Java Code Coverage: Complete Guide with EclEmma (2025)
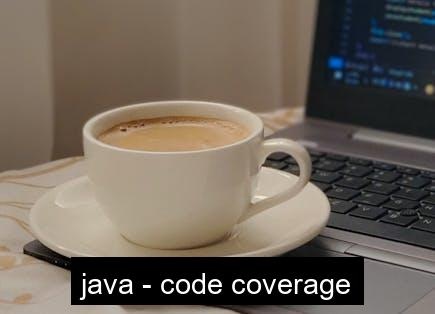
Code coverage is a critical metric in software development that measures how much of your code is tested. This comprehensive guide explores code coverage in Java, focusing on the powerful EclEmma plugin for Eclipse and other essential tools and practices.
Table of Contents
1. Introduction to Code Coverage
Code coverage is a measure used to describe the degree to which the source code of a program is executed when a particular test suite runs. In Java development, understanding and maintaining good code coverage is essential for:
- Identifying untested code paths
- Ensuring test quality
- Maintaining code reliability
- Supporting refactoring efforts
- Meeting quality standards
2. Code Coverage Metrics
Understanding different types of code coverage metrics is crucial for comprehensive testing. Code coverage metrics help developers and teams assess the quality and completeness of their test suites. Let's explore the main types of coverage metrics:
2.1 Line Coverage
Line coverage, also known as statement coverage, measures the percentage of code lines executed during testing. This is the most basic form of coverage measurement and provides a good starting point for assessing test coverage.
public class UserService {
public User createUser(String name, String email) {
// Line 1: Start of method
if (name == null || email == null) {
// Line 2: Validation check
throw new IllegalArgumentException("Name and email cannot be null");
}
// Line 3: Create user
User user = new User(name, email);
// Line 4: Save user
return userRepository.save(user);
}
}
2.2 Branch Coverage
Branch coverage, also called decision coverage, measures the percentage of code branches executed during testing. This metric is more thorough than line coverage as it ensures that both true and false conditions in control structures are tested.
public class PaymentProcessor {
public void processPayment(Payment payment) {
if (payment.getAmount() > 1000) {
// Branch 1: High-value payment
applyHighValueDiscount(payment);
} else if (payment.getAmount() < 100) {
// Branch 2: Low-value payment
applyLowValueFee(payment);
} else {
// Branch 3: Standard payment
processStandardPayment(payment);
}
}
}
2.3 Method Coverage
Method coverage measures the percentage of methods that have been called during test execution. This metric helps identify untested methods in your codebase.
2.4 Class Coverage
Class coverage measures the percentage of classes that have been instantiated during test execution. This is particularly useful for identifying completely untested classes.
3. Industry Standards for Code Coverage
Understanding industry standards for code coverage helps teams set realistic goals and maintain consistent quality across projects. Here are the generally accepted standards:
3.1 Minimum Coverage Requirements
- Critical Systems: 90-100% coverage
- Enterprise Applications: 80-90% coverage
- Web Applications: 70-80% coverage
- Legacy Systems: 50-70% coverage
3.2 Coverage by Component Type
- Core Business Logic: 90%+ coverage
- API Endpoints: 85%+ coverage
- Utility Classes: 80%+ coverage
- UI Components: 70%+ coverage
4. EclEmma Plugin for Eclipse
EclEmma is a powerful code coverage tool for Eclipse that provides real-time code coverage analysis. Let's explore how to set up and use EclEmma effectively.
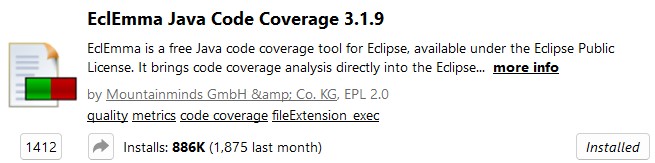
4.1 Installing EclEmma
1. Open Eclipse
2. Go to Help → Eclipse Marketplace
3. Search for "EclEmma"
4. Click "Install" on EclEmma
5. Accept the license agreement
6. Restart Eclipse
4.2 Using EclEmma
EclEmma provides different coverage modes and real-time analysis. Here's an example of how to use it:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
public int multiply(int a, int b) {
return a * b;
}
public double divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("Division by zero");
}
return (double) a / b;
}
}
5. JaCoCo Coverage Tool
JaCoCo is a popular code coverage library that can be integrated with various build tools. It provides detailed coverage reports and can be used in both local development and CI/CD pipelines.
5.1 Maven Integration
Add the following configuration to your pom.xml file:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
</executions>
</plugin>
6. Best Practices
Follow these best practices to maintain high-quality code coverage:
- Write meaningful tests that cover business logic
- Focus on critical paths and edge cases
- Use test-driven development (TDD) when possible
- Regularly review and update test coverage
- Set realistic coverage goals
- Include both positive and negative test cases
- Test exception handling paths
- Use mocking for external dependencies
7. CI/CD Integration
Integrating code coverage into your CI/CD pipeline ensures consistent quality:
7.1 Jenkins Integration
pipeline {
agent any
stages {
stage('Build and Test') {
steps {
sh 'mvn clean verify'
jacoco(
execPattern: '**/target/coverage.exec',
classPattern: '**/target/classes',
sourcePattern: '**/src/main/java',
exclusionPattern: '**/test/**'
)
}
}
}
}
Conclusion
Code coverage is an essential aspect of software quality assurance. By using tools like EclEmma and following best practices, you can maintain high-quality code and ensure your applications are well-tested. Remember that while high coverage is important, it's equally crucial to focus on the quality and relevance of your tests.