Java in the Cloud: A Complete Guide to Cloud-Native Development (2025)
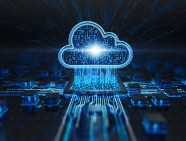
Cloud-native development has revolutionized how we build and deploy Java applications. This comprehensive guide explores modern cloud-native practices, from containerization to serverless computing, helping you build scalable and resilient applications in the cloud.
Pro Tip: Cloud-native development requires a shift in mindset, focusing on scalability, resilience, and automation from the start.
Table of Contents
Containerization with Docker
Note: Containerization is the foundation of cloud-native development, providing consistency and portability across environments.
Dockerfile Example
# Use multi-stage build for smaller final image
FROM eclipse-temurin:17-jdk-alpine AS builder
WORKDIR /app
COPY . .
RUN ./mvnw clean package -DskipTests
FROM eclipse-temurin:17-jre-alpine
WORKDIR /app
COPY --from=builder /app/target/*.jar app.jar
# Add non-root user for security
RUN addgroup -S spring && adduser -S spring -G spring
USER spring
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "app.jar"]
Docker Compose Configuration
version: '3.8'
services:
app:
build: .
ports:
- "8080:8080"
environment:
- SPRING_PROFILES_ACTIVE=prod
- DB_HOST=db
depends_on:
- db
healthcheck:
test: ["CMD", "curl", "-f", "http://localhost:8080/actuator/health"]
interval: 30s
timeout: 10s
retries: 3
db:
image: postgres:14-alpine
environment:
- POSTGRES_DB=myapp
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
Kubernetes Orchestration
Pro Tip: Kubernetes provides powerful orchestration capabilities for managing containerized applications at scale.
Kubernetes Deployment Example
apiVersion: apps/v1
kind: Deployment
metadata:
name: java-app
spec:
replicas: 3
selector:
matchLabels:
app: java-app
template:
metadata:
labels:
app: java-app
spec:
containers:
- name: java-app
image: myapp:latest
ports:
- containerPort: 8080
resources:
requests:
memory: "512Mi"
cpu: "200m"
limits:
memory: "1Gi"
cpu: "500m"
livenessProbe:
httpGet:
path: /actuator/health/liveness
port: 8080
initialDelaySeconds: 60
periodSeconds: 10
readinessProbe:
httpGet:
path: /actuator/health/readiness
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
---
apiVersion: v1
kind: Service
metadata:
name: java-app-service
spec:
selector:
app: java-app
ports:
- port: 80
targetPort: 8080
type: LoadBalancer
Cloud Platforms
Note: Different cloud platforms offer unique features and services for Java applications.
AWS Integration Example
@Configuration
public class AwsConfig {
@Bean
public AmazonS3 amazonS3Client() {
return AmazonS3ClientBuilder.standard()
.withRegion(Regions.US_EAST_1)
.withCredentials(new DefaultAWSCredentialsProviderChain())
.build();
}
@Bean
public AmazonDynamoDB amazonDynamoDBClient() {
return AmazonDynamoDBClientBuilder.standard()
.withRegion(Regions.US_EAST_1)
.withCredentials(new DefaultAWSCredentialsProviderChain())
.build();
}
}
@Service
public class S3Service {
private final AmazonS3 s3Client;
public S3Service(AmazonS3 s3Client) {
this.s3Client = s3Client;
}
public void uploadFile(String bucketName, String key, File file) {
s3Client.putObject(new PutObjectRequest(bucketName, key, file));
}
public S3Object downloadFile(String bucketName, String key) {
return s3Client.getObject(new GetObjectRequest(bucketName, key));
}
}
Spring Cloud Integration
Pro Tip: Spring Cloud provides powerful tools for building cloud-native applications.
Spring Cloud Configuration Example
@SpringBootApplication
@EnableDiscoveryClient
public class CloudApplication {
public static void main(String[] args) {
SpringApplication.run(CloudApplication.class, args);
}
}
@Configuration
public class CloudConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
@Bean
public LoadBalancerClient loadBalancerClient() {
return new RibbonLoadBalancerClient();
}
}
@Service
public class CloudService {
private final RestTemplate restTemplate;
private final LoadBalancerClient loadBalancer;
public CloudService(RestTemplate restTemplate, LoadBalancerClient loadBalancer) {
this.restTemplate = restTemplate;
this.loadBalancer = loadBalancer;
}
public String callService(String serviceId, String path) {
ServiceInstance instance = loadBalancer.choose(serviceId);
String url = instance.getUri().toString() + path;
return restTemplate.getForObject(url, String.class);
}
}
Serverless Computing
Note: Serverless computing offers a new paradigm for running Java applications without managing servers.
AWS Lambda Example
public class LambdaHandler implements RequestHandler {
private final ObjectMapper objectMapper;
public LambdaHandler() {
this.objectMapper = new ObjectMapper();
}
@Override
public APIGatewayProxyResponseEvent handleRequest(
APIGatewayProxyRequestEvent input, Context context) {
try {
// Process request
String body = input.getBody();
UserRequest userRequest = objectMapper.readValue(body, UserRequest.class);
// Business logic
UserResponse response = processUserRequest(userRequest);
// Return response
return APIGatewayProxyResponseEvent.builder()
.statusCode(200)
.body(objectMapper.writeValueAsString(response))
.build();
} catch (Exception e) {
context.getLogger().log("Error: " + e.getMessage());
return APIGatewayProxyResponseEvent.builder()
.statusCode(500)
.body("{\"error\": \"" + e.getMessage() + "\"}")
.build();
}
}
}
Cloud-Native Patterns
Pro Tip: Understanding cloud-native patterns helps build resilient and scalable applications.
Circuit Breaker Pattern Example
@Configuration
public class CircuitBreakerConfig {
@Bean
public CircuitBreaker circuitBreaker() {
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.permittedNumberOfCallsInHalfOpenState(3)
.slidingWindowSize(2)
.build();
return CircuitBreaker.of("serviceName", config);
}
}
@Service
public class ResilientService {
private final CircuitBreaker circuitBreaker;
public ResilientService(CircuitBreaker circuitBreaker) {
this.circuitBreaker = circuitBreaker;
}
public String callExternalService() {
return circuitBreaker.executeSupplier(() -> {
// Call external service
return externalServiceClient.call();
});
}
}
Best Practices
Note: Following cloud-native best practices ensures successful deployment and operation of applications.
Key Best Practices
- Use containerization for consistent environments
- Implement proper health checks and monitoring
- Use configuration management for environment-specific settings
- Implement proper logging and tracing
- Use service mesh for microservices communication
- Implement proper security measures
- Use cloud-native databases and caching
- Implement proper CI/CD pipelines
Conclusion
Cloud-native development with Java offers powerful capabilities for building scalable and resilient applications. By understanding and implementing containerization, orchestration, and cloud-native patterns, you can create applications that thrive in modern cloud environments.