Java and AI: Building Intelligent Applications (2025)
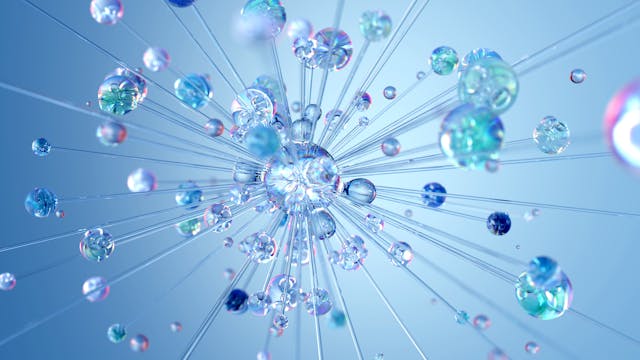
Java provides powerful capabilities for building intelligent applications with AI. This guide explores modern AI integration patterns and techniques for Java applications.
Table of Contents
Machine Learning Integration
Machine learning integration in Java applications enables developers to build intelligent systems that can learn from data and make predictions. This section explores how to integrate machine learning capabilities using popular libraries like DL4J (Deep Learning for Java), which provides a comprehensive set of tools for implementing neural networks and other machine learning algorithms. We'll cover configuration, model training, and practical implementation patterns.
DL4J Configuration
@Configuration
public class DL4JConfig {
@Bean
public MultiLayerNetwork neuralNetwork() {
MultiLayerConfiguration conf = new NeuralNetConfiguration.Builder()
.seed(123)
.optimizationAlgo(OptimizationAlgorithm.STOCHASTIC_GRADIENT_DESCENT)
.iterations(1)
.learningRate(0.01)
.list()
.layer(0, new DenseLayer.Builder()
.nIn(784)
.nOut(250)
.activation(Activation.RELU)
.build())
.layer(1, new OutputLayer.Builder()
.nIn(250)
.nOut(10)
.activation(Activation.SOFTMAX)
.lossFunction(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD)
.build())
.pretrain(false)
.backprop(true)
.build();
return new MultiLayerNetwork(conf);
}
}
Model Training
@Service
public class ModelTrainingService {
private final MultiLayerNetwork network;
public ModelTrainingService(MultiLayerNetwork network) {
this.network = network;
}
public void trainModel(DataSet trainingData) {
network.init();
for (int i = 0; i < 100; i++) {
network.fit(trainingData);
System.out.println("Epoch " + i + " completed");
}
}
public double evaluate(DataSet testData) {
Evaluation eval = new Evaluation(10);
INDArray output = network.output(testData.getFeatures());
eval.eval(testData.getLabels(), output);
return eval.accuracy();
}
}
Natural Language Processing
Natural Language Processing (NLP) capabilities in Java enable applications to understand, analyze, and generate human language. This section demonstrates how to implement NLP features using Apache OpenNLP, a powerful library that provides tools for text tokenization, part-of-speech tagging, named entity recognition, and more. We'll explore practical examples of text analysis and language processing.
OpenNLP Integration
@Service
public class NLPService {
private final Tokenizer tokenizer;
private final POSTagger tagger;
private final NameFinder nameFinder;
public NLPService() throws IOException {
// Load models
InputStream tokenModelIn = getClass().getResourceAsStream("/models/en-token.bin");
InputStream posModelIn = getClass().getResourceAsStream("/models/en-pos-maxent.bin");
InputStream nameModelIn = getClass().getResourceAsStream("/models/en-ner-person.bin");
TokenizerModel tokenModel = new TokenizerModel(tokenModelIn);
POSModel posModel = new POSModel(posModelIn);
TokenNameFinderModel nameModel = new TokenNameFinderModel(nameModelIn);
this.tokenizer = new TokenizerME(tokenModel);
this.tagger = new POSTaggerME(posModel);
this.nameFinder = new NameFinderME(nameModel);
}
public List tokenize(String text) {
return Arrays.asList(tokenizer.tokenize(text));
}
public List tagPOS(String text) {
String[] tokens = tokenizer.tokenize(text);
return Arrays.asList(tagger.tag(tokens));
}
public List findNames(String text) {
String[] tokens = tokenizer.tokenize(text);
return Arrays.asList(nameFinder.find(tokens));
}
}
Deep Learning
Deep learning represents a subset of machine learning that uses multiple layers of neural networks to learn complex patterns in data. This section covers how to implement deep learning models in Java applications using DL4J's advanced features. We'll explore LSTM networks, convolutional neural networks, and other deep learning architectures for tasks like sequence prediction and pattern recognition.
Deep Learning Model
@Service
public class DeepLearningService {
private final ComputationGraph network;
public DeepLearningService() {
ComputationGraphConfiguration conf = new NeuralNetConfiguration.Builder()
.seed(123)
.optimizationAlgo(OptimizationAlgorithm.STOCHASTIC_GRADIENT_DESCENT)
.iterations(1)
.learningRate(0.01)
.graphBuilder()
.addInputs("input")
.setOutputs("output")
.addLayer("lstm1", new LSTM.Builder()
.nIn(100)
.nOut(200)
.activation(Activation.TANH)
.build(), "input")
.addLayer("lstm2", new LSTM.Builder()
.nIn(200)
.nOut(100)
.activation(Activation.TANH)
.build(), "lstm1")
.addLayer("output", new RnnOutputLayer.Builder()
.nIn(100)
.nOut(10)
.activation(Activation.SOFTMAX)
.lossFunction(LossFunctions.LossFunction.MCXENT)
.build(), "lstm2")
.build();
this.network = new ComputationGraph(conf);
this.network.init();
}
public INDArray predict(INDArray input) {
return network.outputSingle(input);
}
}
AI Services Integration
Cloud-based AI services provide powerful capabilities without requiring complex local implementation. This section explores how to integrate popular AI services like AWS Comprehend into Java applications. We'll cover authentication, API integration, and practical examples of using cloud AI services for tasks like sentiment analysis, entity detection, and text classification.
AWS AI Integration
@Service
public class AWSComprehendService {
private final AmazonComprehend comprehendClient;
public AWSComprehendService() {
this.comprehendClient = AmazonComprehendClientBuilder.standard()
.withRegion(Regions.US_EAST_1)
.build();
}
public List detectEntities(String text) {
DetectEntitiesRequest request = new DetectEntitiesRequest()
.withText(text)
.withLanguageCode("en");
DetectEntitiesResult result = comprehendClient.detectEntities(request);
return result.getEntities().stream()
.map(Entity::getText)
.collect(Collectors.toList());
}
public Map detectSentiment(String text) {
DetectSentimentRequest request = new DetectSentimentRequest()
.withText(text)
.withLanguageCode("en");
DetectSentimentResult result = comprehendClient.detectSentiment(request);
return Map.of(
"positive", result.getSentimentScore().getPositive(),
"negative", result.getSentimentScore().getNegative(),
"neutral", result.getSentimentScore().getNeutral(),
"mixed", result.getSentimentScore().getMixed()
);
}
}
Best Practices
Implementing AI features in Java applications requires careful consideration of various factors to ensure reliability, performance, and maintainability. This section covers essential best practices for AI integration, including data preprocessing, model validation, error handling, security considerations, and deployment strategies. Following these guidelines helps create robust and efficient AI-powered applications.
AI Best Practices
- Choose appropriate AI tools
- Implement proper data preprocessing
- Use proper model validation
- Implement proper error handling
- Use proper model versioning
- Enable proper monitoring
- Implement proper security
- Use proper data privacy
- Enable proper scaling
- Implement proper testing
- Use proper documentation
- Enable proper logging
- Implement proper deployment
- Use proper optimization
- Follow AI guidelines
Conclusion
Java provides powerful capabilities for building intelligent applications with AI. By understanding and utilizing these tools effectively, you can create sophisticated AI-powered applications that solve complex problems.