Java 24 Features: A Comprehensive Guide for Advanced Developers
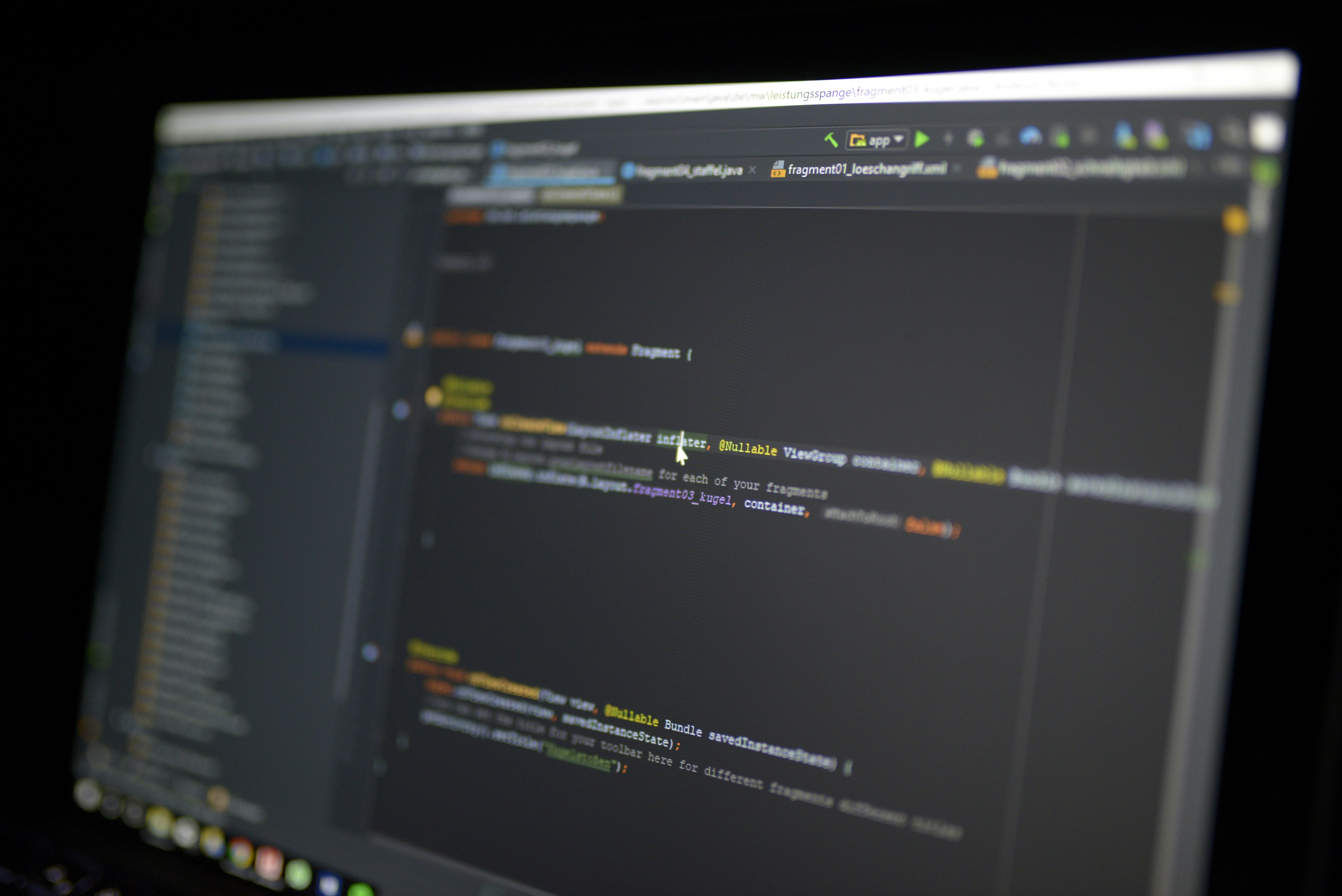
Java 24 brings significant enhancements and new features that advance the language's capabilities for modern application development. In this comprehensive guide, we'll explore the major features introduced in Java 24 with practical code examples for advanced developers.
Table of Contents
1. Scoped Values
Scoped Values is a new feature in Java 24 that provides a more efficient way to share data between methods without using method parameters or global variables. It's particularly useful for passing context information through the call stack.
// Example of using Scoped Values
public class ScopedValueExample {
private static final ScopedValue USER_CONTEXT = ScopedValue.newInstance();
public void processRequest() {
ScopedValue.where(USER_CONTEXT, "John Doe").run(() -> {
handleUserRequest();
});
}
private void handleUserRequest() {
String user = USER_CONTEXT.get();
// Use the user context
}
}
2. Record Patterns
Record Patterns enhance pattern matching capabilities by allowing you to deconstruct record values directly in pattern matching expressions. This feature makes working with records more convenient and expressive.
// Example of Record Patterns
record Point(int x, int y) {}
public class RecordPatternExample {
public String describePoint(Object obj) {
return switch (obj) {
case Point(int x, int y) when x == y -> "Point on diagonal";
case Point(int x, int y) -> "Point at (" + x + ", " + y + ")";
default -> "Not a point";
};
}
}
3. Pattern Matching for Switch Statements
Pattern matching for switch statements has been enhanced in Java 24, allowing for more sophisticated pattern matching capabilities in switch expressions and statements.
// Example of Enhanced Pattern Matching in Switch
public class SwitchPatternExample {
public String processShape(Object shape) {
return switch (shape) {
case Circle c -> "Circle with radius " + c.radius();
case Rectangle r -> "Rectangle with width " + r.width() + " and height " + r.height();
case null -> "Null shape";
default -> "Unknown shape";
};
}
}
4. Foreign Function & Memory API
The Foreign Function & Memory API has been updated in Java 24 to provide better performance and more capabilities for interacting with native code and memory.
// Example of Foreign Function & Memory API
import java.lang.foreign.*;
public class ForeignMemoryExample {
public void memoryExample() {
try (Arena arena = Arena.ofConfined()) {
MemorySegment segment = arena.allocate(100);
// Use the memory segment
}
}
}
Frequently Asked Questions
What are the main benefits of Scoped Values?
Scoped Values provide several benefits:
- Efficient context passing through the call stack
- Thread-safe by design
- No need for method parameter changes
- Better performance than ThreadLocal
How do Record Patterns improve code readability?
Record Patterns improve code readability by:
- Simplifying record value extraction
- Making pattern matching more intuitive
- Reducing boilerplate code
- Enabling more expressive control flow
What's new in Pattern Matching for Switch?
Java 24 enhances pattern matching in switch by:
- Supporting more complex patterns
- Improving type inference
- Better null handling
- More efficient pattern matching
How does the Foreign Function & Memory API help developers?
The Foreign Function & Memory API provides:
- Direct access to native memory
- Better performance for native code interaction
- Improved safety and security
- Simplified native code integration
Conclusion
Java 24 introduces several powerful features that enhance the language's capabilities for modern application development. Scoped Values, Record Patterns, enhanced Pattern Matching, and the updated Foreign Function & Memory API provide developers with new tools for building efficient and maintainable applications.
These features demonstrate Java's continued evolution as a modern programming language while maintaining its core principles of reliability, security, and performance.
Comparison with Previous Java Versions
Feature | Java 24 | Previous Versions | Benefits |
---|---|---|---|
Pattern Matching | Enhanced switch expressions and record patterns | Basic pattern matching in instanceof (Java 16) | More concise code, better type safety |
Thread-Local Data | ScopedValue API | ThreadLocal | Better memory management, thread safety |
Native Interop | Enhanced Foreign Function & Memory API | JNI, Panama Foreign API (Preview) | Safer native access, better performance |
Migration Guide
1. Preparing for Migration
- Update your build tools and CI/CD pipelines to support Java 24
- Review deprecated features and their replacements
- Test your application with Java 24 in a staging environment
- Update your dependencies to versions compatible with Java 24
2. Code Updates
// Old code using ThreadLocal
private static final ThreadLocal<String> transactionId =
ThreadLocal.withInitial(() -> "");
// New code using ScopedValue
private static final ScopedValue<String> TRANSACTION_ID =
ScopedValue.newInstance();
// Old pattern matching
if (obj instanceof Rectangle) {
Rectangle rect = (Rectangle) obj;
System.out.println(rect.width() * rect.height());
}
// New pattern matching
if (obj instanceof Rectangle(double w, double h)) {
System.out.println(w * h);
}
3. Performance Optimization Tips
- Profile your application to identify potential bottlenecks
- Use the new Foreign Memory API for large off-heap allocations
- Replace ThreadLocal usage with ScopedValue where appropriate
- Leverage pattern matching for more efficient type checking
4. Testing Strategy
- Update test frameworks to support Java 24
- Add tests for new language features
- Verify thread safety with ScopedValue
- Test native interop performance
Frequently Asked Questions About Java 24
What are the major improvements in Java 24 compared to Java 21?
Java 24 introduces several significant improvements over Java 21, including:
- Enhanced switch pattern matching with more sophisticated capabilities
- Scoped Values as a better alternative to ThreadLocal
- Improved Foreign Function & Memory API for native code integration
What are the benefits of using Scoped Values over ThreadLocal?
Scoped Values offer several advantages over ThreadLocal:
- Immutable values that prevent accidental modifications
- Automatic cleanup without explicit remove() calls
- Better integration with structured concurrency
- Improved performance through optimized implementation
- Thread safety by design
Can Java 24's Foreign Function & Memory API replace JNI?
While the Foreign Function & Memory API in Java 24 can replace many use cases of JNI (Java Native Interface), it's not a complete replacement. The API provides a safer, more efficient way to interact with native code and manage off-heap memory, but some legacy systems may still require JNI. The main advantages include type safety, better performance, and reduced risk of memory leaks.