Web Security Basics: Essential Guide
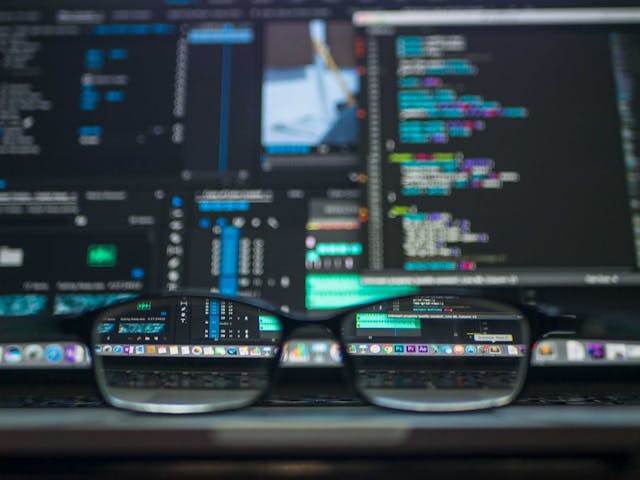
Web Security Fundamentals Overview
Introduction to Web Security
Web security is crucial in today's digital landscape where web applications handle sensitive data and critical business operations. This guide covers fundamental security concepts, common vulnerabilities, and essential protection measures.
Core Security Principles
- Confidentiality: Ensuring data is accessible only to authorized users
- Integrity: Maintaining data accuracy and trustworthiness
- Availability: Keeping systems and data accessible when needed
Common Web Vulnerabilities
1. Injection Attacks
Occurs when untrusted data is sent to an interpreter as part of a command or query.
// Vulnerable SQL Query String query = "SELECT * FROM users WHERE username = '" + username + "'"; // Secure Parameterized Query PreparedStatement stmt = conn.prepareStatement("SELECT * FROM users WHERE username = ?"); stmt.setString(1, username);
2. Cross-Site Scripting (XSS)
Occurs when an application includes untrusted data in a web page without proper validation.
// Vulnerable element.innerHTML = userInput; // Secure element.textContent = userInput;
3. Broken Authentication
Weaknesses in authentication and session management that allow attackers to compromise passwords or session tokens.
Essential Security Measures
1. Input Validation
- Validate input on both client and server side
- Use whitelisting approach
- Sanitize output data
2. Authentication & Authorization
- Implement strong password policies
- Use multi-factor authentication
- Implement proper session management
3. HTTPS Implementation
- Use HTTPS for all pages
- Implement HSTS
- Keep SSL/TLS certificates updated
Security Headers
Content-Security-Policy: default-src 'self' X-Frame-Options: DENY X-XSS-Protection: 1; mode=block X-Content-Type-Options: nosniff Referrer-Policy: strict-origin-when-cross-origin Permissions-Policy: geolocation=()
Security Best Practices
- Keep software and dependencies updated
- Implement proper error handling
- Use secure configuration in production
- Regular security testing and audits
- Implement logging and monitoring