Java Security Best Practices: Beyond OWASP (2025)
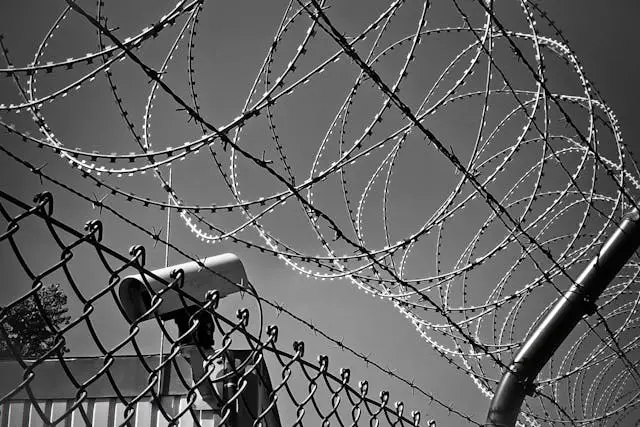
Security is crucial in modern Java applications. This comprehensive guide explores advanced security practices, patterns, and techniques beyond basic OWASP guidelines.
Pro Tip: Implementing comprehensive security measures helps protect applications from evolving threats.
Table of Contents
Advanced Encryption
Note: Proper encryption is essential for protecting sensitive data in transit and at rest.
Encryption Implementation
public class EncryptionService {
private static final String ALGORITHM = "AES/GCM/NoPadding";
private static final int GCM_IV_LENGTH = 12;
private static final int GCM_TAG_LENGTH = 16;
private final SecretKey key;
public EncryptionService(byte[] keyBytes) {
this.key = new SecretKeySpec(keyBytes, "AES");
}
public String encrypt(String plaintext) throws Exception {
byte[] iv = generateIV();
Cipher cipher = Cipher.getInstance(ALGORITHM);
GCMParameterSpec spec = new GCMParameterSpec(GCM_TAG_LENGTH * 8, iv);
cipher.init(Cipher.ENCRYPT_MODE, key, spec);
byte[] encrypted = cipher.doFinal(plaintext.getBytes(StandardCharsets.UTF_8));
byte[] combined = new byte[iv.length + encrypted.length];
System.arraycopy(iv, 0, combined, 0, iv.length);
System.arraycopy(encrypted, 0, combined, iv.length, encrypted.length);
return Base64.getEncoder().encodeToString(combined);
}
public String decrypt(String ciphertext) throws Exception {
byte[] combined = Base64.getDecoder().decode(ciphertext);
byte[] iv = new byte[GCM_IV_LENGTH];
byte[] encrypted = new byte[combined.length - GCM_IV_LENGTH];
System.arraycopy(combined, 0, iv, 0, GCM_IV_LENGTH);
System.arraycopy(combined, GCM_IV_LENGTH, encrypted, 0, encrypted.length);
Cipher cipher = Cipher.getInstance(ALGORITHM);
GCMParameterSpec spec = new GCMParameterSpec(GCM_TAG_LENGTH * 8, iv);
cipher.init(Cipher.DECRYPT_MODE, key, spec);
byte[] decrypted = cipher.doFinal(encrypted);
return new String(decrypted, StandardCharsets.UTF_8);
}
private byte[] generateIV() {
byte[] iv = new byte[GCM_IV_LENGTH];
new SecureRandom().nextBytes(iv);
return iv;
}
}
Authentication & Authorization
Pro Tip: Implement strong authentication and fine-grained authorization for better security.
JWT Authentication
@Service
public class JwtAuthenticationService {
private final JwtProperties jwtProperties;
private final Key key;
@Autowired
public JwtAuthenticationService(JwtProperties jwtProperties) {
this.jwtProperties = jwtProperties;
this.key = Keys.hmacShaKeyFor(jwtProperties.getSecret().getBytes());
}
public String generateToken(UserDetails userDetails) {
Map claims = new HashMap<>();
claims.put("sub", userDetails.getUsername());
claims.put("roles", userDetails.getAuthorities().stream()
.map(GrantedAuthority::getAuthority)
.collect(Collectors.toList()));
return Jwts.builder()
.setClaims(claims)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + jwtProperties.getExpiration()))
.signWith(key, SignatureAlgorithm.HS512)
.compact();
}
public Claims validateToken(String token) {
try {
return Jwts.parserBuilder()
.setSigningKey(key)
.build()
.parseClaimsJws(token)
.getBody();
} catch (JwtException e) {
throw new InvalidTokenException("Invalid JWT token", e);
}
}
}
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.antMatchers("/api/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
@Bean
public JwtAuthenticationFilter jwtAuthenticationFilter() {
return new JwtAuthenticationFilter();
}
}
Secure Coding Practices
Note: Secure coding practices help prevent common vulnerabilities.
Secure Exception Handling
@ControllerAdvice
public class GlobalExceptionHandler {
private final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(Exception.class)
public ResponseEntity handleException(Exception ex) {
logger.error("Unexpected error occurred", ex);
// Don't expose internal error details
ErrorResponse response = new ErrorResponse(
"An unexpected error occurred",
"INTERNAL_ERROR"
);
return ResponseEntity
.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body(response);
}
@ExceptionHandler(ValidationException.class)
public ResponseEntity handleValidationException(ValidationException ex) {
ErrorResponse response = new ErrorResponse(
ex.getMessage(),
"VALIDATION_ERROR"
);
return ResponseEntity
.status(HttpStatus.BAD_REQUEST)
.body(response);
}
}
public class SecureFileService {
public void processFile(Path filePath) {
// Validate file path
if (!filePath.normalize().startsWith("/secure/upload/")) {
throw new SecurityException("Invalid file path");
}
// Check file size
if (Files.size(filePath) > MAX_FILE_SIZE) {
throw new ValidationException("File too large");
}
// Validate file type
String contentType = Files.probeContentType(filePath);
if (!ALLOWED_CONTENT_TYPES.contains(contentType)) {
throw new ValidationException("Invalid file type");
}
// Process file securely
try (InputStream is = Files.newInputStream(filePath)) {
// Process file content
}
}
}
Input Validation
Pro Tip: Strong input validation prevents injection attacks and data corruption.
Input Validation Implementation
public class InputValidator {
private static final Pattern USERNAME_PATTERN =
Pattern.compile("^[a-zA-Z0-9_]{3,20}$");
private static final Pattern EMAIL_PATTERN =
Pattern.compile("^[A-Za-z0-9+_.-]+@(.+)$");
public void validateUserInput(UserInput input) {
List errors = new ArrayList<>();
// Validate username
if (!USERNAME_PATTERN.matcher(input.getUsername()).matches()) {
errors.add("Invalid username format");
}
// Validate email
if (!EMAIL_PATTERN.matcher(input.getEmail()).matches()) {
errors.add("Invalid email format");
}
// Validate password strength
if (!isPasswordStrong(input.getPassword())) {
errors.add("Password does not meet security requirements");
}
// Validate and sanitize HTML content
if (input.getBio() != null) {
input.setBio(sanitizeHtml(input.getBio()));
}
if (!errors.isEmpty()) {
throw new ValidationException(errors);
}
}
private boolean isPasswordStrong(String password) {
return password != null
&& password.length() >= 8
&& password.matches(".*[A-Z].*")
&& password.matches(".*[a-z].*")
&& password.matches(".*\\d.*")
&& password.matches(".*[!@#$%^&*()].*");
}
private String sanitizeHtml(String html) {
return Jsoup.clean(html, Whitelist.basic());
}
}
Secure Configuration
Note: Secure configuration management is crucial for application security.
Configuration Management
@Configuration
@EnableConfigurationProperties
public class SecurityConfig {
@Bean
public SecurityProperties securityProperties() {
return new SecurityProperties();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder(12);
}
}
@ConfigurationProperties(prefix = "security")
@Component
public class SecurityProperties {
private String jwtSecret;
private long jwtExpiration;
private List allowedOrigins;
private boolean csrfEnabled;
// Getters and setters
}
// application.yml
security:
jwt:
secret: ${JWT_SECRET:your-secure-secret-key}
expiration: 86400000
cors:
allowed-origins:
- https://trusted-domain.com
- https://api.secure-app.com
csrf:
enabled: true
rate-limit:
enabled: true
max-requests: 100
window-seconds: 60
Best Practices
Pro Tip: Following security best practices helps maintain a robust security posture.
Security Best Practices
- Use strong encryption algorithms
- Implement proper key management
- Follow the principle of least privilege
- Validate all inputs
- Use secure session management
- Implement proper error handling
- Use secure configuration management
- Regular security audits
- Keep dependencies updated
- Implement logging and monitoring
- Use secure communication protocols
- Implement rate limiting
- Regular security testing
- Follow secure coding guidelines
- Implement proper access controls
Conclusion
Security is an ongoing process that requires constant attention and updates. By implementing these advanced security practices and following best practices, developers can build more secure Java applications.