OWASP Top 10 Web Application Security Risks
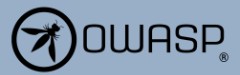
OWASP Top 10 Security Risks Overview
The OWASP Top 10 represents the most critical security risks to web applications. This guide provides detailed information about each risk, including examples and prevention methods.
A01:2021 - Broken Access Control High Risk
Access control enforces policy such that users cannot act outside of their intended permissions.
Common Vulnerabilities:
- Bypassing access control checks
- Elevation of privilege
- Metadata manipulation
// Vulnerable Code if (user.isLoggedIn()) { return sensitiveData; } // Secure Code if (user.isLoggedIn() && user.hasPermission('VIEW_SENSITIVE_DATA')) { return sensitiveData; }
Prevention Methods:
- Implement proper access controls
- Deny by default
- Log access control failures
- Rate limit API and controller access
A02:2021 - Cryptographic Failures High Risk
Failures related to cryptography that often lead to exposure of sensitive data.
Common Issues:
- Transmission of sensitive data in clear text
- Weak cryptographic algorithms
- Incorrect certificate validation
Prevention Methods:
- Encrypt all sensitive data at rest
- Use strong, up-to-date algorithms
- Proper key management
- Enforce HTTPS for all connections
A03:2021 - Injection High Risk
Injection flaws occur when untrusted data is sent to an interpreter as part of a command or query.
// SQL Injection Example - Vulnerable String query = "SELECT * FROM users WHERE username = '" + username + "'"; // Safe Implementation PreparedStatement stmt = connection.prepareStatement( "SELECT * FROM users WHERE username = ?" ); stmt.setString(1, username);
Prevention Methods:
- Use parameterized queries
- Input validation
- Escape special characters
- Use ORMs with proper configuration
A10:2021 - Server-Side Request Forgery Medium Risk
SSRF flaws occur when a web application is fetching a remote resource without validating the user-supplied URL.
// Vulnerable Code app.get('/fetch', (req, res) => { fetch(req.query.url) .then(response => response.json()) .then(data => res.json(data)); }); // Secure Code app.get('/fetch', (req, res) => { const url = new URL(req.query.url); if (isAllowedHost(url.hostname)) { fetch(url) .then(response => response.json()) .then(data => res.json(data)); } });
Prevention Methods:
- Validate and sanitize all client-supplied input data
- Enforce URL schema, port, and destination with a positive allow list
- Do not send raw responses to clients
- Disable HTTP redirections