Advanced Memory Management in Java: Beyond Garbage Collection (2025)
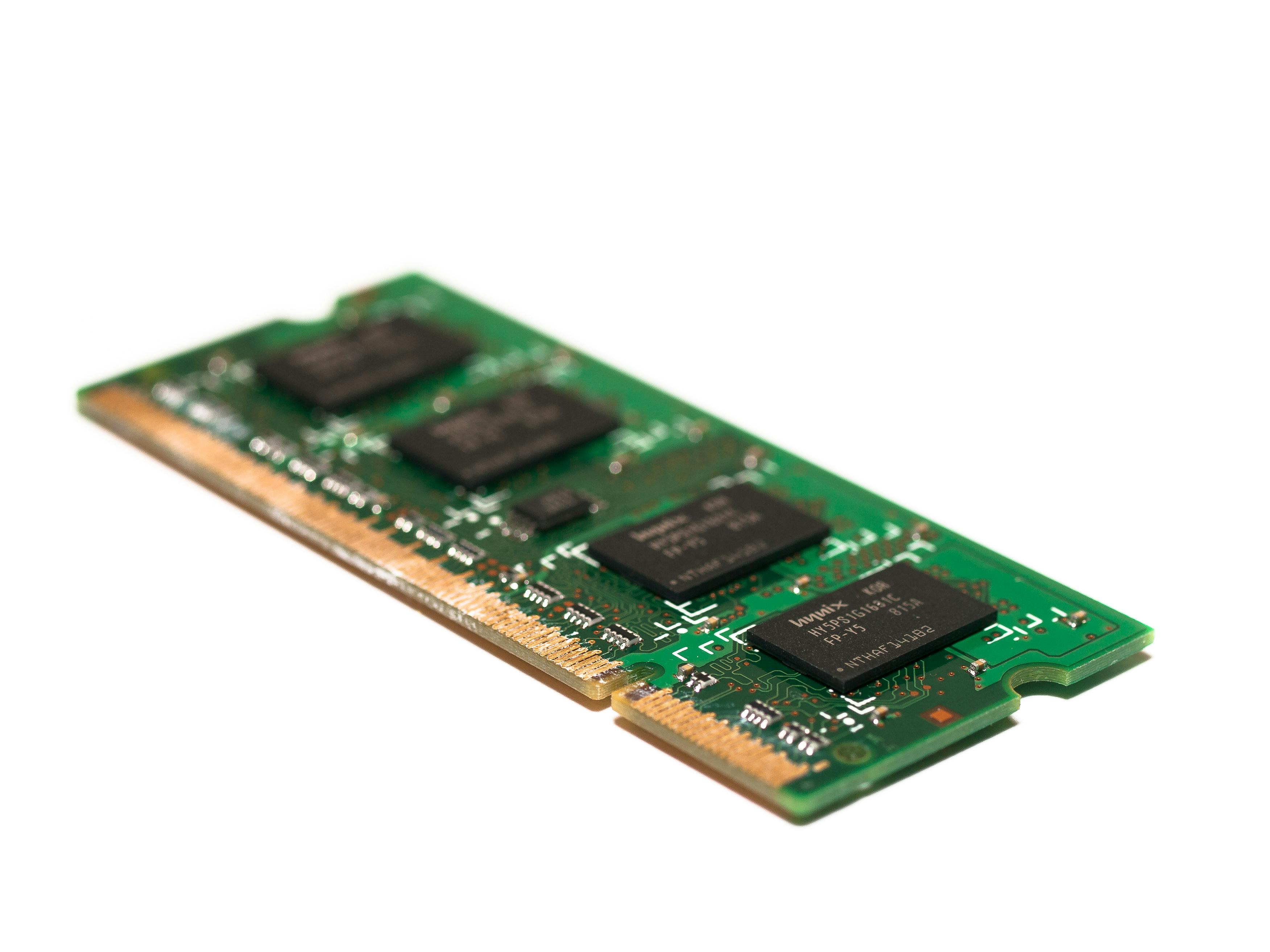
Memory management in Java goes far beyond simple garbage collection. This comprehensive guide explores advanced memory management concepts, techniques, and best practices for enterprise Java applications.
Pro Tip: Understanding Java's memory model is crucial for developing high-performance applications and preventing memory-related issues.
Table of Contents
Java Memory Model
Note: The Java Memory Model (JMM) defines how threads interact with memory and how changes made by one thread become visible to others.
Memory Areas
Memory Area | Description | Key Characteristics |
---|---|---|
Heap | Runtime data area for object allocation | Shared by all threads, managed by GC |
Stack | Thread-specific memory area | Stores local variables and method frames |
Metaspace | Class metadata storage | Replaced PermGen in Java 8+ |
Direct Memory | Native memory allocation | Managed outside JVM heap |
Direct Memory Management
Pro Tip: Direct memory operations can significantly improve performance for large data operations but require careful management.
Direct ByteBuffer Example
// Example of DirectByteBuffer usage
public class DirectMemoryExample {
public void processLargeData() {
// Allocate direct memory
ByteBuffer buffer = ByteBuffer.allocateDirect(1024 * 1024); // 1MB
try {
// Write data to direct memory
buffer.put(new byte[1024 * 1024]);
buffer.flip();
// Process data
while (buffer.hasRemaining()) {
byte b = buffer.get();
// Process byte
}
} finally {
// Clean up
buffer.clear();
}
}
}
Memory Leak Detection
Note: Memory leaks in Java often occur due to improper resource management or circular references.
Common Memory Leak Patterns
// Example of a memory leak due to static collection
public class MemoryLeakExample {
private static final Map cache = new HashMap<>();
public void addToCache(String key, Object value) {
cache.put(key, value);
}
// Missing cleanup method
}
// Correct implementation with cleanup
public class MemoryLeakFix {
private static final Map cache = new HashMap<>();
public void addToCache(String key, Object value) {
cache.put(key, value);
}
public void removeFromCache(String key) {
cache.remove(key);
}
public void clearCache() {
cache.clear();
}
}
Off-Heap Memory
Pro Tip: Off-heap memory can be used to store large datasets without affecting garbage collection.
Off-Heap Storage Example
public class OffHeapStorage {
private final Unsafe unsafe;
private long address;
private final long size;
public OffHeapStorage(long size) throws Exception {
this.size = size;
Field field = Unsafe.class.getDeclaredField("theUnsafe");
field.setAccessible(true);
unsafe = (Unsafe) field.get(null);
address = unsafe.allocateMemory(size);
}
public void write(byte[] data, long offset) {
unsafe.copyMemory(data, Unsafe.ARRAY_BYTE_BASE_OFFSET, null, address + offset, data.length);
}
public void read(byte[] data, long offset) {
unsafe.copyMemory(null, address + offset, data, Unsafe.ARRAY_BYTE_BASE_OFFSET, data.length);
}
public void free() {
unsafe.freeMemory(address);
}
}
Memory Optimization Techniques
Object Pooling
public class ObjectPool {
private final Queue pool;
private final Supplier objectFactory;
public ObjectPool(int size, Supplier objectFactory) {
this.pool = new ConcurrentLinkedQueue<>();
this.objectFactory = objectFactory;
// Pre-populate pool
for (int i = 0; i < size; i++) {
pool.offer(objectFactory.get());
}
}
public T borrow() {
T obj = pool.poll();
return obj != null ? obj : objectFactory.get();
}
public void returnObject(T obj) {
pool.offer(obj);
}
}
Memory Monitoring
Note: Regular memory monitoring is essential for identifying potential issues before they impact application performance.
Memory Monitoring Tools
- JConsole
- VisualVM
- Java Flight Recorder
- JProfiler
- MAT (Memory Analyzer Tool)
Best Practices
Pro Tip: Following memory management best practices can prevent common issues and improve application performance.
Key Best Practices
- Use appropriate collection types
- Implement proper resource cleanup
- Monitor memory usage
- Use weak references when appropriate
- Implement proper exception handling
- Use object pooling for frequently created objects
- Consider using off-heap storage for large datasets
Conclusion
Advanced memory management in Java requires a deep understanding of the JVM's memory model and various memory management techniques. By implementing proper memory management strategies and following best practices, developers can create more efficient and reliable applications.