Java Concurrency Deep Dive: From Threads to Virtual Threads (2025)
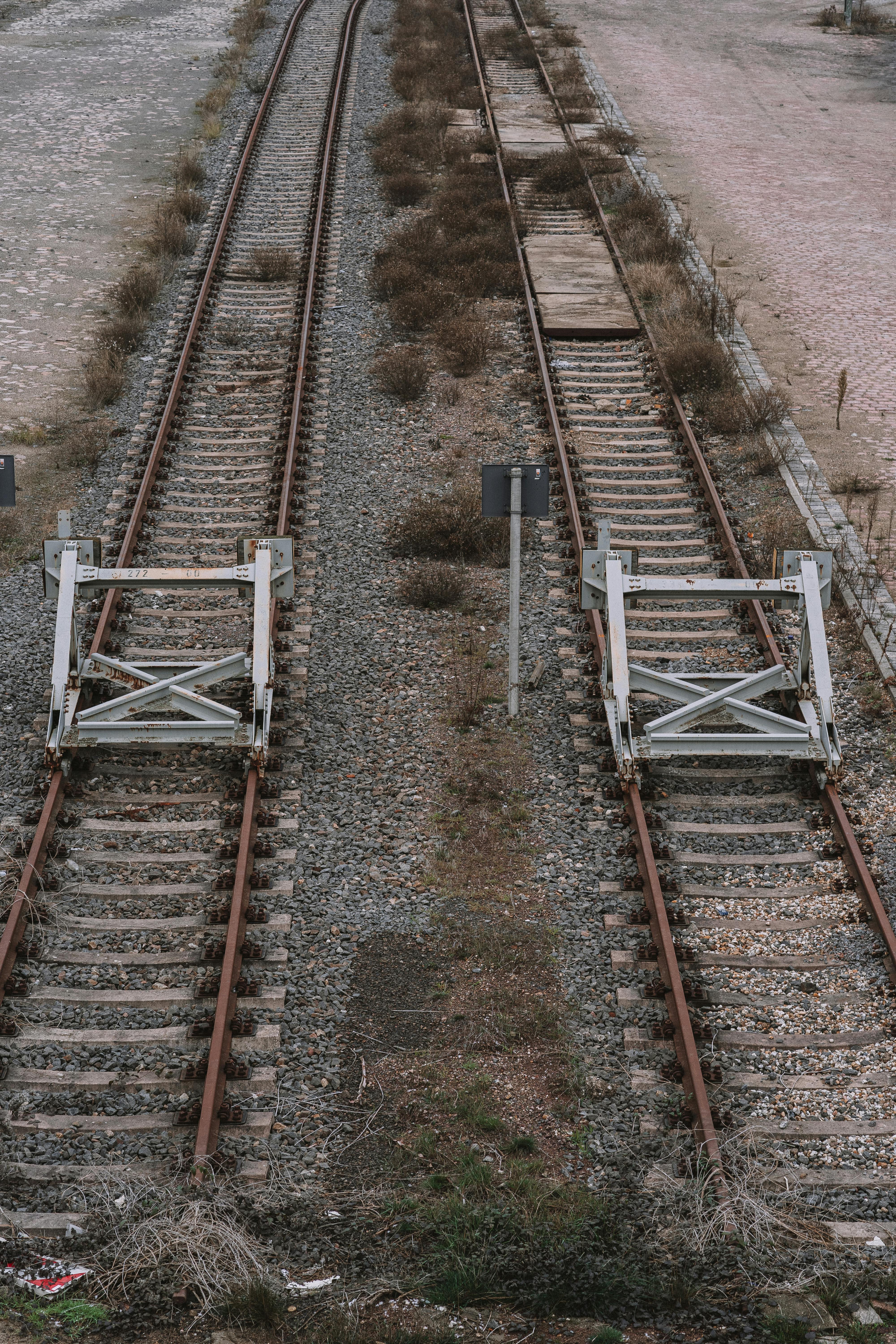
Java's concurrency model has evolved significantly from basic thread management to the revolutionary virtual threads introduced in Java 21. This comprehensive guide explores the entire spectrum of Java concurrency, from fundamental concepts to advanced patterns.
Pro Tip: Understanding Java's concurrency model is crucial for building scalable, high-performance applications.
Table of Contents
Thread Basics
Note: Understanding thread lifecycle and states is fundamental to Java concurrency.
Thread States and Lifecycle
Thread State | Description | How to Achieve |
---|---|---|
NEW | Thread created but not started | new Thread() |
RUNNABLE | Thread is executing | thread.start() |
BLOCKED | Thread blocked waiting for lock | synchronized block |
WAITING | Thread waiting indefinitely | object.wait() |
TIMED_WAITING | Thread waiting for specified time | Thread.sleep() |
TERMINATED | Thread completed execution | run() method completes |
Thread Pools
Pro Tip: Thread pools are essential for managing thread lifecycle and preventing resource exhaustion.
ThreadPoolExecutor Example
public class ThreadPoolExample {
private final ExecutorService executor;
public ThreadPoolExample() {
this.executor = new ThreadPoolExecutor(
5, // core pool size
10, // max pool size
60L, // keep alive time
TimeUnit.SECONDS, // time unit
new LinkedBlockingQueue<>(100), // work queue
new ThreadFactory() {
private final AtomicInteger counter = new AtomicInteger(1);
@Override
public Thread newThread(Runnable r) {
Thread thread = new Thread(r);
thread.setName("Worker-" + counter.getAndIncrement());
return thread;
}
},
new ThreadPoolExecutor.CallerRunsPolicy() // rejection policy
);
}
public Future submitTask(Callable task) {
return executor.submit(task);
}
public void shutdown() {
executor.shutdown();
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) {
executor.shutdownNow();
}
} catch (InterruptedException e) {
executor.shutdownNow();
Thread.currentThread().interrupt();
}
}
}
Concurrent Collections
Note: Concurrent collections provide thread-safe alternatives to standard collections.
ConcurrentHashMap Example
public class ConcurrentHashMapExample {
private final ConcurrentHashMap cache = new ConcurrentHashMap<>();
public void updateCache(String key, Integer value) {
// Atomic update
cache.compute(key, (k, v) -> {
if (v == null) return value;
return v + value;
});
}
public void removeIfPresent(String key) {
// Atomic remove
cache.remove(key, 0); // Only remove if value is 0
}
public void processAllEntries() {
// Thread-safe iteration
cache.forEach(1, (key, value) -> {
System.out.println("Processing: " + key + " = " + value);
});
}
}
Synchronization Mechanisms
Pro Tip: Modern Java provides multiple synchronization mechanisms beyond basic synchronized blocks.
ReentrantLock Example
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
private final Condition notFull = lock.newCondition();
private final Condition notEmpty = lock.newCondition();
public void put(Object item) throws InterruptedException {
lock.lock();
try {
while (queue.isFull()) {
notFull.await();
}
queue.add(item);
notEmpty.signal();
} finally {
lock.unlock();
}
}
public Object take() throws InterruptedException {
lock.lock();
try {
while (queue.isEmpty()) {
notEmpty.await();
}
Object item = queue.remove();
notFull.signal();
return item;
} finally {
lock.unlock();
}
}
}
Asynchronous Programming
Note: CompletableFuture provides a modern way to handle asynchronous operations.
CompletableFuture Example
public class AsyncExample {
private final ExecutorService executor = Executors.newFixedThreadPool(4);
public CompletableFuture processAsync(String input) {
return CompletableFuture.supplyAsync(() -> {
// Simulate processing
try {
Thread.sleep(1000);
return input.toUpperCase();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw new CompletionException(e);
}
}, executor)
.thenApply(result -> "Processed: " + result)
.exceptionally(throwable -> "Error: " + throwable.getMessage());
}
public CompletableFuture combineResults(
CompletableFuture future1,
CompletableFuture future2) {
return future1.thenCombine(future2, (result1, result2) ->
result1 + " | " + result2);
}
}
Virtual Threads
Pro Tip: Virtual threads in Java 21 provide lightweight concurrency without the overhead of platform threads.
Virtual Thread Example
public class VirtualThreadExample {
public void processWithVirtualThreads() {
// Create virtual thread
Thread vThread = Thread.startVirtualThread(() -> {
try {
// Simulate I/O operation
Thread.sleep(1000);
System.out.println("Virtual thread completed");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
// Create virtual thread with custom name
Thread namedVThread = Thread.ofVirtual()
.name("VirtualWorker-1")
.start(() -> {
// Process work
System.out.println("Named virtual thread working");
});
// Create virtual thread with custom uncaught exception handler
Thread errorHandledVThread = Thread.ofVirtual()
.uncaughtExceptionHandler((thread, throwable) ->
System.err.println("Error in virtual thread: " + throwable.getMessage()))
.start(() -> {
throw new RuntimeException("Test exception");
});
}
public void structuredConcurrency() {
try (var scope = new StructuredTaskScope.ShutdownOnFailure()) {
Future future1 = scope.fork(() -> {
Thread.sleep(1000);
return "Result 1";
});
Future future2 = scope.fork(() -> {
Thread.sleep(500);
return "Result 2";
});
scope.join();
scope.throwIfFailed();
String result1 = future1.resultNow();
String result2 = future2.resultNow();
System.out.println(result1 + " | " + result2);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Best Practices
Pro Tip: Following concurrency best practices helps prevent common issues and improves application reliability.
Key Best Practices
- Use thread pools instead of creating threads directly
- Prefer concurrent collections over synchronized collections
- Use CompletableFuture for asynchronous operations
- Implement proper exception handling in threads
- Use virtual threads for I/O-bound operations
- Follow the principle of least privilege
- Implement proper shutdown hooks
- Use appropriate synchronization mechanisms
Conclusion
Java's concurrency model has evolved to provide powerful tools for building scalable applications. From basic thread management to modern virtual threads, understanding these concepts is crucial for developing high-performance applications.