Java Microservices Performance: A Complete Guide (2025)
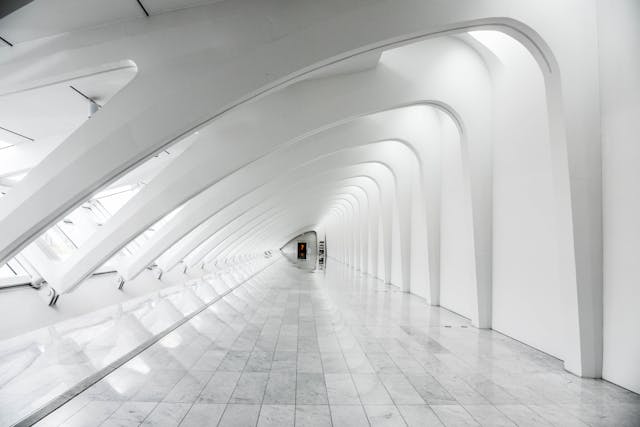
Building high-performance microservices in Java requires a deep understanding of various optimization techniques and best practices. This comprehensive guide explores key aspects of microservices performance, from caching strategies to monitoring and scaling.
Pro Tip: Performance optimization in microservices requires a holistic approach, considering both individual service performance and system-wide interactions.
Table of Contents
Caching Strategies
Note: Effective caching can significantly improve microservices performance by reducing database load and network calls.
Multi-Level Caching Example
public class MultiLevelCacheService {
private final Cache localCache;
private final RedisTemplate redisCache;
private final UserRepository userRepository;
public MultiLevelCacheService() {
// Local cache with Caffeine
this.localCache = Caffeine.newBuilder()
.maximumSize(1000)
.expireAfterWrite(5, TimeUnit.MINUTES)
.build();
// Redis cache configuration
this.redisCache = new RedisTemplate<>();
redisCache.setKeySerializer(new StringRedisSerializer());
redisCache.setValueSerializer(new GenericJackson2JsonRedisSerializer());
this.userRepository = new UserRepository();
}
public User getUser(String userId) {
// Try local cache first
User user = localCache.getIfPresent(userId);
if (user != null) {
return user;
}
// Try Redis cache
user = redisCache.opsForValue().get("user:" + userId);
if (user != null) {
localCache.put(userId, user);
return user;
}
// Fallback to database
user = userRepository.findById(userId);
if (user != null) {
localCache.put(userId, user);
redisCache.opsForValue().set("user:" + userId, user, 30, TimeUnit.MINUTES);
}
return user;
}
}
Load Balancing
Pro Tip: Effective load balancing is crucial for distributing traffic evenly across microservices instances.
Load Balancer Configuration Example
@Configuration
public class LoadBalancerConfig {
@Bean
public LoadBalancerClient loadBalancerClient() {
return new RibbonLoadBalancerClient();
}
@Bean
public IRule loadBalancingRule() {
return new WeightedResponseTimeRule();
}
@Bean
public IPing loadBalancerPing() {
return new PingUrl();
}
}
@Service
public class ServiceClient {
private final LoadBalancerClient loadBalancer;
private final RestTemplate restTemplate;
public ServiceClient(LoadBalancerClient loadBalancer) {
this.loadBalancer = loadBalancer;
this.restTemplate = new RestTemplate();
}
public String callService(String serviceId, String path) {
ServiceInstance instance = loadBalancer.choose(serviceId);
String url = instance.getUri().toString() + path;
return restTemplate.getForObject(url, String.class);
}
}
Database Optimization
Note: Database performance is often a critical factor in microservices performance.
Database Connection Pool Example
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/microservice_db");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(20);
config.setMinimumIdle(5);
config.setIdleTimeout(300000);
config.setConnectionTimeout(20000);
return new HikariDataSource(config);
}
@Bean
public JdbcTemplate jdbcTemplate(DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
}
@Service
public class DatabaseService {
private final JdbcTemplate jdbcTemplate;
public DatabaseService(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public List getUsers() {
return jdbcTemplate.query(
"SELECT * FROM users",
(rs, rowNum) -> new User(
rs.getString("id"),
rs.getString("name"),
rs.getString("email")
)
);
}
}
Asynchronous Processing
Pro Tip: Asynchronous processing can significantly improve microservices performance by handling non-blocking operations efficiently.
Async Processing Example
@Service
public class AsyncProcessingService {
private final ExecutorService executor;
private final KafkaTemplate kafkaTemplate;
public AsyncProcessingService() {
this.executor = Executors.newFixedThreadPool(10);
this.kafkaTemplate = new KafkaTemplate<>(producerFactory());
}
public CompletableFuture processOrderAsync(Order order) {
return CompletableFuture.runAsync(() -> {
// Process order asynchronously
try {
// Validate order
validateOrder(order);
// Send to message queue
kafkaTemplate.send("orders", order.getId(), order.toString());
// Update inventory
updateInventory(order);
// Send notification
sendNotification(order);
} catch (Exception e) {
handleError(order, e);
}
}, executor);
}
public void processBatchOrders(List orders) {
List> futures = orders.stream()
.map(this::processOrderAsync)
.collect(Collectors.toList());
CompletableFuture.allOf(futures.toArray(new CompletableFuture[0]))
.thenRun(() -> System.out.println("All orders processed"))
.exceptionally(throwable -> {
System.err.println("Error processing orders: " + throwable.getMessage());
return null;
});
}
}
Performance Monitoring
Note: Comprehensive monitoring is essential for identifying and resolving performance bottlenecks.
Monitoring Configuration Example
@Configuration
public class MonitoringConfig {
@Bean
public MeterRegistry meterRegistry() {
return new SimpleMeterRegistry();
}
@Bean
public TimedAspect timedAspect(MeterRegistry registry) {
return new TimedAspect(registry);
}
@Bean
public PrometheusMeterRegistry prometheusMeterRegistry() {
return new PrometheusMeterRegistry(PrometheusConfig.DEFAULT);
}
}
@Service
public class MonitoringService {
private final MeterRegistry meterRegistry;
public MonitoringService(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@Timed(value = "service.method.duration", description = "Time taken to process request")
public void monitorMethodExecution() {
Timer.Sample sample = Timer.start(meterRegistry);
try {
// Method implementation
Thread.sleep(100); // Simulate work
} finally {
sample.stop(Timer.builder("service.method.duration")
.tag("method", "monitorMethodExecution")
.register(meterRegistry));
}
}
public void recordMetrics() {
Counter counter = Counter.builder("service.requests")
.tag("type", "api")
.description("Total number of API requests")
.register(meterRegistry);
Gauge gauge = Gauge.builder("service.memory.usage",
Runtime.getRuntime(), this::getMemoryUsage)
.tag("type", "heap")
.description("Memory usage in bytes")
.register(meterRegistry);
}
}
Scaling Strategies
Pro Tip: Choose appropriate scaling strategies based on your application's specific needs and resource constraints.
Horizontal Scaling Example
@Configuration
public class ScalingConfig {
@Bean
public KubernetesClient kubernetesClient() {
return new DefaultKubernetesClient();
}
}
@Service
public class ScalingService {
private final KubernetesClient kubernetesClient;
public ScalingService(KubernetesClient kubernetesClient) {
this.kubernetesClient = kubernetesClient;
}
public void scaleDeployment(String deploymentName, int replicas) {
Deployment deployment = kubernetesClient.apps()
.deployments()
.inNamespace("default")
.withName(deploymentName)
.get();
deployment.getSpec().setReplicas(replicas);
kubernetesClient.apps()
.deployments()
.inNamespace("default")
.withName(deploymentName)
.replace(deployment);
}
public void autoScaleBasedOnMetrics(String deploymentName) {
HorizontalPodAutoscaler hpa = new HorizontalPodAutoscalerBuilder()
.withNewMetadata()
.withName(deploymentName + "-hpa")
.withNamespace("default")
.endMetadata()
.withNewSpec()
.withScaleTargetRef(new CrossVersionObjectReferenceBuilder()
.withKind("Deployment")
.withName(deploymentName)
.withApiVersion("apps/v1")
.build())
.withMinReplicas(2)
.withMaxReplicas(10)
.withTargetCPUUtilizationPercentage(80)
.endSpec()
.build();
kubernetesClient.autoscaling()
.horizontalPodAutoscalers()
.inNamespace("default")
.create(hpa);
}
}
Best Practices
Pro Tip: Following microservices performance best practices helps maintain system reliability and scalability.
Key Best Practices
- Implement circuit breakers for fault tolerance
- Use appropriate caching strategies
- Implement proper monitoring and alerting
- Use connection pooling for databases
- Implement rate limiting
- Use asynchronous processing where appropriate
- Implement proper error handling
- Use appropriate scaling strategies
Conclusion
Optimizing microservices performance requires a comprehensive approach that considers various aspects of the system. By implementing proper caching, load balancing, monitoring, and scaling strategies, you can build high-performance microservices that meet your application's requirements.