Secure Coding in Java: Advanced Techniques (2025)
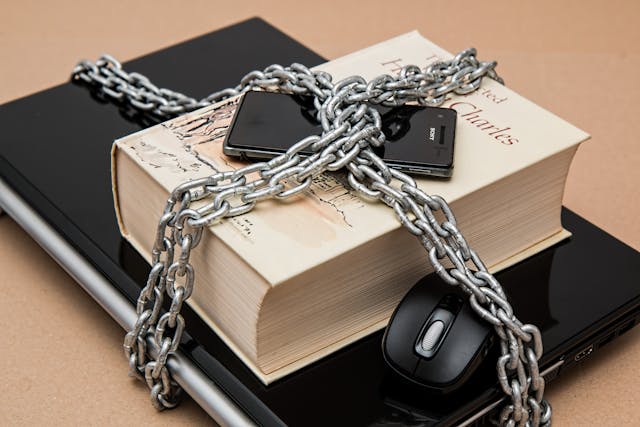
Secure coding is essential for building robust and reliable Java applications. This comprehensive guide explores advanced secure coding techniques, patterns, and best practices.
Pro Tip: Following secure coding practices helps prevent vulnerabilities and ensures application reliability.
Table of Contents
Memory Safety
Note: Proper memory management is crucial for preventing memory leaks and security vulnerabilities.
Memory Management Implementation
public class SecureMemoryManager {
private final Map sensitiveData;
private final ScheduledExecutorService cleanupExecutor;
public SecureMemoryManager() {
this.sensitiveData = Collections.synchronizedMap(new HashMap<>());
this.cleanupExecutor = Executors.newSingleThreadScheduledExecutor();
scheduleCleanup();
}
public void storeSensitiveData(String key, byte[] data) {
// Create a copy of the data
byte[] secureCopy = data.clone();
sensitiveData.put(key, secureCopy);
}
public byte[] retrieveSensitiveData(String key) {
byte[] data = sensitiveData.get(key);
return data != null ? data.clone() : null;
}
public void clearSensitiveData(String key) {
byte[] data = sensitiveData.remove(key);
if (data != null) {
Arrays.fill(data, (byte) 0);
}
}
private void scheduleCleanup() {
cleanupExecutor.scheduleAtFixedRate(() -> {
sensitiveData.forEach((key, data) -> {
if (isDataExpired(key)) {
clearSensitiveData(key);
}
});
}, 1, 1, TimeUnit.HOURS);
}
private boolean isDataExpired(String key) {
// Implement expiration logic
return false;
}
public void shutdown() {
cleanupExecutor.shutdown();
try {
if (!cleanupExecutor.awaitTermination(5, TimeUnit.SECONDS)) {
cleanupExecutor.shutdownNow();
}
} catch (InterruptedException e) {
cleanupExecutor.shutdownNow();
Thread.currentThread().interrupt();
}
}
}
Thread Safety
Pro Tip: Proper thread synchronization is essential for concurrent applications.
Thread-Safe Implementation
public class ThreadSafeCache {
private final Map cache;
private final ReadWriteLock lock;
private final Lock readLock;
private final Lock writeLock;
public ThreadSafeCache() {
this.cache = new HashMap<>();
this.lock = new ReentrantReadWriteLock();
this.readLock = lock.readLock();
this.writeLock = lock.writeLock();
}
public V get(K key) {
readLock.lock();
try {
return cache.get(key);
} finally {
readLock.unlock();
}
}
public void put(K key, V value) {
writeLock.lock();
try {
cache.put(key, value);
} finally {
writeLock.unlock();
}
}
public void remove(K key) {
writeLock.lock();
try {
cache.remove(key);
} finally {
writeLock.unlock();
}
}
public void clear() {
writeLock.lock();
try {
cache.clear();
} finally {
writeLock.unlock();
}
}
}
public class SecureCounter {
private final AtomicLong counter;
private final AtomicLong lastUpdateTime;
public SecureCounter() {
this.counter = new AtomicLong(0);
this.lastUpdateTime = new AtomicLong(System.currentTimeMillis());
}
public long increment() {
long currentTime = System.currentTimeMillis();
long lastUpdate = lastUpdateTime.get();
// Prevent rapid increments
if (currentTime - lastUpdate < 100) {
throw new IllegalStateException("Too many increments");
}
lastUpdateTime.set(currentTime);
return counter.incrementAndGet();
}
public long getValue() {
return counter.get();
}
}
Resource Management
Note: Proper resource management prevents resource leaks and ensures system stability.
Resource Management Implementation
public class SecureResourceManager implements AutoCloseable {
private final Set resources;
private final Lock resourceLock;
public SecureResourceManager() {
this.resources = Collections.synchronizedSet(new HashSet<>());
this.resourceLock = new ReentrantLock();
}
public T register(T resource) {
resourceLock.lock();
try {
resources.add(resource);
return resource;
} finally {
resourceLock.unlock();
}
}
public void unregister(Closeable resource) {
resourceLock.lock();
try {
resources.remove(resource);
} finally {
resourceLock.unlock();
}
}
@Override
public void close() {
resourceLock.lock();
try {
for (Closeable resource : resources) {
try {
resource.close();
} catch (IOException e) {
// Log error but continue closing other resources
}
}
resources.clear();
} finally {
resourceLock.unlock();
}
}
}
public class SecureFileProcessor {
private final Path secureDirectory;
private final int maxFileSize;
public SecureFileProcessor(Path secureDirectory, int maxFileSize) {
this.secureDirectory = secureDirectory.normalize();
this.maxFileSize = maxFileSize;
}
public void processFile(Path filePath) throws IOException {
// Validate file path
if (!filePath.normalize().startsWith(secureDirectory)) {
throw new SecurityException("Invalid file path");
}
// Check file size
if (Files.size(filePath) > maxFileSize) {
throw new IllegalArgumentException("File too large");
}
// Process file with try-with-resources
try (InputStream is = Files.newInputStream(filePath);
BufferedInputStream bis = new BufferedInputStream(is);
OutputStream os = Files.newOutputStream(filePath.resolveSibling("processed_" + filePath.getFileName()));
BufferedOutputStream bos = new BufferedOutputStream(os)) {
byte[] buffer = new byte[8192];
int bytesRead;
while ((bytesRead = bis.read(buffer)) != -1) {
bos.write(buffer, 0, bytesRead);
}
bos.flush();
}
}
}
Secure Input Handling
Pro Tip: Proper input validation and sanitization prevents injection attacks.
Input Handling Implementation
public class SecureInputHandler {
private static final Pattern SAFE_STRING_PATTERN =
Pattern.compile("^[a-zA-Z0-9\\s.,!?-]*$");
private static final int MAX_INPUT_LENGTH = 1000;
public String sanitizeInput(String input) {
if (input == null) {
return null;
}
// Check length
if (input.length() > MAX_INPUT_LENGTH) {
throw new IllegalArgumentException("Input too long");
}
// Validate pattern
if (!SAFE_STRING_PATTERN.matcher(input).matches()) {
throw new IllegalArgumentException("Invalid input characters");
}
// Trim and normalize
return input.trim().normalize(Normalizer.Form.NFKC);
}
public String sanitizeHtml(String html) {
if (html == null) {
return null;
}
// Use JSoup for HTML sanitization
return Jsoup.clean(html, Whitelist.basic());
}
public String sanitizeSql(String sql) {
if (sql == null) {
return null;
}
// Remove SQL comments
sql = sql.replaceAll("--.*$", "");
sql = sql.replaceAll("/\\*.*?\\*/", "");
// Remove multiple spaces
sql = sql.replaceAll("\\s+", " ");
// Validate against SQL injection patterns
if (sql.matches("(?i).*(union|select|insert|update|delete|drop|alter).*")) {
throw new IllegalArgumentException("Invalid SQL input");
}
return sql;
}
}
Secure Serialization
Note: Secure serialization prevents deserialization vulnerabilities.
Secure Serialization Implementation
public class SecureSerializer {
private final ObjectInputFilter filter;
public SecureSerializer() {
this.filter = createObjectInputFilter();
}
public byte[] serialize(Object obj) throws IOException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos)) {
oos.writeObject(obj);
return baos.toByteArray();
}
}
public Object deserialize(byte[] data) throws IOException, ClassNotFoundException {
try (ByteArrayInputStream bais = new ByteArrayInputStream(data);
ObjectInputStream ois = new ObjectInputStream(bais)) {
ois.setObjectInputFilter(filter);
return ois.readObject();
}
}
private ObjectInputFilter createObjectInputFilter() {
return filterInfo -> {
Class clazz = filterInfo.serialClass();
if (clazz == null) {
return ObjectInputFilter.Status.ALLOWED;
}
// Check for dangerous classes
if (clazz.getName().startsWith("java.lang.invoke") ||
clazz.getName().startsWith("java.lang.reflect") ||
clazz.getName().startsWith("java.lang.Process")) {
return ObjectInputFilter.Status.REJECTED;
}
return ObjectInputFilter.Status.ALLOWED;
};
}
}
@SuppressWarnings("serial")
public class SecureSerializable implements Serializable {
private static final long serialVersionUID = 1L;
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
// Add additional security checks
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Validate object state
validateState();
}
private void validateState() {
// Implement state validation
}
}
Best Practices
Pro Tip: Following secure coding best practices helps maintain code quality and security.
Secure Coding Best Practices
- Use immutable objects where possible
- Implement proper exception handling
- Use secure random number generation
- Validate all inputs
- Use prepared statements for SQL
- Implement proper logging
- Use secure string comparison
- Implement proper access controls
- Use secure file operations
- Implement proper session management
- Use secure communication protocols
- Implement proper error handling
- Use secure configuration management
- Implement proper resource cleanup
- Follow secure coding guidelines
Conclusion
Secure coding is essential for building reliable and secure Java applications. By implementing these advanced techniques and following best practices, developers can create more robust and secure code.