OpenJDK 8 Code Samples
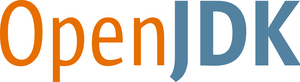
Try - With - Resource: Example 1
import java.io.BufferedOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
/**
* This sample demonstrates the ability to create custom resource that
* implements the {@code AutoCloseable} interface. This resource can be used in
* the try-with-resources construct.
*/
public class CustomAutoCloseableSample {
/**
* The main method for the CustomAutoCloseableSample program.
*
* @param args is not used.
*/
public static void main(String[] args) {
/*
* TeeStream will be closed automatically after the try block.
*/
try (TeeStream teeStream = new TeeStream(System.out, Paths.get("out.txt"));
PrintStream out = new PrintStream(teeStream)) {
out.print("Hello, world");
} catch (Exception e) {
e.printStackTrace();
System.exit(1);
}
}
/**
* Passes the output through to the specified output stream while copying it into a file.
* The TeeStream functionality is similar to the Unix tee utility.
* TeeStream implements AutoCloseable interface. See OutputStream for details.
*/
public static class TeeStream extends OutputStream {
private final OutputStream fileStream;
private final OutputStream outputStream;
/**
* Creates a TeeStream.
*
* @param outputStream an output stream.
* @param outputFile an path to file.
* @throws IOException If an I/O error occurs.
*/
public TeeStream(OutputStream outputStream, Path outputFile) throws IOException {
this.fileStream = new BufferedOutputStream(Files.newOutputStream(outputFile));
this.outputStream = outputStream;
}
/**
* Writes the specified byte to the specified output stream
* and copies it to the file.
*
* @param b the byte to be written.
* @throws IOException If an I/O error occurs.
*/
@Override
public void write(int b) throws IOException {
fileStream.write(b);
outputStream.write(b);
}
/**
* Flushes this output stream and forces any buffered output bytes
* to be written out.
* The flush
method of TeeStream
flushes
* the specified output stream and the file output stream.
*
* @throws IOException if an I/O error occurs.
*/
@Override
public void flush() throws IOException {
outputStream.flush();
fileStream.flush();
}
/**
* Closes underlying streams and resources.
* The external output stream won't be closed.
* This method is the member of AutoCloseable interface and
* it will be invoked automatically after the try-with-resources block.
*
* @throws IOException If an I/O error occurs.
*/
@Override
public void close() throws IOException {
try (OutputStream file = fileStream) {
flush();
}
}
}
}
Try - With - Resource : Example 2
/**
* Extract (unzip) a file to the current directory.
*/
public class Unzip {
/**
* The main method for the Unzip program. Run the program with an empty
* argument list to see possible arguments.
*
* @param args the argument list for {@code Unzip}.
*/
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Usage: Unzip zipfile");
}
final Path destDir = Paths.get(".");
/*
* Create AutoCloseable FileSystem. It will be closed automatically
* after the try block.
*/
try (FileSystem zipFileSystem = FileSystems.newFileSystem(Paths.get(args[0]), null)) {
Path top = zipFileSystem.getPath("/");
Files.walk(top).skip(1).forEach(file -> {
Path target = destDir.resolve(top.relativize(file).toString());
System.out.println("Extracting " + target);
try {
Files.copy(file, target, REPLACE_EXISTING);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
});
} catch (UncheckedIOException | IOException e) {
e.printStackTrace();
System.exit(1);
}
}
}
Try - With - Resource : Example 3
/**
* Prints data of the specified file to standard output from a zip archive.
*/
public class ZipCat {
/**
* The main method for the ZipCat program. Run the program with an empty
* argument list to see possible arguments.
*
* @param args the argument list for ZipCat
*/
public static void main(String[] args) {
if (args.length != 2) {
System.out.println("Usage: ZipCat zipfile fileToPrint");
}
/*
* Creates AutoCloseable FileSystem and BufferedReader.
* They will be closed automatically after the try block.
* If reader initialization fails, then zipFileSystem will be closed
* automatically.
*/
try (FileSystem zipFileSystem
= FileSystems.newFileSystem(Paths.get(args[0]),null);
InputStream input
= Files.newInputStream(zipFileSystem.getPath(args[1]))) {
byte[] buffer = new byte[1024];
int len;
while ((len = input.read(buffer)) != -1) {
System.out.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
System.exit(1);
}
}
}