Mutation Testing in Java: Beyond Code Coverage (2025)
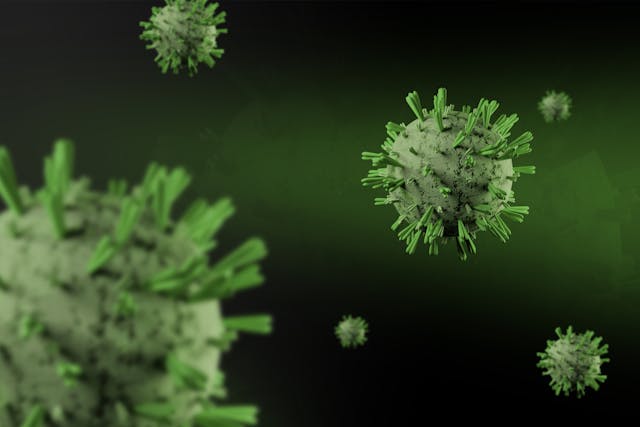
Mutation testing goes beyond traditional code coverage metrics to evaluate the quality of your test suite. This comprehensive guide explores advanced mutation testing techniques and tools in Java.
Table of Contents
PITest Integration
PITest is a powerful mutation testing tool specifically designed for Java applications. It helps developers evaluate the quality of their test suites by systematically introducing small changes (mutations) to the code and checking if the tests can detect these changes. This section covers how to integrate PITest into your Maven project and configure it for optimal results.
PITest Configuration
<plugin>
<groupId>org.pitest</groupId>
<artifactId>pitest-maven</artifactId>
<version>1.15.0</version>
<configuration>
<targetClasses>
<param>com.example.order.*</param>
</targetClasses>
<targetTests>
<param>com.example.order.*Test</param>
</targetTests>
<mutationEngine>gregor</mutationEngine>
<outputFormats>
<outputFormat>HTML</outputFormat>
<outputFormat>XML</outputFormat>
</outputFormats>
<failWhenNoMutations>false</failWhenNoMutations>
<timestampedReports>false</timestampedReports>
</configuration>
<dependencies>
<dependency>
<groupId>org.pitest</groupId>
<artifactId>pitest-junit5-plugin</artifactId>
<version>1.1.2</version>
</dependency>
</dependencies>
</plugin>
Running PITest
public class OrderValidatorTest {
private OrderValidator validator;
@BeforeEach
void setUp() {
validator = new OrderValidator();
}
@Test
void shouldValidateValidOrder() {
Order order = new Order(100.0, "USD");
assertTrue(validator.isValid(order));
}
@Test
void shouldRejectInvalidOrder() {
Order order = new Order(-100.0, "USD");
assertFalse(validator.isValid(order));
}
@Test
void shouldRejectNullCurrency() {
Order order = new Order(100.0, null);
assertFalse(validator.isValid(order));
}
}
Mutation Operators
Mutation operators are the building blocks of mutation testing. They define the types of changes that can be made to your code to create mutants. Understanding different mutation operators is crucial for effective mutation testing, as they help identify potential weaknesses in your test suite. This section explores common mutation operators and their impact on code quality assessment.
Common Mutation Operators
public class OrderValidator {
// Original code
public boolean isValid(Order order) {
return order != null
&& order.getAmount() > 0
&& order.getCurrency() != null
&& !order.getCurrency().isEmpty();
}
// Arithmetic Operator Mutation
public boolean isValid(Order order) {
return order != null
&& order.getAmount() >= 0 // Changed > to >=
&& order.getCurrency() != null
&& !order.getCurrency().isEmpty();
}
// Logical Operator Mutation
public boolean isValid(Order order) {
return order != null
&& order.getAmount() > 0
&& order.getCurrency() != null
&& order.getCurrency().isEmpty(); // Changed !isEmpty() to isEmpty()
}
// Conditional Operator Mutation
public boolean isValid(Order order) {
return order == null // Changed != to ==
&& order.getAmount() > 0
&& order.getCurrency() != null
&& !order.getCurrency().isEmpty();
}
}
Custom Operators
While PITest provides a comprehensive set of built-in mutation operators, sometimes you need specialized operators for your specific use cases. Custom operators allow you to define your own mutation rules that are tailored to your application's requirements. This section demonstrates how to create and implement custom mutation operators to enhance your testing strategy.
Custom Operator Implementation
public class CustomMutationOperator extends MutationOperator {
@Override
public List apply(MutationDetails details) {
List mutations = new ArrayList<>();
// Example: Mutate string comparisons
if (details.getMethod().getName().contains("validate")) {
mutations.add(createStringComparisonMutation(details));
}
return mutations;
}
private MutationDetails createStringComparisonMutation(MutationDetails details) {
return new MutationDetails(
details.getId(),
details.getFilename(),
details.getLineNumber(),
details.getBlock(),
details.getIndexInBlock(),
details.getDescription()
);
}
}
// Configuration in pom.xml
org.pitest
pitest-maven
custom
com.example.CustomMutationOperator
Mutation Analysis
Mutation analysis is the process of evaluating the results of mutation testing to understand the quality of your test suite. This involves calculating mutation scores, identifying surviving mutations, and generating detailed reports. This section covers how to analyze mutation testing results and use them to improve your test coverage.
Analyzing Results
public class MutationAnalysis {
public void analyzeResults(File resultsFile) {
// Parse PITest results
MutationResults results = parseResults(resultsFile);
// Calculate metrics
double mutationScore = calculateMutationScore(results);
List survivingMutations = findSurvivingMutations(results);
// Generate report
generateReport(mutationScore, survivingMutations);
}
private double calculateMutationScore(MutationResults results) {
int totalMutations = results.getTotalMutations();
int killedMutations = results.getKilledMutations();
return (double) killedMutations / totalMutations;
}
private void generateReport(double mutationScore, List survivingMutations) {
System.out.println("Mutation Testing Results:");
System.out.println("Mutation Score: " + (mutationScore * 100) + "%");
if (!survivingMutations.isEmpty()) {
System.out.println("\nSurviving Mutations:");
survivingMutations.forEach(mutation ->
System.out.println(mutation.getDescription()));
}
}
}
Testing Strategies
Effective mutation testing requires a well-thought-out strategy that combines different testing approaches. This section explores various testing strategies that work well with mutation testing, including unit testing, integration testing, and test-driven development. Learn how to implement these strategies to maximize the benefits of mutation testing.
Testing Strategy Implementation
public class OrderServiceTest {
private OrderService orderService;
private OrderRepository orderRepository;
@BeforeEach
void setUp() {
orderRepository = mock(OrderRepository.class);
orderService = new OrderService(orderRepository);
}
@Test
void shouldProcessValidOrder() {
// Arrange
Order order = new Order(100.0, "USD");
when(orderRepository.save(any())).thenReturn(order);
// Act
OrderResult result = orderService.processOrder(order);
// Assert
assertEquals("SUCCESS", result.getStatus());
verify(orderRepository).save(order);
}
@Test
void shouldHandleInvalidOrder() {
// Arrange
Order order = new Order(-100.0, "USD");
// Act
OrderResult result = orderService.processOrder(order);
// Assert
assertEquals("FAILED", result.getStatus());
verify(orderRepository, never()).save(any());
}
@Test
void shouldHandleRepositoryException() {
// Arrange
Order order = new Order(100.0, "USD");
when(orderRepository.save(any())).thenThrow(new RuntimeException("DB Error"));
// Act
OrderResult result = orderService.processOrder(order);
// Assert
assertEquals("ERROR", result.getStatus());
assertTrue(result.getError().contains("DB Error"));
}
}
Best Practices
To get the most out of mutation testing, it's important to follow established best practices. This section covers essential guidelines for implementing mutation testing effectively, from initial setup to continuous integration. These practices help ensure that your mutation testing efforts yield meaningful results and contribute to code quality improvement.
Mutation Testing Best Practices
- Start with high code coverage
- Use appropriate mutation operators
- Focus on critical code paths
- Regularly update test suite
- Analyze surviving mutations
- Use continuous integration
- Document test results
- Review and refactor tests
Conclusion
Mutation testing provides a powerful way to evaluate the quality of your test suite beyond simple code coverage metrics. By understanding and implementing these techniques, developers can create more robust and reliable tests.