SOLID Principles in Java: A Comprehensive Guide (2025)
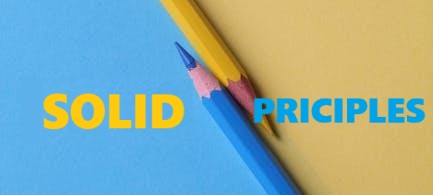
SOLID principles are fundamental guidelines in object-oriented programming that help developers create more maintainable, flexible, and scalable software. This comprehensive guide explores each principle with practical Java examples and real-world applications.
Table of Contents
Introduction to SOLID Principles
SOLID is an acronym for five design principles in object-oriented programming that help developers create more maintainable and flexible software. These principles are:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
These principles were first introduced by Robert C. Martin (Uncle Bob) in his paper "Design Principles and Design Patterns" and later popularized in his book "Clean Code". They serve as guidelines for writing clean, maintainable, and scalable code that can adapt to changing requirements without major refactoring.
Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This means that a class should have only one job or responsibility. When a class has multiple responsibilities, it becomes harder to maintain, test, and modify. Following SRP helps in:
- Improving code readability
- Making the code easier to test
- Reducing the risk of bugs
- Making the code more maintainable
Example of SRP
// Bad Example - Multiple Responsibilities
class Employee {
public void calculateSalary() { /* ... */ }
public void saveToDatabase() { /* ... */ }
public void generateReport() { /* ... */ }
}
// Good Example - Single Responsibility
class Employee {
private String name;
private double salary;
// Getters and setters
}
class SalaryCalculator {
public double calculateSalary(Employee employee) {
// Salary calculation logic
return employee.getSalary();
}
}
class EmployeeRepository {
public void save(Employee employee) {
// Database operations
}
}
class ReportGenerator {
public void generateReport(Employee employee) {
// Report generation logic
}
}
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that you should be able to add new functionality without changing existing code. The benefits of following OCP include:
- Reduced risk of introducing bugs in existing code
- Better code organization
- Easier to add new features
- Improved maintainability
Example of OCP
// Bad Example - Not Open for Extension
class Rectangle {
public double width;
public double height;
}
class AreaCalculator {
public double calculateArea(Rectangle rectangle) {
return rectangle.width * rectangle.height;
}
}
// Good Example - Open for Extension
interface Shape {
double calculateArea();
}
class Rectangle implements Shape {
private double width;
private double height;
@Override
public double calculateArea() {
return width * height;
}
}
class Circle implements Shape {
private double radius;
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle, named after Barbara Liskov, states that objects of a superclass should be replaceable with objects of its subclasses without breaking the application. This principle ensures that:
- Subtypes must be substitutable for their base types
- Subclasses should not strengthen preconditions
- Subclasses should not weaken postconditions
- Subclasses should maintain the invariant of the superclass
Following LSP helps in:
- Maintaining type safety
- Ensuring proper inheritance hierarchy
- Making code more reliable
- Facilitating proper polymorphism
Example of LSP
// Bad Example - Violating LSP
class Bird {
public void fly() {
// Flying implementation
}
}
class Penguin extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Penguins can't fly!");
}
}
// Good Example - Following LSP
interface FlyingBird {
void fly();
}
interface SwimmingBird {
void swim();
}
class Sparrow implements FlyingBird {
@Override
public void fly() {
// Flying implementation
}
}
class Penguin implements SwimmingBird {
@Override
public void swim() {
// Swimming implementation
}
}
Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they don't use. This means that:
- Interfaces should be specific to the client's needs
- Large interfaces should be broken down into smaller ones
- Clients should only know about the methods they use
- Changes to one interface should not affect clients using another interface
Benefits of following ISP include:
- Better code organization
- Reduced coupling between components
- Easier to maintain and modify
- Improved code reusability
Example of ISP
// Bad Example - Too Large Interface
interface Worker {
void work();
void eat();
void sleep();
}
// Good Example - Segregated Interfaces
interface Workable {
void work();
}
interface Eatable {
void eat();
}
interface Sleepable {
void sleep();
}
class Human implements Workable, Eatable, Sleepable {
@Override
public void work() { /* ... */ }
@Override
public void eat() { /* ... */ }
@Override
public void sleep() { /* ... */ }
}
class Robot implements Workable {
@Override
public void work() { /* ... */ }
}
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. This principle helps in:
- Reducing coupling between modules
- Making the code more flexible and maintainable
- Facilitating easier testing
- Enabling better code reuse
Key aspects of DIP include:
- Depend on abstractions, not concrete implementations
- Use dependency injection
- Program to an interface, not an implementation
- Invert the control of dependencies
Example of DIP
// Bad Example - Direct Dependency
class EmailNotifier {
public void sendEmail(String message) {
// Email sending implementation
}
}
class OrderProcessor {
private EmailNotifier emailNotifier = new EmailNotifier();
public void processOrder(Order order) {
// Process order
emailNotifier.sendEmail("Order processed");
}
}
// Good Example - Dependency Inversion
interface NotificationService {
void sendNotification(String message);
}
class EmailNotifier implements NotificationService {
@Override
public void sendNotification(String message) {
// Email sending implementation
}
}
class SMSNotifier implements NotificationService {
@Override
public void sendNotification(String message) {
// SMS sending implementation
}
}
class OrderProcessor {
private final NotificationService notificationService;
public OrderProcessor(NotificationService notificationService) {
this.notificationService = notificationService;
}
public void processOrder(Order order) {
// Process order
notificationService.sendNotification("Order processed");
}
}
Best Practices and Implementation
Implementing SOLID principles effectively requires a balanced approach. Here are some key considerations:
Implementation Guidelines
- Start with a clear understanding of the problem domain
- Identify potential areas of change
- Design interfaces carefully
- Use dependency injection
- Apply principles gradually
- Consider the trade-offs
- Test your design decisions
Common Pitfalls to Avoid
- Over-engineering solutions
- Creating too many small classes
- Ignoring the YAGNI principle
- Making the code more complex than necessary
- Not considering the actual requirements
Conclusion
SOLID principles provide a solid foundation for writing clean, maintainable, and scalable code. By following these principles, developers can create more flexible and robust software systems. Remember that while these principles are important, they should be applied thoughtfully and in the context of your specific project needs.