Java 24 in Action: Real-World Use Cases and Problem-Solving Examples
This is a companion article to our Java 24 Features Guide. Here, we'll explore practical implementations and real-world solutions using Java 24's new features.
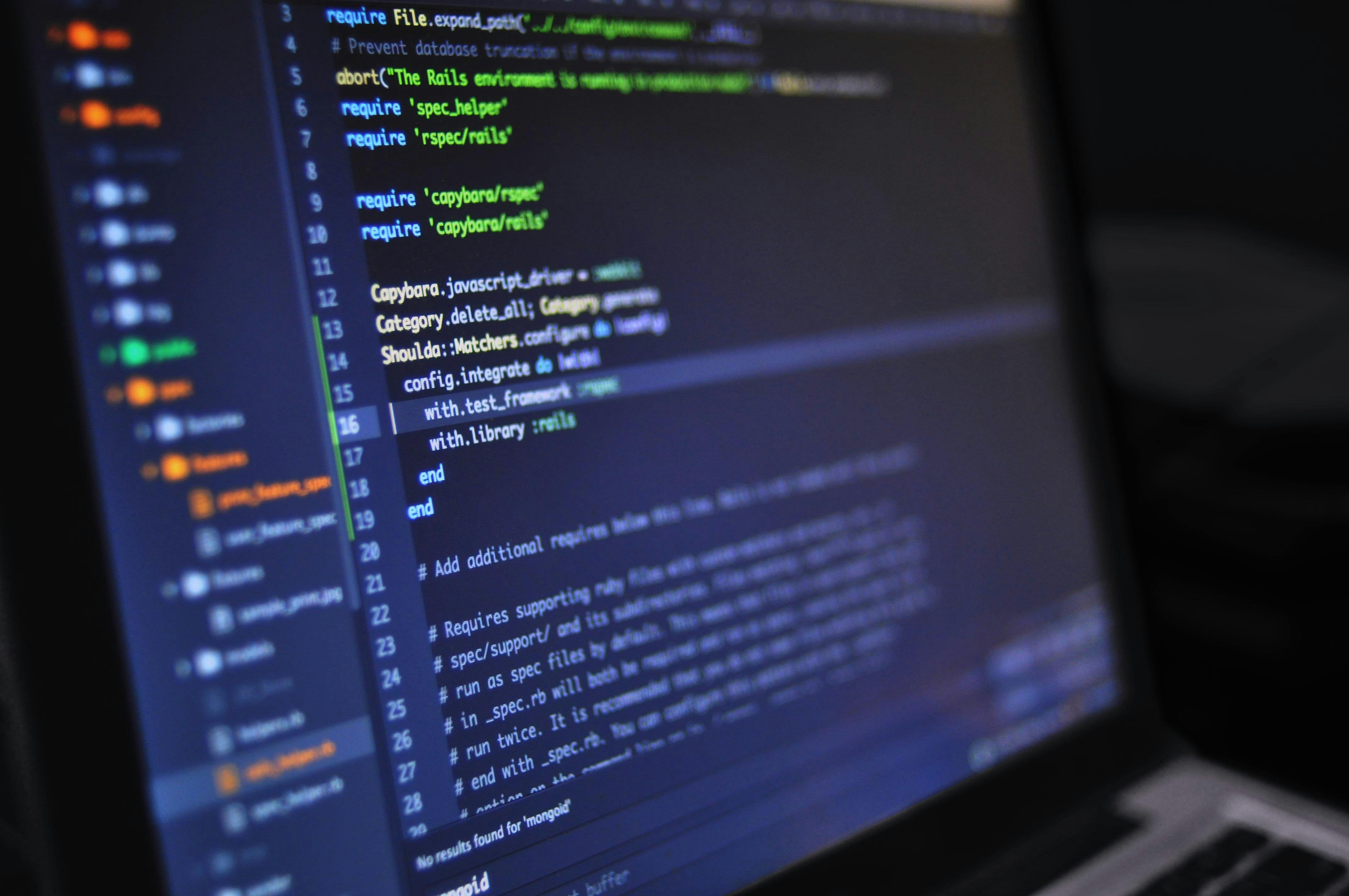
1. Efficient Memory Management with Foreign Function & Memory API
Using the Foreign Function & Memory API for high-performance data processing
Processing large datasets in Java has always been memory-intensive. The Foreign Function & Memory API in Java 24 enables direct memory access and efficient data manipulation.
Problem with Traditional Approach:
// Old approach - loads entire dataset into heap memory
public class DataProcessor {
public void processLargeDataset(String filePath) {
try {
byte[] data = Files.readAllBytes(Path.of(filePath));
// Process data in memory
for (int i = 0; i < data.length; i++) {
// Memory-intensive operation
data[i] = processByte(data[i]);
}
Files.write(Path.of("output.dat"), data);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Solution with Java 24 Foreign Function & Memory API:
public class EfficientDataProcessor {
// Modern record for processing metadata
record ProcessingMetadata(long size, int chunkSize) {}
// Modern sealed interface for processing operations
sealed interface ProcessingOperation {
record Transform(byte[] transformMatrix) implements ProcessingOperation {}
record Filter(byte threshold) implements ProcessingOperation {}
record Compress(int level) implements ProcessingOperation {}
}
public void processLargeDataset(String filePath, ProcessingOperation operation) {
try (var arena = Arena.ofConfined()) {
// Modern pattern matching for file operations
var result = switch (operation) {
case ProcessingOperation.Transform transform ->
processWithTransform(arena, filePath, transform.transformMatrix());
case ProcessingOperation.Filter filter ->
processWithFilter(arena, filePath, filter.threshold());
case ProcessingOperation.Compress compress ->
processWithCompression(arena, filePath, compress.level());
};
// Write processed data using modern try-with-resources
try (var channel = FileChannel.open(
Path.of("output.dat"),
StandardOpenOption.CREATE,
StandardOpenOption.WRITE)) {
channel.write(result.asByteBuffer());
}
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
private MemorySegment processWithTransform(Arena arena, String filePath, byte[] matrix) {
// Implementation using modern memory access patterns
var metadata = readFileMetadata(filePath);
var buffer = arena.allocate(metadata.size());
// Process data with transformation matrix
return buffer;
}
private ProcessingMetadata readFileMetadata(String filePath) {
// Implementation for reading file metadata
return new ProcessingMetadata(1024 * 1024 * 100, 8192); // 100MB file, 8KB chunks
}
public static void main(String[] args) {
var processor = new EfficientDataProcessor();
processor.processLargeDataset("input.dat",
new ProcessingOperation.Transform(new byte[]{1, 2, 3, 4}));
}
}
2. Thread-Safe Context Propagation with Scoped Values
Using Scoped Values for reliable context management
Managing context across async operations has been challenging. Java 24's Scoped Values provide a safer alternative to ThreadLocal for context propagation.
Problem with Traditional Approach:
// Old approach using ThreadLocal - prone to memory leaks
public class RequestContext {
private static final ThreadLocal requestId =
ThreadLocal.withInitial(() -> "");
private static final ThreadLocal currentUser =
ThreadLocal.withInitial(() -> null);
public static void setContext(String reqId, User user) {
requestId.set(reqId);
currentUser.set(user);
}
public static void clearContext() {
requestId.remove();
currentUser.remove();
}
public static void processRequest() {
try {
// Set context
setContext("REQ-123", new User("john"));
// Async operation - context lost!
CompletableFuture.runAsync(() -> {
// ThreadLocal values don't propagate
System.out.println("Request ID: " + requestId.get());
});
} finally {
clearContext(); // Must remember to clear
}
}
}
Solution with Java 24 Scoped Values:
public class ModernRequestContext {
// Modern sealed interface for context values
sealed interface ContextValue {
record RequestId(String id) implements ContextValue {}
record User(String name, String role) implements ContextValue {}
record Tenant(String id, String name) implements ContextValue {}
}
// Modern scoped values with type safety
private static final ScopedValue REQUEST_ID = ScopedValue.newInstance();
private static final ScopedValue CURRENT_USER = ScopedValue.newInstance();
private static final ScopedValue CURRENT_TENANT = ScopedValue.newInstance();
// Modern record for request context
record RequestContext(
RequestId requestId,
User user,
Tenant tenant
) {}
public void processRequest(RequestContext context) {
// Modern pattern matching with scoped values
ScopedValue.where(REQUEST_ID, context.requestId())
.where(CURRENT_USER, context.user())
.where(CURRENT_TENANT, context.tenant())
.run(() -> {
// Process request with modern switch expressions
var result = switch (context.user().role()) {
case "admin" -> processAdminRequest();
case "user" -> processUserRequest();
default -> throw new IllegalStateException("Unknown role");
};
// Modern async processing with structured concurrency
try (var scope = new StructuredTaskScope.ShutdownOnFailure()) {
var future = scope.fork(() -> processAsync(result));
scope.join();
future.get();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
private String processAdminRequest() {
return "Admin request processed";
}
private String processUserRequest() {
return "User request processed";
}
private CompletableFuture processAsync(String result) {
return CompletableFuture.completedFuture(result);
}
public static void main(String[] args) {
var context = new RequestContext(
new ContextValue.RequestId("REQ-123"),
new ContextValue.User("john", "admin"),
new ContextValue.Tenant("T1", "Acme Corp")
);
new ModernRequestContext().processRequest(context);
}
}
3. Enhanced Pattern Matching with Record Patterns
Using Record Patterns for more expressive data handling
Java 24 introduces Record Patterns, making it easier to work with record types and nested data structures.
Problem with Traditional Approach:
// Old approach - verbose pattern matching
public class DataProcessor {
public void processData(Object data) {
if (data instanceof Point point) {
int x = point.x();
int y = point.y();
processPoint(x, y);
} else if (data instanceof Rectangle rect) {
Point topLeft = rect.topLeft();
Point bottomRight = rect.bottomRight();
processRectangle(topLeft.x(), topLeft.y(),
bottomRight.x(), bottomRight.y());
}
}
}
Solution with Java 24 Record Patterns:
public class ModernDataProcessor {
// Modern record types
record Point(int x, int y) {}
record Rectangle(Point topLeft, Point bottomRight) {}
record Circle(Point center, int radius) {}
// Modern sealed interface for shapes
sealed interface Shape {
record Square(Point topLeft, int size) implements Shape {}
record Triangle(Point p1, Point p2, Point p3) implements Shape {}
}
public void processData(Object data) {
// Modern record patterns with nested matching
switch (data) {
case Point(int x, int y) ->
processPoint(x, y);
case Rectangle(Point(int x1, int y1), Point(int x2, int y2)) ->
processRectangle(x1, y1, x2, y2);
case Circle(Point(int cx, int cy), int r) ->
processCircle(cx, cy, r);
case Shape.Square(Point(int x, int y), int size) ->
processSquare(x, y, size);
case Shape.Triangle(Point(int x1, int y1),
Point(int x2, int y2),
Point(int x3, int y3)) ->
processTriangle(x1, y1, x2, y2, x3, y3);
default ->
throw new IllegalArgumentException("Unknown shape");
}
}
private void processPoint(int x, int y) {
System.out.printf("Processing point at (%d, %d)%n", x, y);
}
private void processRectangle(int x1, int y1, int x2, int y2) {
System.out.printf("Processing rectangle from (%d, %d) to (%d, %d)%n",
x1, y1, x2, y2);
}
private void processCircle(int cx, int cy, int r) {
System.out.printf("Processing circle at (%d, %d) with radius %d%n",
cx, cy, r);
}
private void processSquare(int x, int y, int size) {
System.out.printf("Processing square at (%d, %d) with size %d%n",
x, y, size);
}
private void processTriangle(int x1, int y1, int x2, int y2, int x3, int y3) {
System.out.printf("Processing triangle with points (%d, %d), (%d, %d), (%d, %d)%n",
x1, y1, x2, y2, x3, y3);
}
public static void main(String[] args) {
var processor = new ModernDataProcessor();
// Test with various shapes
processor.processData(new Point(10, 20));
processor.processData(new Rectangle(new Point(0, 0), new Point(100, 100)));
processor.processData(new Circle(new Point(50, 50), 25));
processor.processData(new Shape.Square(new Point(0, 0), 50));
processor.processData(new Shape.Triangle(
new Point(0, 0),
new Point(50, 0),
new Point(25, 50)
));
}
}
Conclusion and Best Practices
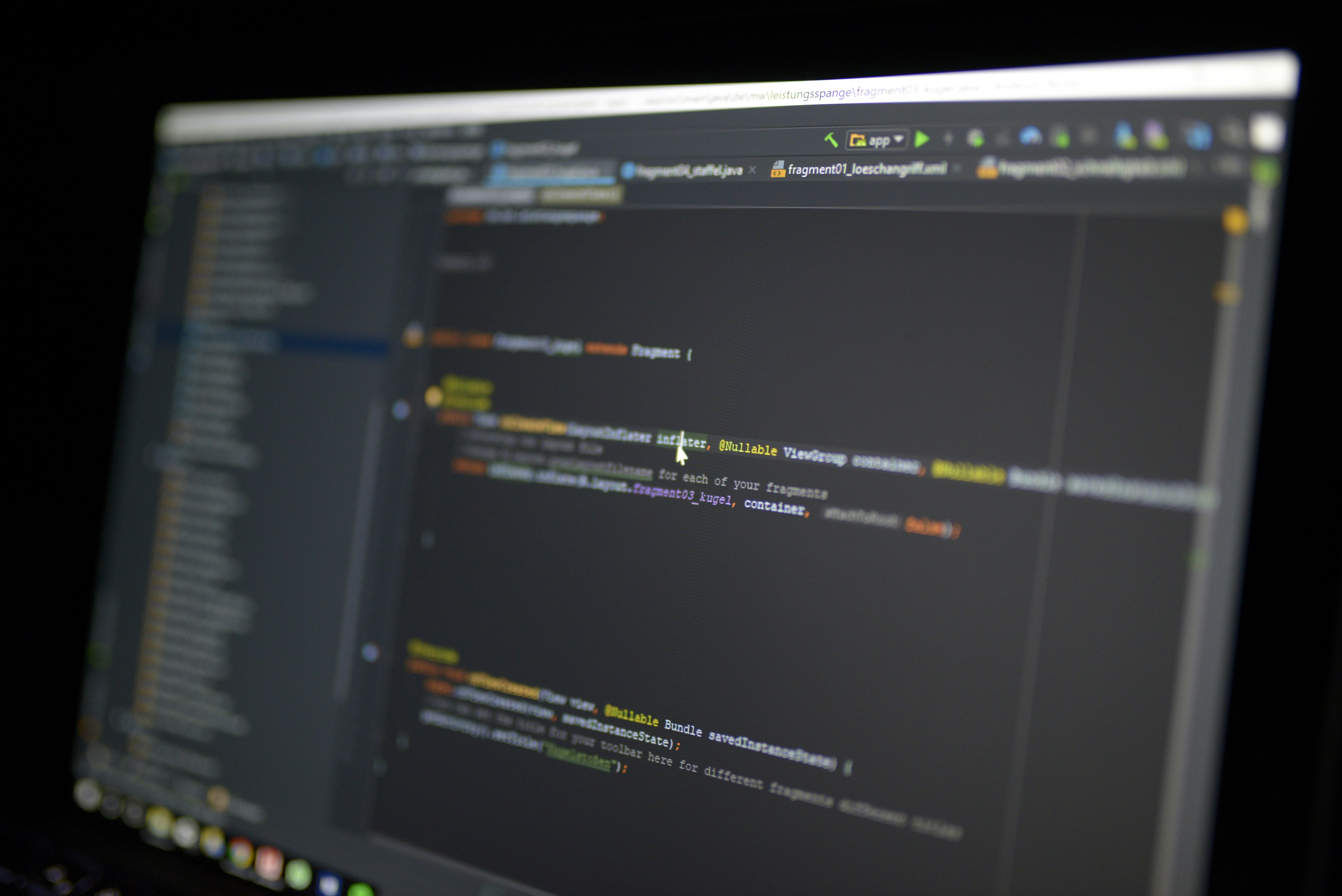
These examples demonstrate how Java 24's features solve real-world problems:
- Foreign Function & Memory API enables efficient memory management for large data processing
- Scoped Values offer a safer alternative to ThreadLocal for context propagation
- Record Patterns provide more expressive pattern matching for complex data structures
Remember these best practices:
- Always use try-with-resources with Arena instances when using Foreign Memory API
- Design your Scoped Values to be immutable
- Use Record Patterns to simplify complex data structure handling
- Consider performance implications when choosing between heap and off-heap memory
GitHub Repository: Find the complete source code for these examples in our
Java 24 Examples repository.