25 Essential Algorithms Every Developer Should Know
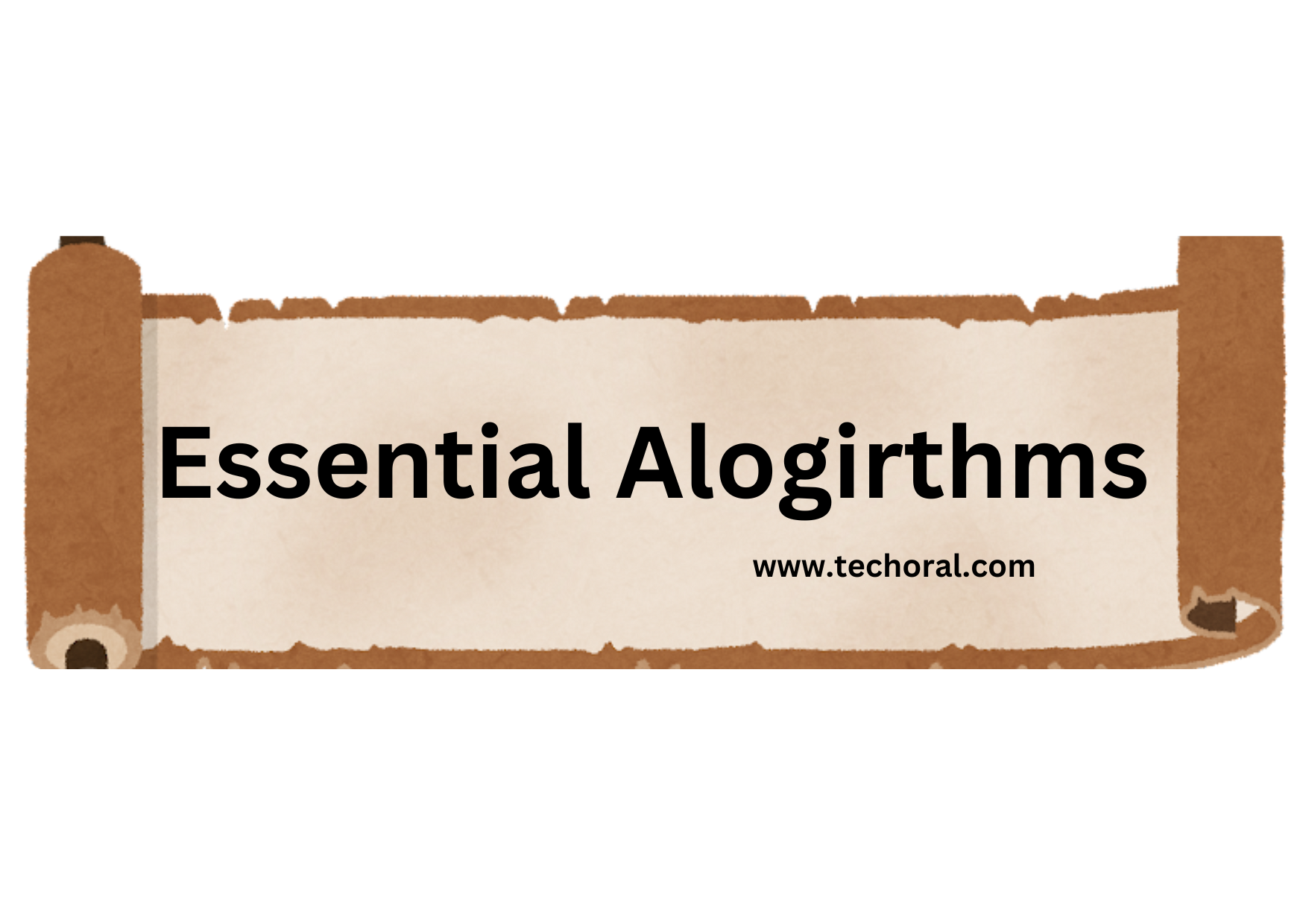
Hey there, Future Coder! ๐
Ready to level up your coding game? These 25 algorithms are your ultimate coding toolkit - from finding the fastest route to your favorite coffee shop to organizing your Spotify playlist!
Here's What We're Diving Into:
๐ Search Squad
Linear, Binary, and friends - they're like your phone's search function, helping you find stuff super fast.
๐ Sorting Crew
From Quick Sort (the speed demon) to Merge Sort (the reliable one) - these guys keep your data organized like a pro.
๐ธ๏ธ Graph Gang
Dijkstra, Kruskal, and crew - imagine Google Maps finding the best route to your next party, that's these algorithms at work!
๐ Array Avengers
Kadane's algorithm finding your best workout streak, Floyd's cycle detection catching those pesky infinite loops.
๐ง Basic Bosses
From compressing your files (Huffman) to organizing your social circles (Union Find) - these are your everyday problem solvers.
Think of these algorithms as your coding superpowers - each one solving real-world problems in clever ways. Let's make coding awesome! ๐
Quick Jump to Algorithms:
- ๐ Search Algorithms:
- ๐ Sorting Algorithms:
- ๐ธ๏ธ Graph Algorithms:
1. Search Algorithms ๐
1.1 Linear Search - The Simple Explorer
[๐ฆ][๐ฆ][๐ฏ][๐ฆ][๐ฆ][๐ฆ] ๐ โ โ Found!
Just like scanning through your Spotify playlist one song at a time, linear search checks each element sequentially until it finds what you're looking for. It's straightforward but can be slow for large datasets.
Java Example:
public class LinearSearch {
public static int findItem(int[] boxes, int target) {
for(int i = 0; i < boxes.length; i++) {
if(boxes[i] == target) {
return i; // Found it!
}
}
return -1; // Not found
}
}
1.2 Binary Search - The Smart Detective
1--2--3--4--5--6--7--8 โ 3--4--5 โ 4 Found!
Think of it as the optimal strategy in a number guessing game. By consistently eliminating half the remaining possibilities, you can find your target much faster than checking every number - but only works on sorted data.
Java Implementation:
public class BinarySearch {
public static int find(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
// Found the target
if (arr[mid] == target) {
return mid;
}
// Target is in the right half
if (arr[mid] < target) {
left = mid + 1;
}
// Target is in the left half
else {
right = mid - 1;
}
}
return -1; // Target not found
}
}
Real-World Example:
// Finding a song in a sorted playlist
public class PlaylistSearch {
public static void main(String[] args) {
String[] playlist = {
"All of Me",
"Believer",
"Dance Monkey",
"Happy",
"Shape of You",
"Uptown Funk"
};
String searchSong = "Happy";
int index = findSong(playlist, searchSong);
System.out.println("Found '" + searchSong + "' at position: " + index);
// Output: Found 'Happy' at position: 3
}
public static int findSong(String[] songs, String target) {
int left = 0;
int right = songs.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
int comparison = songs[mid].compareTo(target);
if (comparison == 0) return mid;
if (comparison < 0) left = mid + 1;
else right = mid - 1;
}
return -1;
}
}
Real-World Applications:
- ๐ต Spotify & Apple Music
When you search for a song, these apps use binary search on sorted song databases to quickly find your music from millions of tracks.
- ๐ฑ Instagram & Facebook
Finding users from billions of sorted usernames when you type in the search bar.
- ๐ Amazon Kindle
Looking up words in the dictionary feature or finding specific pages in an e-book.
- ๐ฎ Gaming
Finding player ranks in leaderboards (like in PUBG or Fortnite) or matching players of similar skill levels.
- ๐ณ Payment Apps (PayPal, Stripe)
Searching through sorted transaction histories or finding specific transaction IDs.
Technical Applications:
- Version control systems (Git) for finding commits
- Database indexing in MySQL and PostgreSQL
- IP routing tables in network devices
- Auto-complete suggestions in IDEs and text editors
- Finding elements in sorted arrays in memory
1.3 Depth First Search - The Deep Explorer
Similar to exploring a multi-level parking garage where you go all the way down one lane before checking the next. It's perfect for thoroughly exploring branching paths, like mapping all possible moves in a chess game.
1 / | \ 2 3 4 / / \ 5 6 7 Visit: 1โ2โ5โ3โ4โ6โ7
Social Network Friend Recommendations Implementation:
public class SocialNetworkDFS {
private Map> friendNetwork;
private Set visited;
public SocialNetworkDFS() {
this.friendNetwork = new HashMap<>();
this.visited = new HashSet<>();
}
// Find all friends within N degrees of connection
public List findFriendsOfFriends(String user, int maxDepth) {
List recommendations = new ArrayList<>();
visited.clear();
dfs(user, maxDepth, 0, recommendations);
recommendations.remove(user); // Remove self from recommendations
return recommendations;
}
private void dfs(String currentUser, int maxDepth, int currentDepth,
List recommendations) {
if (currentDepth > maxDepth || visited.contains(currentUser)) {
return;
}
visited.add(currentUser);
recommendations.add(currentUser);
List friends = friendNetwork.getOrDefault(currentUser, new ArrayList<>());
for (String friend : friends) {
dfs(friend, maxDepth, currentDepth + 1, recommendations);
}
}
// Example usage
public static void main(String[] args) {
SocialNetworkDFS network = new SocialNetworkDFS();
// Add some sample connections
network.friendNetwork.put("Alice", Arrays.asList("Bob", "Charlie"));
network.friendNetwork.put("Bob", Arrays.asList("David", "Eve"));
network.friendNetwork.put("Charlie", Arrays.asList("Frank"));
// Find friends up to 2 degrees away from Alice
List recommendations = network.findFriendsOfFriends("Alice", 2);
System.out.println("Friend recommendations for Alice: " + recommendations);
// Output: [Bob, David, Eve, Charlie, Frank]
}
}
Real-World Applications:
- ๐ฎ Game Development
Solving puzzles, pathfinding in maze games, exploring game worlds
- ๐ฑ Social Networks
Finding friend suggestions, detecting communities, content recommendation
- ๐ File Systems
Directory traversal, searching for files, calculating folder sizes
- ๐ Web Crawling
Indexing web pages, finding broken links, site mapping
1.4 Breadth First Search - The Level Explorer
Imagine sending a mass text to your friends, then they forward it to their friends, creating expanding circles of connections. It's ideal for finding the shortest path or mapping social networks.
1 / | \ 2 3 4 / / \ 5 6 7 Visit: 1โ2โ3โ4โ5โ6โ7
Social Network Friend Distance Calculator:
public class SocialNetworkBFS {
private Map> connections;
public SocialNetworkBFS() {
this.connections = new HashMap<>();
}
// Find friends within specific distance/degrees
public Map findFriendsWithinDistance(String startUser, int maxDistance) {
Map distances = new HashMap<>();
Queue queue = new LinkedList<>();
Set visited = new HashSet<>();
queue.offer(startUser);
distances.put(startUser, 0);
visited.add(startUser);
while (!queue.isEmpty()) {
String currentUser = queue.poll();
int currentDistance = distances.get(currentUser);
if (currentDistance >= maxDistance) {
continue;
}
List friends = connections.getOrDefault(currentUser, new ArrayList<>());
for (String friend : friends) {
if (!visited.contains(friend)) {
queue.offer(friend);
visited.add(friend);
distances.put(friend, currentDistance + 1);
}
}
}
return distances;
}
// Example usage
public static void main(String[] args) {
SocialNetworkBFS network = new SocialNetworkBFS();
// Add sample connections
network.connections.put("Alice", Arrays.asList("Bob", "Charlie"));
network.connections.put("Bob", Arrays.asList("David", "Eve"));
network.connections.put("Charlie", Arrays.asList("Frank", "Grace"));
// Find all friends up to 2 degrees away from Alice
Map friendDistances = network.findFriendsWithinDistance("Alice", 2);
// Print distances to each friend
friendDistances.forEach((friend, distance) ->
System.out.println(friend + " is " + distance + " connections away from Alice"));
// Output:
// Alice is 0 connections away from Alice
// Bob is 1 connections away from Alice
// Charlie is 1 connections away from Alice
// David is 2 connections away from Alice
// Eve is 2 connections away from Alice
// Frank is 2 connections away from Alice
// Grace is 2 connections away from Alice
}
}
Real-World Applications:
- ๐ฎ Gaming AI
Finding shortest paths in strategy games, exploring game levels layer by layer
- ๐ฑ Social Media
LinkedIn's "Connections" feature, Facebook's "People You May Know"
- ๐บ๏ธ GPS Navigation
Finding nearest points of interest, calculating shortest routes
- ๐ Network Protocols
Peer discovery in P2P networks, network broadcast algorithms
2. Sorting Algorithms ๐
2.0 Insertion Sort - The Card Arranger
Like organizing your playlist by dragging each song to its correct position. You take one item at a time and place it where it belongs in the already-sorted portion. Efficient for small lists or nearly sorted data.
Initial: [5โ2,4,1,3] Step 1: [2,5โ4,1,3] Step 2: [2,4,5โ1,3] Step 3: [1,2,4,5โ3] Final: [1,2,3,4,5]
Java Implementation:
public class InsertionSort {
public void sort(int[] arr) {
for (int i = 1; i < arr.length; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
}
Real-World Applications:
- ๐ฎ Online Gaming Leaderboards
Real-time updates of player scores in small multiplayer games, where new scores are usually close to their final position
- ๐ฑ Mobile Contact Lists
Maintaining a sorted contact list when adding new contacts, as they're often added in near-alphabetical order
- ๐ณ Transaction Processing
Maintaining chronologically sorted transaction lists in banking apps, where new transactions are typically added at the end
- ๐
Calendar Applications
Inserting new appointments into an already-sorted calendar, as most new events are added in chronological order
- ๐ต Music Players
Organizing playlists where songs are typically added in a nearly-sorted order based on artist or album
Technical Benefits:
- โจ Adaptive: Performs better on partially sorted data
- ๐ Online: Can sort data as it receives it
- ๐พ In-place: Requires minimal extra memory
- โก Fast for small datasets (< 50 elements)
- ๐ Stable: Maintains relative order of equal elements
2.1 Heap Sort - The Priority Manager
Think of organizing a tournament bracket where the strongest player always rises to the top. The algorithm builds a special tree structure where the largest element "bubbles up" to the root, making it perfect for priority-based systems like job schedulers.
9 / \ 6 8 / \ / \ 4 5 2 7 After Heapify: 9 / \ 7 8 / \ / \ 4 5 2 6
Java Implementation:
public class HeapSort {
public void sort(int[] arr) {
int n = arr.length;
// Build max heap (rearrange array)
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(arr, n, i);
}
// Extract elements from heap one by one
for (int i = n - 1; i >= 0; i--) {
// Move current root (largest element) to the end
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
// Heapify the reduced heap
heapify(arr, i, 0);
}
}
// Heapify function to maintain the heap property
private void heapify(int[] arr, int n, int i) {
int largest = i; // Initialize largest as root
int left = 2 * i + 1; // Left child
int right = 2 * i + 2; // Right child
// If left child is larger than root
if (left < n && arr[left] > arr[largest]) {
largest = left;
}
// If right child is larger than the largest so far
if (right < n && arr[right] > arr[largest]) {
largest = right;
}
// If the largest is not the root, swap and continue heapifying
if (largest != i) {
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
// Recursively heapify the affected sub-tree
heapify(arr, n, largest);
}
}
// Helper method to print the array
public void printArray(int[] arr) {
for (int num : arr) {
System.out.print(num + " ");
}
System.out.println();
}
// Main method to test Heap Sort
public static void main(String[] args) {
int[] arr = {12, 11, 13, 5, 6, 7};
HeapSort heapSort = new HeapSort();
System.out.println("Original array:");
heapSort.printArray(arr);
heapSort.sort(arr);
System.out.println("Sorted array:");
heapSort.printArray(arr);
}
}
Real-World Applications:
- ๐ป Operating Systems
Process scheduling, memory management, and prioritizing system tasks based on urgency
- ๐จ๏ธ Printer Queue Management
Organizing print jobs by priority, ensuring urgent documents are printed first
- ๐ฎ Gaming Engines
Managing event queues, AI decision making, and rendering priority in game engines
- ๐ฑ Mobile Networks
Managing network packets and bandwidth allocation in cellular networks
- ๐ฅ Hospital Systems
Emergency room patient prioritization, medical resource allocation
Technical Benefits:
- โก Efficient for large datasets
- ๐ In-place sorting
- ๐ O(n log n) time complexity
- ๐ฏ Perfect for priority queues
- ๐พ Minimal extra memory usage
2.2 Selection Sort - The Minimum Finder
Similar to how you might organize your playlist by repeatedly finding the next best song and moving it to your "sorted" playlist. Simple but inefficient for large lists, it's great for teaching sorting concepts.
Step 1: [64โ34 25 12 22] โ Find minimum Step 2: [12โ34 25 64 22] โ Swap with first Step 3: [12 22โ25 64 34] โ Repeat
Java Implementation:
public class SelectionSort {
public void sort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
// Find minimum element in unsorted array
int minIdx = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIdx]) {
minIdx = j;
}
}
// Swap found minimum with first element
int temp = arr[minIdx];
arr[minIdx] = arr[i];
arr[i] = temp;
}
}
// Example usage
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22};
SelectionSort sorter = new SelectionSort();
System.out.println("Original array:");
printArray(arr);
sorter.sort(arr);
System.out.println("Sorted array:");
printArray(arr);
}
private static void printArray(int[] arr) {
for (int num : arr) {
System.out.print(num + " ");
}
System.out.println();
}
}
Real-World Applications:
- ๐ Educational Tools
Perfect for teaching sorting concepts due to its simple logic and visualization
- ๐ Small Dataset Management
Organizing classroom grades, small inventory lists, or team rankings in local sports
- ๐พ Memory-Constrained Systems
Embedded systems with limited memory as it requires minimal space
- ๐ฑ Mobile Apps
Sorting small lists in mobile applications where simplicity is preferred over speed
- ๐ฎ Gaming Leaderboards
Managing small local game scores where the dataset rarely exceeds 50 entries
Technical Benefits:
- ๐พ Minimal memory usage (in-place sorting)
- ๐ Simple implementation
- โก Good for small arrays (< 50 elements)
- ๐ Easy to verify correctness
- ๐ Stable performance regardless of input order
2.3 Merge Sort - The Divide and Conquer Hero
Like organizing a deck of cards with friends - split the deck, each person sorts their pile, then merge them back together. It's reliable, stable, and great for handling large datasets, especially when working with linked lists.
[38, 27, 43, 3, 9, 82, 10] / \ [38,27,43,3] [9,82,10] / \ / \ [38,27] [43,3] [9] [82,10]
Real-world use: External sorting in databases, sorting linked lists
2.4 Quick Sort - The Partition Master
Imagine organizing your closet by picking a "pivot" item and arranging everything else as "cheaper than" or "pricier than" that item. Then repeat for each section. It's fast and widely used in real-world applications.
Array: [4,2,8,1,9,3] Pivot: 4 Step 1: [2,1,3|4|8,9] Step 2: [1|2,3|4|8,9]
Real-world use: Default sorting in many programming languages, file systems
2.5 Counting Sort - The Number Organizer
Like sorting exam scores when you know all possible grades are between 0-100. Instead of comparing, you just count how many of each score exists. Super efficient for sorting numbers within a known range!
Array: [4,2,1,4,1,3] Count: [0,2,1,1,2] Final: [1,1,2,3,4,4]
Real-world use: Sorting integers with known range, student grades
3. Graph Algorithms ๐ธ๏ธ
3.0 Kruskal's Algorithm - The Network Builder
Think of building a city's road network with a limited budget. You start with the cheapest roads that connect different areas, gradually adding more until every place is reachable. It's like creating the most cost-effective social network that keeps everyone connected!
Initial Graph: Final MST: A--4--B A B | /| | | | / | โ | | |/ | | | C--2--D C-----D Weight: 2+3+4=9 (Minimum Cost)
Real-world use: Designing computer networks, power grids, water supply networks
3.1 Dijkstra's Algorithm - The Path Finder
Like using a GPS to find the fastest route to a concert. It explores all possible paths, keeping track of the shortest distance to each location. Every time it finds a shorter path, it updates its records - just like how you might update your route when you discover a shortcut!
Startโ(2)โBโ(3)โC โ(4)โDโ(1)โEnd
Real-world use: Google Maps navigation, network routing protocols
Java Implementation:
public class Dijkstra {
public int[] shortestPath(int[][] graph, int start) {
int n = graph.length;
int[] distances = new int[n];
boolean[] visited = new boolean[n];
Arrays.fill(distances, Integer.MAX_VALUE);
distances[start] = 0;
for(int i = 0; i < n-1; i++) {
int minVertex = findMinDistance(distances, visited);
visited[minVertex] = true;
for(int j = 0; j < n; j++) {
if(!visited[j] && graph[minVertex][j] != 0 &&
distances[minVertex] != Integer.MAX_VALUE &&
distances[minVertex] + graph[minVertex][j] < distances[j]) {
distances[j] = distances[minVertex] + graph[minVertex][j];
}
}
}
return distances;
}
}
3.2 Bellman Ford Algorithm - The Cost Calculator
Similar to Dijkstra, but can handle situations where some paths might have negative impacts - like currency exchange rates where you might actually gain money in a conversion loop. It's more thorough but slower, checking every possible path multiple times.
A ---(-2)--โ B โ โฑ โ | โฑ | (4)|โฑ(3) |(2) | โ D โ--(-1)-- C
Real-world use: Currency exchange rates, network routing with negative weights
Java Implementation:
public class BellmanFord {
public void findShortestPaths(int[][] graph, int source) {
int V = graph.length;
int[] dist = new int[V];
Arrays.fill(dist, Integer.MAX_VALUE);
dist[source] = 0;
// Relax all edges V-1 times
for (int i = 0; i < V - 1; i++) {
for (int u = 0; u < V; u++) {
for (int v = 0; v < V; v++) {
if (graph[u][v] != 0 &&
dist[u] != Integer.MAX_VALUE &&
dist[u] + graph[u][v] < dist[v]) {
dist[v] = dist[u] + graph[u][v];
}
}
}
}
}
}
3.3 Floyd Warshall - The All-Pairs Pathfinder
Imagine planning a road trip where you want to know the shortest distance between every pair of cities on your map. Instead of calculating each route separately, this algorithm cleverly figures out all possible shortcuts between all locations at once!
โโAโโโโBโโโโCโโ โ โ โ โ โ DโโโโEโโโโF โ โ โ โ โโโGโโโโHโโโโIโ
Real-world use: Finding all possible routes between cities, social network connections
3.4 Topological Sort - The Task Scheduler
Like planning your college courses - you can't take Advanced Physics before Basic Physics! This algorithm arranges tasks in a perfect sequence where all prerequisites are completed first. Perfect for project planning or figuring out your study schedule.
Dependencies: Calculus โ Linear Algebra โ โ Statistics โ Data Science Order: Calculus โ Linear Algebra โ Statistics โ Data Science
Real-world use: Project scheduling, course prerequisites, build systems
3.5 Flood Fill - The Paint Bucket
Just like using the paint bucket tool in Photoshop! When you click on a white pixel, it fills all connected white pixels with your chosen color. It's like a digital version of how water spreads across a flat surface, reaching every connected space.
Before: After: โฌโฌโฌโฌโฌ โฌโฌโฌโฌโฌ โฌโฌโฌโฌโฌ โ โฌโฌโฌโฌโฌ โฌโฌโฌโฌโฌ โฌโฌ๐ต๐ต๐ต โฌโฌโฌโฌโฌ โฌโฌ๐ต๐ต๐ต
Real-world use: Image editing tools, game map exploration, maze solving
Java Implementation:
public class FloodFill {
public void fill(int[][] image, int sr, int sc, int newColor) {
if (image[sr][sc] != newColor) {
dfs(image, sr, sc, image[sr][sc], newColor);
}
}
private void dfs(int[][] image, int r, int c, int color, int newColor) {
if (r >= 0 && r < image.length && c >= 0 && c < image[0].length
&& image[r][c] == color) {
image[r][c] = newColor;
dfs(image, r+1, c, color, newColor);
dfs(image, r-1, c, color, newColor);
dfs(image, r, c+1, color, newColor);
dfs(image, r, c-1, color, newColor);
}
}
}
3.6 Lee Algorithm - The Maze Solver
Think of a robot trying to find its way through a maze. It marks each step with increasing numbers, like leaving numbered breadcrumbs, until it reaches the exit. Then it can trace back the shortest path by following decreasing numbers - super useful for designing circuit boards!
โฌSโฌโฌโฌ โฌS1234 โฌโฌโฌโฌโฌ โ โฌโฌ234โฌ โฌโฌโฌโฌโฌ 345โฌโฌE โฌโฌโฌโฌE 4โฌ5678
Real-world use: Circuit design, robot path planning
4. Array Algorithms ๐
4.1 Kadane's Algorithm - The Money Saver
Like finding the best streak of days where you saved the most pocket money!
Imagine tracking your daily fitness progress to find your best consecutive workout streak. This algorithm helps find the sequence of numbers that add up to the largest sum - perfect for finding the most productive period in any series of positive and negative values!
Java Example:
public class KadaneAlgo {
public static int findBestStreak(int[] savings) {
int currentSaving = 0;
int bestSaving = 0;
for(int today : savings) {
currentSaving = Math.max(0, currentSaving + today);
bestSaving = Math.max(bestSaving, currentSaving);
}
return bestSaving;
}
}
4.2 Floyd's Cycle Detection - The Tortoise and Hare
Picture a race track where one runner moves twice as fast as another. If there's a loop in the track, they'll eventually meet! This clever trick helps find loops in sequences, like detecting infinite loops in computer programs.
Startโ1โ2โ3โ4โ5 โ โ 8โ7โ6 ๐ข = Slow pointer ๐ = Fast pointer
Real-world use: Detecting loops in linked lists, finding repeated elements
4.3 KMP Algorithm - The Pattern Matcher
Like using Ctrl+F in a document, but way smarter! Instead of starting over when a pattern match fails, it remembers what it learned from previous comparisons to skip unnecessary checks. Perfect for finding specific patterns in text or DNA sequences.
Text: ABABDABACDABABCABAB Pattern: ABABCABAB โ Match: ABABCABAB Found at position: 10
Real-world use: Text editors' find function, DNA sequence matching
4.4 Quick Select - The Rank Finder
Like finding the third-tallest person in a group without sorting everyone. You use a clever divide-and-conquer strategy to find the exact element you want without sorting the entire list. Super useful for finding medians or the top-k elements in a dataset!
Find 3rd smallest: [7,2,9,1,5,4] โ [2,1,4|5|9,7] โ [1,2|4|5,9,7] Answer: 4
Real-world use: Finding median, statistics, top-k elements
4.5 Boyer-Moore Majority Vote - The Election Winner
Imagine being an election counter who can only remember one candidate at a time. Each time you see a vote for someone else, you cancel out one of your remembered votes. The person you remember at the end is likely the winner! It's a brilliant way to find the most common element in a list.
Array: [2,2,1,2,1,2,2] Step: 2 2 1 2 1 2 2 Count: 1 2 1 2 1 2 3 Major: 2 2 2 2 2 2 2
Real-world use: Finding majority element in voting systems, data stream analysis
Java Implementation:
public class BoyerMoore {
public int findMajority(int[] nums) {
int count = 0;
Integer candidate = null;
// Phase 1: Finding candidate
for (int num : nums) {
if (count == 0) {
candidate = num;
}
count += (num == candidate) ? 1 : -1;
}
// Phase 2: Verifying candidate
count = 0;
for (int num : nums) {
if (num == candidate) {
count++;
}
}
return count > nums.length/2 ? candidate : -1;
}
}
5. Basic Algorithms ๐ง
5.1 Huffman Coding - The Data Compressor
Think of it as creating the perfect text abbreviations - common words get shorter codes, rare ones get longer codes. Just like how 'lol' is shorter than 'laugh out loud'. This smart compression technique saves space while making sure there's no confusion in decoding!
Frequency Tree: [ROOT] / \ A:45 B:55 / \ / \ C:20 D:25 E:25 F:30
Real-world use: File compression (ZIP files), image compression
5.2 Euclidean Algorithm - The Common Factor Finder
Like finding the biggest pizza size that can evenly feed two different group sizes. By repeatedly dividing the larger number by the smaller one and keeping track of remainders, you find the largest number that divides both perfectly. Essential for simplifying fractions!
GCD(48,18) 48 = 2 ร 18 + 12 18 = 1 ร 12 + 6 12 = 2 ร 6 + 0 GCD = 6 โโโโโโโโโโโ โโโโโโโ โ 48 โโโโโโโโโโโ โโโโโโโ โโโโโ โ 18 โโโโโโโ
Real-world use: Cryptography, reducing fractions to lowest terms
5.3 Union Find - The Group Connector
Picture organizing people into friend groups at a party. When two people become friends, their entire groups merge! This algorithm efficiently tracks these groups and can quickly tell if any two people are in the same group - perfect for social networks!
Initial: 1 2 3 4 5 โ โ โ โ โ Sets: [1][2][3][4][5] After Union(1,2) and Union(3,4): 1 2 3 4 5 โ โ โ โ โ Sets: [1][1][3][3][5]
Real-world use: Network connectivity, social networks friend groups
Quick Navigation
Quick Navigation
Time Complexity Guide
- O(1) - Constant
- O(log n) - Logarithmic
- O(n) - Linear
- O(n log n) - Linearithmic
- O(nยฒ) - Quadratic