Java Build Tools: Beyond Maven and Gradle (2025)
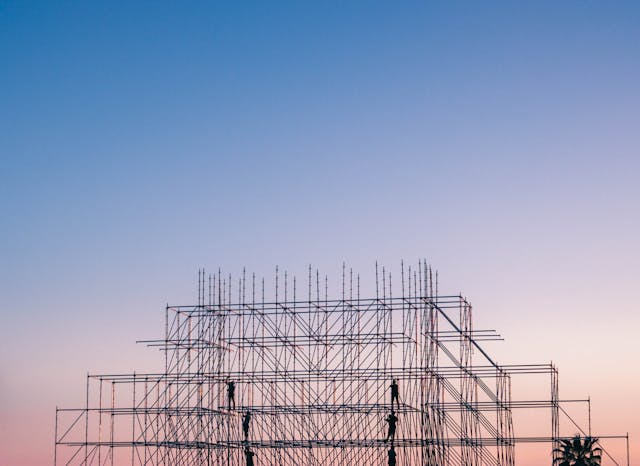
Modern Java development requires efficient build tools beyond traditional Maven and Gradle. This comprehensive guide explores advanced build tools and techniques for modern Java applications.
Table of Contents
1. Bazel
Bazel is Google's open-source build system that provides fast, reliable, and reproducible builds for multi-language projects. This section explores how to use Bazel for Java development, covering workspace configuration, build rules, and advanced features. We'll learn how to set up Java projects, manage dependencies, and create custom build rules for specific requirements.
Bazel Configuration
# WORKSPACE file
workspace(name = "my_java_project")
load("@bazel_tools//tools/build_defs/repo:http.bzl", "http_archive")
http_archive(
name = "rules_java",
url = "https://github.com/bazelbuild/rules_java/releases/download/5.5.0/rules_java-5.5.0.tar.gz",
sha256 = "b8b6e5c1c0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0c0f0",
)
load("@rules_java//java:repositories.bzl", "rules_java_deps", "rules_java_toolchains")
rules_java_deps()
rules_java_toolchains()
# BUILD file
java_binary(
name = "my_app",
srcs = glob(["src/main/java/**/*.java"]),
main_class = "com.example.MyApp",
deps = [
"@maven//:org_junit_junit",
],
)
Bazel Build Rules
# Custom build rule
def _java_custom_rule_impl(ctx):
output = ctx.actions.declare_file(ctx.label.name + ".jar")
ctx.actions.run(
inputs = ctx.files.srcs,
outputs = [output],
executable = ctx.executable._tool,
arguments = [
"--input", ",".join([f.path for f in ctx.files.srcs]),
"--output", output.path,
],
)
return [DefaultInfo(files = depset([output]))]
java_custom_rule = rule(
implementation = _java_custom_rule_impl,
attrs = {
"srcs": attr.label_list(allow_files = True),
"_tool": attr.label(
executable = True,
cfg = "host",
default = Label("//tools:custom_tool"),
),
},
)
2. SBT (Scala Build Tool)
While primarily known for Scala development, SBT is also a powerful build tool for Java projects. This section demonstrates how to leverage SBT's features for Java development, including dependency management, custom tasks, and plugin integration. We'll explore SBT's declarative build configuration and its ability to handle complex build scenarios.
SBT Configuration
// build.sbt
name := "my-java-project"
version := "1.0"
scalaVersion := "2.13.8"
libraryDependencies ++= Seq(
"org.junit.jupiter" % "junit-jupiter" % "5.8.2" % Test,
"org.mockito" % "mockito-core" % "4.5.1" % Test
)
// Custom task
lazy val customTask = taskKey[Unit]("Custom build task")
customTask := {
println("Running custom task...")
// Task implementation
}
// Custom setting
lazy val customSetting = settingKey[String]("Custom build setting")
customSetting := "default value"
SBT Plugins
// project/plugins.sbt
addSbtPlugin("com.github.sbt" % "sbt-native-packager" % "1.9.7")
addSbtPlugin("com.github.sbt" % "sbt-jacoco" % "3.4.0")
// Custom plugin
object CustomPlugin extends AutoPlugin {
override def requires = plugins.JvmPlugin
override def trigger = allRequirements
object autoImport {
val customTask = taskKey[Unit]("Custom plugin task")
}
import autoImport._
override def projectSettings = Seq(
customTask := {
println("Running custom plugin task...")
// Task implementation
}
)
}
3. Apache Ant
Apache Ant is a flexible and extensible build automation tool that has been a mainstay in Java development for many years. This section covers how to use Ant for modern Java projects, including build file creation, task definitions, and integration with other tools. We'll explore Ant's XML-based configuration and its ability to handle complex build processes.
Ant Build File
Custom Ant Task
public class CustomTask extends Task {
private String input;
private String output;
public void setInput(String input) {
this.input = input;
}
public void setOutput(String output) {
this.output = output;
}
@Override
public void execute() throws BuildException {
try {
// Task implementation
log("Processing input: " + input);
log("Generating output: " + output);
} catch (Exception e) {
throw new BuildException("Task failed", e);
}
}
}
4. Buildr
Buildr is a modern build system that combines the power of Ruby with Java development capabilities. This section explores how to use Buildr for Java projects, including project definition, dependency management, and custom task creation. We'll learn how to leverage Buildr's Ruby-based DSL for flexible and maintainable build configurations.
Buildr Configuration
# buildfile
define 'my-java-project' do
project.version = '1.0'
compile.with(
'org.junit:junit:jar:4.13.2',
'org.mockito:mockito-core:jar:4.5.1'
)
test.with(
'org.junit:junit:jar:4.13.2',
'org.mockito:mockito-core:jar:4.5.1'
)
package(:jar).with :manifest => {
'Main-Class' => 'com.example.MyApp'
}
# Custom task
task 'custom_task' do
puts "Running custom task..."
# Task implementation
end
end
Buildr Extension
# lib/custom_extension.rb
module Buildr
module CustomExtension
include Extension
first_time do
desc 'Run custom task'
Project.local_task('custom_task')
end
before_define do |project|
project.recursive_task('custom_task') do
puts "Running custom task for #{project.name}"
# Task implementation
end
end
end
end
class Buildr::Project
include Buildr::CustomExtension
end
5. Best Practices
Effective use of build tools requires understanding and following established best practices. This section covers essential guidelines for build tool usage, including configuration management, performance optimization, and integration with CI/CD pipelines. Following these practices helps create maintainable and efficient build processes.
Build Tool Best Practices
- Use appropriate build tool for project needs
- Maintain clear build configuration
- Optimize build performance
- Manage dependencies effectively
- Use proper versioning
- Implement proper testing
- Use proper security
- Enable proper logging
- Use proper documentation
- Implement proper CI/CD
- Use proper caching
- Enable proper monitoring
- Use proper error handling
- Implement proper validation
- Follow build guidelines
Conclusion
Modern Java build tools provide powerful capabilities for efficient development. By understanding and utilizing these tools effectively, you can streamline your build process and improve development productivity.