
// 1. Start recording
jcmd JFR.start duration=60s filename=bottleneck.jfr
// 2. Run the application
// 3. Stop recording
jcmd JFR.stop name=1
// 4. Analyze results
jfr print bottleneck.jfr
Memory Leak Detection
// Using YourKit
// 1. Start memory profiling
// 2. Monitor heap usage
// 3. Analyze object allocation
// 4. Identify memory leaks
// Using JFR
// 1. Configure memory events
java -XX:StartFlightRecording=duration=300s,filename=memory.jfr,settings=profile YourApplication
// 2. Monitor memory events
// 3. Analyze heap usage
// 4. Identify leaks
Thread Analysis
// Using YourKit
// 1. Start thread profiling
// 2. Monitor thread states
// 3. Analyze thread interactions
// 4. Identify deadlocks
// Using JFR
// 1. Configure thread events
java -XX:StartFlightRecording=duration=60s,filename=threads.jfr,settings=profile YourApplication
// 2. Monitor thread events
// 3. Analyze thread behavior
// 4. Identify issues
Best Practices
Pro Tip: Following performance analysis best practices ensures accurate results.
Performance Analysis Best Practices
- Profile in production-like environment
- Use appropriate sampling rates
- Monitor tool overhead
- Analyze complete scenarios
- Document analysis results
- Use multiple tools when needed
- Regular performance testing
- Set up performance baselines
- Monitor system resources
- Use appropriate event settings
- Implement proper logging
- Enable proper security
- Use proper authentication
- Enable proper encryption
- Follow analysis guidelines
Conclusion
Effective performance analysis is essential for optimizing Java applications. By understanding and utilizing tools like YourKit and JFR properly, you can identify and resolve performance issues efficiently.