IoT Development with Java (2025)
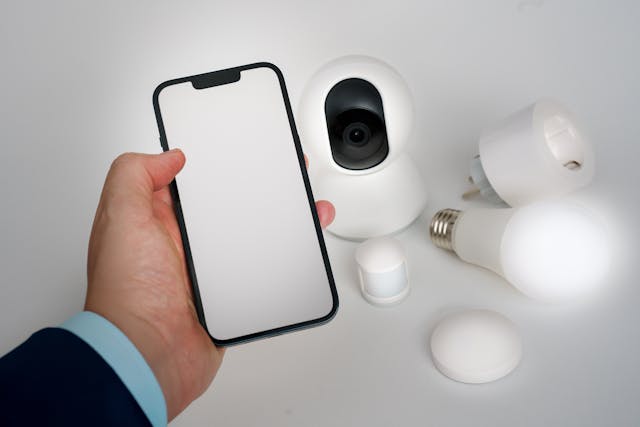
Java provides powerful capabilities for building IoT applications. This guide explores device management, data collection, and integration patterns for IoT applications.
Pro Tip: Modern IoT frameworks and protocols make it easier to build connected device applications.
Table of Contents
Device Management
Note: Effective device management is crucial for IoT applications.
Device Model
@Entity
public class IoTDevice {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String deviceId;
private String name;
private String type;
private String status;
private String firmwareVersion;
private LocalDateTime lastSeen;
private Map properties;
@OneToMany(mappedBy = "device", cascade = CascadeType.ALL)
private List telemetryData;
// Getters and setters
}
Device Service
@Service
public class DeviceManagementService {
private final DeviceRepository deviceRepository;
private final MqttClient mqttClient;
public DeviceManagementService(DeviceRepository deviceRepository, MqttClient mqttClient) {
this.deviceRepository = deviceRepository;
this.mqttClient = mqttClient;
}
public IoTDevice registerDevice(DeviceRegistrationRequest request) {
IoTDevice device = new IoTDevice();
device.setDeviceId(request.getDeviceId());
device.setName(request.getName());
device.setType(request.getType());
device.setStatus("OFFLINE");
device.setProperties(request.getProperties());
return deviceRepository.save(device);
}
public void updateDeviceStatus(String deviceId, String status) {
IoTDevice device = deviceRepository.findByDeviceId(deviceId)
.orElseThrow(() -> new DeviceNotFoundException(deviceId));
device.setStatus(status);
device.setLastSeen(LocalDateTime.now());
deviceRepository.save(device);
}
}
MQTT Integration
Pro Tip: MQTT is a lightweight protocol ideal for IoT communication.
MQTT Configuration
@Configuration
public class MqttConfig {
@Bean
public MqttClient mqttClient() throws MqttException {
MqttClient client = new MqttClient(
"tcp://broker.example.com:1883",
"java-iot-client-" + UUID.randomUUID()
);
MqttConnectOptions options = new MqttConnectOptions();
options.setAutomaticReconnect(true);
options.setCleanSession(true);
options.setConnectionTimeout(10);
client.connect(options);
return client;
}
@Bean
public MqttCallback mqttCallback(DeviceManagementService deviceService) {
return new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
// Handle connection loss
}
@Override
public void messageArrived(String topic, MqttMessage message) {
String payload = new String(message.getPayload());
String deviceId = topic.split("/")[1];
deviceService.processDeviceMessage(deviceId, payload);
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
// Handle message delivery
}
};
}
}
MQTT Message Handler
@Service
public class MqttMessageHandler {
private final MqttClient mqttClient;
private final ObjectMapper objectMapper;
public MqttMessageHandler(MqttClient mqttClient, ObjectMapper objectMapper) {
this.mqttClient = mqttClient;
this.objectMapper = objectMapper;
}
public void publishMessage(String topic, Object message) throws MqttException {
String payload = objectMapper.writeValueAsString(message);
MqttMessage mqttMessage = new MqttMessage(payload.getBytes());
mqttMessage.setQos(1);
mqttClient.publish(topic, mqttMessage);
}
public void subscribeToTopic(String topic, int qos) throws MqttException {
mqttClient.subscribe(topic, qos);
}
}
CoAP Protocol
Note: CoAP is designed for constrained devices and networks.
CoAP Server
@Configuration
public class CoapServerConfig {
@Bean
public CoapServer coapServer() {
CoapServer server = new CoapServer();
server.add(new CoapResource("sensors") {
@Override
public void handleGET(CoapExchange exchange) {
// Handle sensor data request
String response = getSensorData();
exchange.respond(response);
}
@Override
public void handlePOST(CoapExchange exchange) {
// Handle sensor data update
byte[] payload = exchange.getRequestPayload();
updateSensorData(payload);
exchange.respond(ResponseCode.CHANGED);
}
});
server.start();
return server;
}
}
Data Collection
Pro Tip: Efficient data collection and storage are essential for IoT applications.
Telemetry Service
@Service
public class TelemetryService {
private final TelemetryRepository telemetryRepository;
private final KafkaTemplate kafkaTemplate;
public TelemetryService(TelemetryRepository telemetryRepository, KafkaTemplate kafkaTemplate) {
this.telemetryRepository = telemetryRepository;
this.kafkaTemplate = kafkaTemplate;
}
public void processTelemetryData(String deviceId, Map data) {
DeviceTelemetry telemetry = new DeviceTelemetry();
telemetry.setDeviceId(deviceId);
telemetry.setTimestamp(LocalDateTime.now());
telemetry.setData(data);
telemetryRepository.save(telemetry);
// Publish to Kafka for real-time processing
kafkaTemplate.send("device-telemetry", deviceId,
new ObjectMapper().writeValueAsString(telemetry));
}
public List getDeviceTelemetry(String deviceId,
LocalDateTime startTime, LocalDateTime endTime) {
return telemetryRepository.findByDeviceIdAndTimestampBetween(
deviceId, startTime, endTime);
}
}
Best Practices
Note: Following IoT best practices ensures reliable and scalable applications.
IoT Best Practices
- Implement proper device authentication
- Use secure communication protocols
- Implement proper error handling
- Use proper data validation
- Enable proper monitoring
- Implement proper testing
- Use proper documentation
- Enable proper logging
- Implement proper deployment
- Use proper versioning
- Enable proper backup
- Implement proper recovery
- Use proper scaling
- Follow IoT guidelines
- Implement proper security
Conclusion
Java provides powerful capabilities for building IoT applications. By understanding and utilizing these tools effectively, you can create sophisticated connected device applications that leverage the benefits of the Internet of Things.